Python实现判断主要通过条件语句、逻辑运算符、内置函数等方式。条件语句包括if、elif、else,逻辑运算符包括and、or、not。内置函数如isinstance()、in等可以帮助进行类型判断和成员判断。在Python中,if语句是进行条件判断的核心工具。通过if语句,可以对不同的条件进行判断,并根据判断结果执行不同的代码块。下面我将详细解释如何在Python中实现判断。
一、条件语句
Python的条件语句是实现判断的主要工具。条件语句主要包括if
、elif
和else
,用于根据不同的条件执行不同的代码块。
1. if语句
if
语句用于判断一个条件是否为真,如果为真,则执行相应的代码块。
age = 18
if age >= 18:
print("You are an adult.")
在这个例子中,我们使用if
语句判断变量age
是否大于或等于18。如果条件为真,程序将输出"You are an adult."。
2. elif语句
elif
语句用于在if
语句之后添加多个条件。当第一个条件不满足时,程序将检查elif
条件。
age = 16
if age >= 18:
print("You are an adult.")
elif age >= 13:
print("You are a teenager.")
在这个例子中,如果age
不大于或等于18,则程序将检查elif
条件。如果age
大于或等于13,程序将输出"You are a teenager."。
3. else语句
else
语句用于在if
和elif
条件都不满足时执行的代码块。
age = 10
if age >= 18:
print("You are an adult.")
elif age >= 13:
print("You are a teenager.")
else:
print("You are a child.")
在这个例子中,age
既不大于或等于18,也不大于或等于13,因此程序将输出"You are a child."。
二、逻辑运算符
Python提供了逻辑运算符and
、or
和not
,用于连接和反转条件判断。
1. and运算符
and
运算符用于连接多个条件,只有当所有条件都为真时,整个表达式才为真。
age = 20
is_student = True
if age >= 18 and is_student:
print("You are an adult student.")
在这个例子中,只有当age
大于或等于18并且is_student
为真时,程序才会输出"You are an adult student."。
2. or运算符
or
运算符用于连接多个条件,只要有一个条件为真,整个表达式就为真。
age = 20
is_student = False
if age >= 18 or is_student:
print("You are either an adult or a student.")
在这个例子中,只要age
大于或等于18,或者is_student
为真,程序就会输出"You are either an adult or a student."。
3. not运算符
not
运算符用于反转条件判断。
age = 20
is_student = False
if not is_student:
print("You are not a student.")
在这个例子中,如果is_student
为假,程序将输出"You are not a student."。
三、内置函数
Python提供了许多内置函数,用于帮助进行判断。
1. isinstance()函数
isinstance()
函数用于判断一个对象是否是某个类型的实例。
x = 5
if isinstance(x, int):
print("x is an integer.")
在这个例子中,isinstance()
函数用于判断变量x
是否是整数类型。
2. in运算符
in
运算符用于判断一个值是否在序列中。
fruits = ["apple", "banana", "cherry"]
if "banana" in fruits:
print("Banana is in the list.")
在这个例子中,in
运算符用于判断字符串"banana"是否在列表fruits
中。
四、结合条件语句和函数实现复杂判断
通过将条件语句、逻辑运算符和内置函数结合使用,可以实现复杂的判断逻辑。
1. 复杂条件判断
age = 20
is_student = True
is_employed = False
if age >= 18 and (is_student or is_employed):
print("You are eligible for the program.")
else:
print("You are not eligible for the program.")
在这个例子中,我们使用了and
和or
运算符,以及括号来定义复杂的条件判断。只有当age
大于或等于18,并且是学生或者有工作时,程序才会输出"You are eligible for the program."。
2. 使用函数进行判断
可以将判断逻辑封装在函数中,以提高代码的可读性和可维护性。
def is_eligible_for_discount(age, is_student):
return age < 18 or is_student
age = 17
is_student = False
if is_eligible_for_discount(age, is_student):
print("You are eligible for a discount.")
else:
print("You are not eligible for a discount.")
在这个例子中,我们定义了一个函数is_eligible_for_discount()
,用于判断一个人是否有资格享受折扣。通过将判断逻辑封装在函数中,可以更清晰地表达判断条件,并在需要时轻松复用。
五、使用Python实现判断的最佳实践
-
简化条件表达式:尽量简化条件表达式,以提高代码的可读性。可以通过使用变量名来替代复杂的表达式。
-
避免重复判断:如果多个条件判断中有重复的部分,可以考虑将其提取为单独的变量或函数,以避免重复。
-
使用函数封装判断逻辑:将复杂的判断逻辑封装在函数中,以提高代码的可读性和可维护性。
-
考虑边界条件:在进行条件判断时,确保考虑到所有可能的边界条件,以避免遗漏。
-
使用注释:在复杂的条件判断中,使用注释来解释判断逻辑,以帮助其他开发人员理解代码。
通过以上方法和技巧,可以在Python中有效地实现各种判断逻辑,并提高代码的质量和可维护性。
相关问答FAQs:
如何在Python中进行条件判断?
在Python中,条件判断主要通过if
语句实现。你可以使用if
、elif
和else
来控制程序的执行流程。例如,下面的代码演示了如何判断一个数字是正数、负数还是零:
number = 10
if number > 0:
print("这是一个正数")
elif number < 0:
print("这是一个负数")
else:
print("这是零")
通过这种结构,可以实现多种条件判断。
Python中的逻辑运算符如何在判断中使用?
逻辑运算符如and
、or
和not
可以帮助你在条件判断中组合多个条件。例如,你可以同时判断一个数是否在特定范围内:
age = 20
if age >= 18 and age <= 65:
print("年龄在18到65岁之间")
使用逻辑运算符可以让条件判断更加灵活和强大。
如何使用Python的三元表达式简化判断?
Python支持三元表达式,可以用来简化简单的条件判断。这种写法使得代码更简洁。例如,以下代码展示了如何用三元表达式判断一个数的奇偶性:
number = 4
result = "偶数" if number % 2 == 0 else "奇数"
print(result)
这种方式在需要根据条件快速返回结果时非常实用。
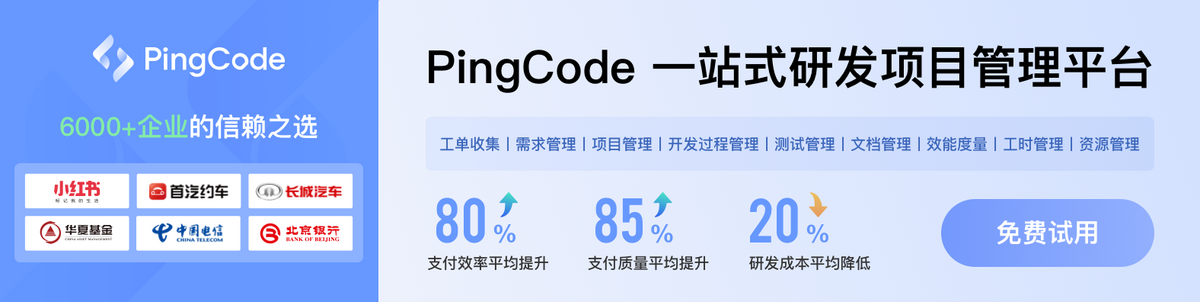