一、PYTHON读取HTML的三种方式:使用内置库、使用外部库、使用网络请求库
Python读取HTML的方式包括使用内置库、使用外部库、使用网络请求库。 在实际使用中,选择哪种方式取决于具体需求。例如,如果只需要简单地读取和解析HTML,可以选择Python的内置库html.parser
。如果需要更强大的功能和更灵活的解析能力,则可以选择外部库如BeautifulSoup或lxml。对于需要从网络上抓取HTML内容的情况,可以使用requests库进行HTTP请求,然后结合BeautifulSoup进行解析。
-
使用内置库:html.parser
Python的标准库中包含一个简单的HTML解析器
html.parser
。虽然功能相对较为基础,但对于处理简单的HTML文档已经足够。使用html.parser
可以轻松解析HTML文档,并进行简单的DOM操作。详细描述:
html.parser
是Python内置的HTML解析库,其使用方法简单直接。首先,可以通过Python的open()
函数读取HTML文件。然后,将读取的内容传递给html.parser
进行解析。解析后的数据可以通过find()
、find_all()
等方法进行查找和操作。例如,如果需要提取HTML中的特定标签内容,可以通过find_all('tag_name')
获取所有该标签的内容列表。html.parser
的优点是易于使用且不需要额外安装第三方库,但在处理复杂HTML文档时,可能不如其他外部库高效。
二、使用外部库:BeautifulSoup、lxml
-
BeautifulSoup
BeautifulSoup是一个强大的HTML/XML解析库,提供了丰富的API来处理和提取HTML数据。它支持多种解析器,可以通过
html.parser
、lxml
等进行解析。 -
lxml
lxml是一个高效的XML和HTML解析库,具有强大的解析和操作能力。与BeautifulSoup相比,lxml在处理大型HTML文档时表现更好,但其API使用相对复杂。
三、使用网络请求库:requests
-
使用requests获取HTML内容
对于从网络上获取HTML内容的需求,requests库是一个非常方便的选择。它可以轻松发送HTTP请求,获取网页内容,并结合BeautifulSoup等库进行解析。
-
结合BeautifulSoup解析请求内容
将requests获取的HTML内容传递给BeautifulSoup进行解析,可以轻松实现对网页内容的提取和操作。
以下是详细的介绍和示例代码:
一、使用内置库:html.parser
-
解析HTML文件
使用Python的
open()
函数读取HTML文件,并使用html.parser
进行解析。from html.parser import HTMLParser
class MyHTMLParser(HTMLParser):
def handle_starttag(self, tag, attrs):
print(f"Start tag: {tag}")
def handle_endtag(self, tag):
print(f"End tag: {tag}")
def handle_data(self, data):
print(f"Data: {data}")
读取本地HTML文件
with open('example.html', 'r', encoding='utf-8') as file:
content = file.read()
parser = MyHTMLParser()
parser.feed(content)
-
解析HTML字符串
如果HTML内容是以字符串形式存在,也可以直接使用
html.parser
进行解析。html_content = """
<html>
<head><title>Test</title></head>
<body>
<h1>Parse me!</h1>
</body>
</html>
"""
parser = MyHTMLParser()
parser.feed(html_content)
二、使用外部库:BeautifulSoup、lxml
-
使用BeautifulSoup解析HTML
BeautifulSoup提供了更强大的解析功能,使用简单且支持多种解析器。
from bs4 import BeautifulSoup
html_doc = """
<html>
<head><title>The Dormouse's story</title></head>
<body>
<p class="title"><b>The Dormouse's story</b></p>
<p class="story">Once upon a time there were three little sisters; and their names were
<a href="http://example.com/elsie" class="sister" id="link1">Elsie</a>,
<a href="http://example.com/lacie" class="sister" id="link2">Lacie</a> and
<a href="http://example.com/tillie" class="sister" id="link3">Tillie</a>;
and they lived at the bottom of a well.</p>
<p class="story">...</p>
</body>
</html>
"""
soup = BeautifulSoup(html_doc, 'html.parser')
print(soup.prettify()) # 美化输出HTML
print(soup.title) # 获取title标签内容
print(soup.find_all('a')) # 获取所有a标签
-
使用lxml解析HTML
lxml是另一个强大的解析库,适用于需要高效处理HTML/XML的场景。
from lxml import html
html_content = """
<html>
<head><title>Sample Page</title></head>
<body>
<h1>Welcome to My Page</h1>
<p>This is a <a href="http://example.com">link</a> to an example site.</p>
</body>
</html>
"""
tree = html.fromstring(html_content)
title = tree.xpath('//title/text()') # 使用XPath获取title内容
print(title)
三、使用网络请求库:requests
-
使用requests获取HTML内容
requests库简化了HTTP请求的处理,并支持SSL验证、cookie等功能。
import requests
url = 'http://example.com'
response = requests.get(url)
if response.status_code == 200:
html_content = response.text
print("HTML content successfully retrieved.")
else:
print("Failed to retrieve content.")
-
结合BeautifulSoup解析请求内容
获取的HTML内容可以结合BeautifulSoup进行进一步解析和数据提取。
from bs4 import BeautifulSoup
url = 'http://example.com'
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.text, 'html.parser')
print(soup.prettify())
print(soup.title.string)
通过以上方式,Python可以轻松实现HTML内容的读取和解析。根据具体需求选择合适的库和方法,可以有效提高开发效率和代码的可维护性。
相关问答FAQs:
如何在Python中读取HTML内容?
在Python中,可以使用多种库读取HTML内容。最常用的库包括requests
和BeautifulSoup
。首先,使用requests
库获取网页的HTML内容,然后利用BeautifulSoup
解析这些内容,以便提取所需的信息。以下是一个简单的示例:
import requests
from bs4 import BeautifulSoup
url = 'https://example.com'
response = requests.get(url)
html_content = response.text
soup = BeautifulSoup(html_content, 'html.parser')
print(soup.prettify()) # 打印格式化后的HTML
这个过程可以帮助你获取网页内容并进行后续分析。
是否可以通过Python读取本地HTML文件?
确实可以。Python不仅可以读取在线HTML内容,也能够读取存储在本地的HTML文件。你可以使用内置的文件处理功能来打开文件,然后使用BeautifulSoup
进行解析。示例代码如下:
from bs4 import BeautifulSoup
with open('local_file.html', 'r', encoding='utf-8') as file:
html_content = file.read()
soup = BeautifulSoup(html_content, 'html.parser')
print(soup.title.string) # 打印HTML文件中的标题
这种方法适合处理不需要联网的HTML文件。
在读取HTML时如何处理编码问题?
编码问题是读取HTML时常见的挑战,尤其是当网页使用不同的字符编码时。为了确保正确解析HTML内容,可以在使用requests
库时指定编码,或者在读取本地文件时使用合适的编码格式。例如,使用response.encoding
来设置或获取响应的编码。以下是处理编码的代码示例:
import requests
url = 'https://example.com'
response = requests.get(url)
response.encoding = response.apparent_encoding # 自动检测编码
html_content = response.text
这样可以有效避免因编码问题导致的乱码或解析错误。
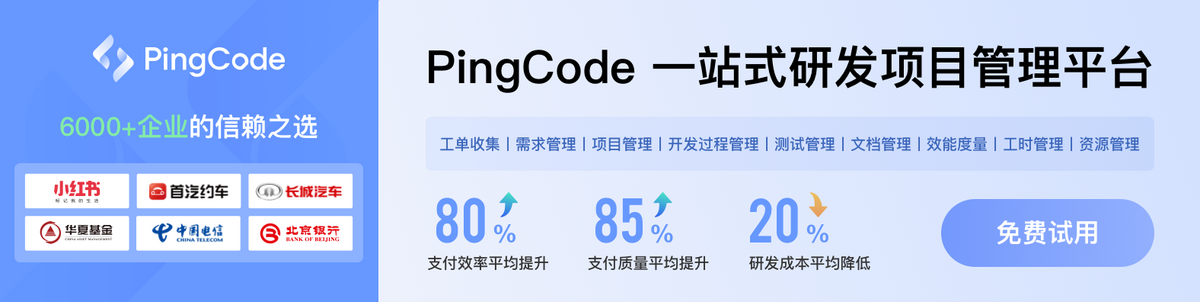