在Python中,统计行数的方法有多种,具体取决于数据的来源和格式。常见的方法包括使用文件读取的方法统计文本文件中的行数、使用Pandas库统计CSV或Excel文件中的行数、利用正则表达式进行复杂文本处理等。下面将详细介绍如何使用这些方法,其中一种方法是通过文件读取统计文本文件中的行数。
在Python中统计行数的基本方法是通过文件操作。可以使用内置的open()
函数打开文件,并利用readlines()
或迭代器来统计行数。以下是详细步骤:
一、使用文件操作统计文本文件的行数
通过Python内置的文件操作,可以很方便地统计文本文件中的行数。这对于处理纯文本文件非常有效。
1.1 使用 readlines()
方法
readlines()
方法将文件中的所有行读取到一个列表中,然后可以通过获取列表的长度来确定行数。
def count_lines_with_readlines(file_path):
with open(file_path, 'r') as file:
lines = file.readlines()
return len(lines)
file_path = 'example.txt'
line_count = count_lines_with_readlines(file_path)
print(f"Total lines (using readlines): {line_count}")
优势:readlines()
方法简单直观,适合处理小文件。
劣势:对于大文件,readlines()
会将整个文件内容加载到内存中,可能导致内存不足。
1.2 使用迭代器
使用文件对象本身作为迭代器,一次读取一行,可以有效减少内存使用。
def count_lines_with_iterator(file_path):
line_count = 0
with open(file_path, 'r') as file:
for _ in file:
line_count += 1
return line_count
file_path = 'example.txt'
line_count = count_lines_with_iterator(file_path)
print(f"Total lines (using iterator): {line_count}")
优势:适合处理大文件,因为只在内存中保留一行数据。
劣势:稍微复杂一些,但在处理大数据集时更高效。
二、使用Pandas统计CSV或Excel文件的行数
Pandas是Python中用于数据分析的强大库,提供了快速读取和处理表格数据的能力。
2.1 使用 Pandas 读取 CSV 文件
import pandas as pd
def count_lines_in_csv(file_path):
df = pd.read_csv(file_path)
return len(df)
file_path = 'example.csv'
line_count = count_lines_in_csv(file_path)
print(f"Total lines in CSV: {line_count}")
优势:处理结构化数据方便快捷,可以同时获取列数和其他统计信息。
劣势:对于非常大的CSV文件,Pandas读取速度可能较慢。
2.2 使用 Pandas 读取 Excel 文件
import pandas as pd
def count_lines_in_excel(file_path, sheet_name=0):
df = pd.read_excel(file_path, sheet_name=sheet_name)
return len(df)
file_path = 'example.xlsx'
line_count = count_lines_in_excel(file_path)
print(f"Total lines in Excel: {line_count}")
优势:支持多种格式的Excel文件,处理表格数据灵活。
劣势:需要依赖额外的库,如openpyxl
或xlrd
。
三、使用正则表达式统计行数
正则表达式可以用于复杂的文本处理,特别是在需要过滤特定格式行时。
3.1 统计符合特定格式的行数
import re
def count_lines_matching_pattern(file_path, pattern):
line_count = 0
with open(file_path, 'r') as file:
for line in file:
if re.match(pattern, line):
line_count += 1
return line_count
file_path = 'example.txt'
pattern = r'^Error:'
line_count = count_lines_matching_pattern(file_path, pattern)
print(f"Total lines matching pattern: {line_count}")
优势:可以灵活地根据需要统计特定类型的行。
劣势:正则表达式的编写可能较为复杂,尤其是对于不熟悉的人。
四、统计代码行数
对于开发者来说,统计代码行数是常见需求,可以通过以下方法实现。
4.1 使用 cloc
工具
cloc
是一个命令行工具,可以直接统计代码库中的行数,包括注释、空行和有效代码行。
cloc path/to/code
优势:无需编写代码,直接使用命令行工具。
劣势:需要安装额外的工具,无法在Python脚本中直接调用。
4.2 使用Python脚本分析代码行
def count_code_lines(file_path):
line_count = 0
with open(file_path, 'r') as file:
for line in file:
stripped_line = line.strip()
if stripped_line and not stripped_line.startswith('#'):
line_count += 1
return line_count
file_path = 'example.py'
line_count = count_code_lines(file_path)
print(f"Total code lines: {line_count}")
优势:可以自定义过滤规则,例如排除注释行。
劣势:需要手动编写过滤逻辑。
五、使用第三方库统计行数
除了内置方法和Pandas外,还有其他第三方库可以帮助统计行数。
5.1 使用 line_profiler
库
line_profiler
是一个Python性能分析工具,主要用于分析函数的执行时间,但也可以用于统计行数。
pip install line_profiler
优势:可以同时进行性能分析和行数统计。
劣势:主要用于性能分析,对统计行数的支持有限。
5.2 使用 numpy
加速大数据集的行数统计
在处理大数据集时,numpy
可以通过其向量化操作加速行数统计。
import numpy as np
def count_lines_with_numpy(file_path):
data = np.genfromtxt(file_path, delimiter=',', dtype=None)
return data.shape[0]
file_path = 'example.csv'
line_count = count_lines_with_numpy(file_path)
print(f"Total lines with numpy: {line_count}")
优势:对于特定格式的数据集,numpy
的效率非常高。
劣势:仅适用于结构化数据,且需要理解numpy
的用法。
六、总结
统计行数在数据处理和分析中是一个基础而又重要的任务。在Python中,根据数据的格式和规模,可以选择不同的方法:对于小型文本文件,直接使用文件操作;对于结构化数据,Pandas是首选;在处理大文件时,迭代器和numpy
提供了高效的解决方案。此外,正则表达式和自定义脚本可以帮助实现更复杂的行数统计需求。根据具体的应用场景选择合适的方法,可以显著提高数据处理的效率和准确性。
相关问答FAQs:
如何使用Python统计文本文件的行数?
在Python中,可以通过读取文件并使用readlines()
方法来统计行数。以下是一个简单的示例代码:
with open('your_file.txt', 'r') as file:
lines = file.readlines()
line_count = len(lines)
print(f'The number of lines is: {line_count}')
这种方法适用于小型文件,对于大型文件,使用循环逐行读取会更加高效。
使用Python统计CSV文件的行数的方法是什么?
可以使用Python的csv
模块来统计CSV文件的行数。示例如下:
import csv
with open('your_file.csv', 'r') as csvfile:
reader = csv.reader(csvfile)
line_count = sum(1 for row in reader)
print(f'The number of rows in the CSV file is: {line_count}')
这种方法可以有效地处理CSV文件并统计行数,而不会占用过多内存。
在Python中如何统计多种文件类型的行数?
可以通过编写一个函数来处理不同文件类型的行数统计。示例代码如下:
def count_lines(filename):
if filename.endswith('.txt'):
with open(filename, 'r') as file:
return len(file.readlines())
elif filename.endswith('.csv'):
with open(filename, 'r') as csvfile:
return sum(1 for row in csv.reader(csvfile))
else:
raise ValueError("Unsupported file type")
line_count_txt = count_lines('your_file.txt')
line_count_csv = count_lines('your_file.csv')
print(f'The number of lines in text file: {line_count_txt}')
print(f'The number of rows in CSV file: {line_count_csv}')
通过这种方法,可以轻松扩展以支持其他文件类型的行数统计。
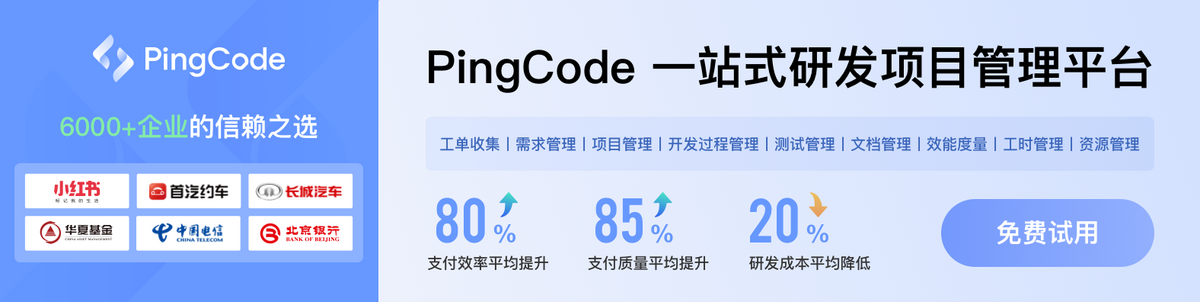