Python中实现卷积的方式主要有:使用NumPy手动实现、利用SciPy库的convolve函数、以及使用TensorFlow或PyTorch等深度学习框架。其中,NumPy提供了灵活性,适合初学者了解卷积的基本原理;SciPy则提供了更高效的实现,适用于科学计算;TensorFlow和PyTorch能够处理复杂的多维卷积运算,常用于深度学习模型的构建。下面将详细介绍这几种方法。
一、NUMPY手动实现卷积
使用NumPy手动实现卷积运算是理解卷积基本原理的一个好方法。卷积运算的核心思想是通过一个内核(或称滤波器)在输入数据上滑动,并计算内核与数据的点积之和。
-
理解卷积的基本原理
卷积是一种信号处理技术,广泛用于图像处理和神经网络中。基本步骤是:首先翻转卷积核,然后将其沿输入数组滑动,并计算重叠区域的点积。这个过程在图像处理和神经网络中用于特征提取和模式识别。
-
使用NumPy实现一维卷积
在NumPy中,我们可以通过循环和数组操作手动实现一维卷积。这种方法灵活且易于理解,但在处理大规模数据时效率可能较低。
import numpy as np
def conv1d(input_array, kernel):
input_length = len(input_array)
kernel_length = len(kernel)
output_length = input_length - kernel_length + 1
output_array = np.zeros(output_length)
for i in range(output_length):
output_array[i] = np.sum(input_array[i:i+kernel_length] * kernel[::-1])
return output_array
示例
input_array = np.array([1, 2, 3, 4, 5])
kernel = np.array([1, 0, -1])
result = conv1d(input_array, kernel)
print("一维卷积结果:", result)
-
使用NumPy实现二维卷积
二维卷积在图像处理中特别有用。我们可以扩展一维卷积的思路,通过嵌套循环实现二维卷积。
def conv2d(input_matrix, kernel):
input_height, input_width = input_matrix.shape
kernel_height, kernel_width = kernel.shape
output_height = input_height - kernel_height + 1
output_width = input_width - kernel_width + 1
output_matrix = np.zeros((output_height, output_width))
for i in range(output_height):
for j in range(output_width):
output_matrix[i, j] = np.sum(input_matrix[i:i+kernel_height, j:j+kernel_width] * kernel[::-1, ::-1])
return output_matrix
示例
input_matrix = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
kernel = np.array([[1, 0], [0, -1]])
result = conv2d(input_matrix, kernel)
print("二维卷积结果:\n", result)
二、使用SCIPY库的CONVOLVE函数
SciPy是一个强大的科学计算库,提供了高效的卷积操作函数,如convolve
和convolve2d
,使得卷积操作更为简便和快速。
-
一维卷积的实现
SciPy中的
convolve
函数可以直接用于一维卷积。相比手动实现,这个函数的速度更快,并且使用起来更加方便。from scipy.signal import convolve
input_array = np.array([1, 2, 3, 4, 5])
kernel = np.array([1, 0, -1])
result = convolve(input_array, kernel, mode='valid')
print("SciPy一维卷积结果:", result)
-
二维卷积的实现
对于二维卷积,SciPy提供了
convolve2d
函数。这个函数专为2D数组设计,适用于图像处理等应用。from scipy.signal import convolve2d
input_matrix = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
kernel = np.array([[1, 0], [0, -1]])
result = convolve2d(input_matrix, kernel, mode='valid')
print("SciPy二维卷积结果:\n", result)
三、利用TENSORFLOW进行卷积操作
TensorFlow是一个广泛使用的深度学习框架,提供了强大的卷积操作功能,支持多维度的数据处理,适合处理复杂的神经网络任务。
-
TensorFlow中的卷积层
TensorFlow中的卷积操作主要通过
tf.nn.conv1d
、tf.nn.conv2d
等函数实现。使用TensorFlow进行卷积操作时,通常需要先定义输入数据和卷积核的形状,并设置步长、填充等参数。import tensorflow as tf
一维卷积
input_array = tf.constant([[1, 2, 3, 4, 5]], dtype=tf.float32)
kernel = tf.constant([[1, 0, -1]], dtype=tf.float32)
result = tf.nn.conv1d(input_array, kernel, stride=1, padding='VALID')
print("TensorFlow一维卷积结果:", result.numpy())
二维卷积
input_matrix = tf.constant([[[1], [2], [3]], [[4], [5], [6]], [[7], [8], [9]]], dtype=tf.float32)
kernel = tf.constant([[[1]], [[0]], [[-1]]], dtype=tf.float32)
result = tf.nn.conv2d(input_matrix, kernel, strides=[1, 1, 1, 1], padding='VALID')
print("TensorFlow二维卷积结果:\n", result.numpy())
-
使用卷积层构建神经网络
在TensorFlow中,卷积层通常与其他层(如池化层、全连接层)结合使用,构建复杂的神经网络结构。卷积神经网络(CNN)在图像分类、目标检测等领域取得了显著的成果。
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Conv2D, Flatten, Dense
model = Sequential([
Conv2D(32, (3, 3), activation='relu', input_shape=(28, 28, 1)),
Flatten(),
Dense(10, activation='softmax')
])
model.summary()
四、利用PYTORCH进行卷积操作
PyTorch是另一个广泛使用的深度学习框架,与TensorFlow相似,提供了强大的卷积操作功能,支持灵活的模型构建和训练。
-
PyTorch中的卷积层
在PyTorch中,卷积层通过
torch.nn.Conv1d
、torch.nn.Conv2d
等模块实现。与TensorFlow类似,使用PyTorch进行卷积操作时,需要先定义输入数据和卷积核的形状,并设置步长、填充等参数。import torch
import torch.nn as nn
一维卷积
input_array = torch.tensor([[1, 2, 3, 4, 5]], dtype=torch.float32)
kernel = torch.tensor([[[1, 0, -1]]], dtype=torch.float32)
conv1d = nn.Conv1d(in_channels=1, out_channels=1, kernel_size=3, stride=1)
conv1d.weight.data = kernel
result = conv1d(input_array.unsqueeze(0))
print("PyTorch一维卷积结果:", result)
二维卷积
input_matrix = torch.tensor([[[[1, 2, 3], [4, 5, 6], [7, 8, 9]]]], dtype=torch.float32)
kernel = torch.tensor([[[[1, 0], [0, -1]]]], dtype=torch.float32)
conv2d = nn.Conv2d(in_channels=1, out_channels=1, kernel_size=(2, 2), stride=1)
conv2d.weight.data = kernel
result = conv2d(input_matrix)
print("PyTorch二维卷积结果:\n", result)
-
使用卷积层构建神经网络
PyTorch中的卷积层通常与其他层(如池化层、全连接层)结合使用,构建复杂的神经网络结构。其灵活的动态计算图机制,使得模型的构建和调试更加直观和便利。
import torch.nn.functional as F
class SimpleCNN(nn.Module):
def __init__(self):
super(SimpleCNN, self).__init__()
self.conv1 = nn.Conv2d(1, 32, kernel_size=3)
self.fc1 = nn.Linear(5408, 10) # 调整输入特征数量
def forward(self, x):
x = F.relu(self.conv1(x))
x = x.view(x.size(0), -1)
x = self.fc1(x)
return F.log_softmax(x, dim=1)
model = SimpleCNN()
print(model)
通过以上不同方法的介绍,可以看到Python中进行卷积操作的多种实现方式,每种方法各有其适用场景和优势。对于初学者来说,理解卷积的基本原理是关键,而对于需要进行大规模数据处理或深度学习模型构建的用户,SciPy、TensorFlow和PyTorch提供了更高效和强大的工具。根据具体需求选择合适的方法,可以更好地实现卷积操作。
相关问答FAQs:
如何在Python中实现卷积操作?
在Python中,可以使用NumPy库轻松实现卷积操作。NumPy提供了np.convolve()
函数,适用于一维卷积。对于二维卷积,可以使用scipy.ndimage.convolve()
或tensorflow
、pytorch
等深度学习框架中的卷积函数。这些库支持对图像等多维数组进行卷积,提供了灵活的参数设置以适应不同的需求。
卷积操作在图像处理中有什么应用?
卷积在图像处理中被广泛应用,尤其是在边缘检测、模糊处理和特征提取等方面。通过不同的卷积核(滤波器),可以实现各种效果,例如使用拉普拉斯算子检测边缘,或者使用高斯滤波器进行图像平滑。掌握卷积的应用有助于提升图像处理的效果。
如何选择卷积核以获得最佳效果?
选择卷积核通常依赖于具体的应用场景。常见的卷积核包括锐化、模糊、边缘检测等。对于特定任务,可以根据需求自定义卷积核。了解不同卷积核的特性与用途,有助于在实际操作中获得理想效果,提升图像处理的质量和效率。
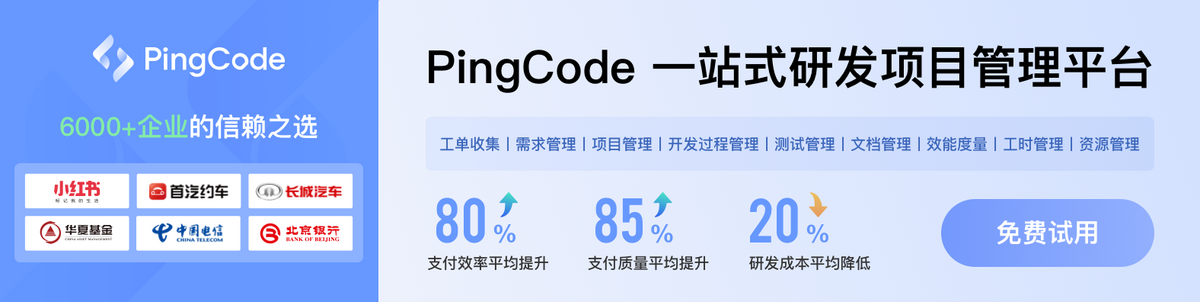