要在Python中引入math模块,可以使用import math
语句。这一模块提供了许多数学函数和常数,能够简化复杂的数学计算。引入math模块的步骤简单、函数种类丰富、提高计算精度。下面将详细介绍如何引入math模块及其应用。
引入math模块的方法
在Python程序中使用math模块非常简单,只需在代码的开头部分加入import math
即可。这样,程序就可以访问该模块中的所有函数和常量。例如,如果需要计算平方根,可以使用math.sqrt()
函数。
引入math模块不仅可以帮助程序员简化代码,还能提高程序的计算精度和效率。math模块提供了一系列的数学函数,如对数函数、指数函数、三角函数等,这些函数经过优化,可以处理各种数学计算。
一、MATH模块的基本使用
math模块提供了基本的数学运算功能,包括加减乘除、取整、求余等。这些基础功能在科学计算和工程应用中具有重要作用。
-
基本运算函数
Python的math模块中包含基本的数学运算函数,例如
math.ceil()
用于向上取整,math.floor()
用于向下取整,math.fabs()
用于计算绝对值等。这些函数可以帮助程序员在处理数值时提高精度和简化代码。import math
向上取整
result_ceil = math.ceil(4.2)
print("Ceil of 4.2:", result_ceil)
向下取整
result_floor = math.floor(4.8)
print("Floor of 4.8:", result_floor)
绝对值
result_fabs = math.fabs(-5)
print("Absolute value of -5:", result_fabs)
-
指数与对数运算
math模块提供了指数和对数运算函数,例如
math.exp()
用于计算e的指数,math.log()
用于计算对数。对数函数可以指定底数,默认是自然对数。import math
指数运算
result_exp = math.exp(2)
print("Exponential of 2:", result_exp)
对数运算
result_log = math.log(10, 10)
print("Logarithm of 10 base 10:", result_log)
二、MATH模块的三角函数
三角函数在图形学、物理学和工程学中有着广泛应用。math模块提供了丰富的三角函数,满足各种计算需求。
-
基本三角函数
math模块中包含基本的三角函数,如
math.sin()
、math.cos()
、math.tan()
,用于计算正弦、余弦和正切值。函数接受弧度为单位的输入。import math
正弦
result_sin = math.sin(math.pi/2)
print("Sine of π/2:", result_sin)
余弦
result_cos = math.cos(0)
print("Cosine of 0:", result_cos)
正切
result_tan = math.tan(math.pi/4)
print("Tangent of π/4:", result_tan)
-
反三角函数
除了基本三角函数,math模块还提供了反三角函数,如
math.asin()
、math.acos()
、math.atan()
,用于计算反正弦、反余弦和反正切值。import math
反正弦
result_asin = math.asin(1)
print("Arcsine of 1:", result_asin)
反余弦
result_acos = math.acos(0)
print("Arccosine of 0:", result_acos)
反正切
result_atan = math.atan(1)
print("Arctangent of 1:", result_atan)
三、MATH模块的常数
math模块中还定义了一些常用的数学常数,如π(pi)、e,这些常数在科学计算中至关重要。
-
数学常数
在使用math模块时,可以直接访问数学常数
math.pi
(π)和math.e
(自然对数的底)。这些常数提高了代码的可读性和精确度。import math
常数 π
pi_value = math.pi
print("Value of π:", pi_value)
常数 e
e_value = math.e
print("Value of e:", e_value)
-
常数的应用
数学常数在很多科学计算中都是不可或缺的。例如,在计算圆的面积或圆周长时,需要用到π;在计算复利增长时,需要用到e。
import math
计算圆的面积
radius = 5
area = math.pi * radius 2
print("Area of circle with radius 5:", area)
计算复利
principal = 1000
rate = 0.05
time = 10
amount = principal * math.e (rate * time)
print("Compound amount after 10 years:", amount)
四、MATH模块的特殊函数
math模块还提供了一些特殊的数学函数,主要用于高级数学运算。
-
双曲函数
math模块包含双曲函数,如
math.sinh()
、math.cosh()
、math.tanh()
,用于计算双曲正弦、双曲余弦和双曲正切。import math
双曲正弦
result_sinh = math.sinh(1)
print("Hyperbolic sine of 1:", result_sinh)
双曲余弦
result_cosh = math.cosh(1)
print("Hyperbolic cosine of 1:", result_cosh)
双曲正切
result_tanh = math.tanh(1)
print("Hyperbolic tangent of 1:", result_tanh)
-
阶乘与组合函数
对于需要进行组合数学运算的场合,math模块提供了
math.factorial()
用于计算阶乘,math.comb()
用于计算组合数。import math
阶乘
result_factorial = math.factorial(5)
print("Factorial of 5:", result_factorial)
组合
result_comb = math.comb(5, 2)
print("Combination of 5 choose 2:", result_comb)
五、MATH模块的应用案例
通过几个实际案例,说明如何将math模块应用于解决实际问题。
-
计算抛物线的顶点
在解析几何中,抛物线的顶点坐标可以通过math模块进行计算。例如,计算方程y=ax^2+bx+c的顶点。
import math
抛物线参数
a = 1
b = -4
c = 4
计算顶点
h = -b / (2 * a)
k = a * h 2 + b * h + c
print("Vertex of the parabola:", (h, k))
-
计算复利
复利计算在金融领域非常重要,math模块中的指数函数可以帮助进行精确计算。
import math
复利参数
principal = 1000
rate = 0.05
time = 10
计算复利
amount = principal * math.e (rate * time)
interest = amount - principal
print("Interest earned after 10 years:", interest)
通过对math模块的深入了解,程序员可以在Python中更高效地进行数学计算,提高代码的性能和可读性。无论是基础数学运算、三角函数、还是复杂的科学计算,math模块都提供了强大的支持。
相关问答FAQs:
如何在Python中导入math模块?
要在Python中使用math模块,您只需在代码的开头添加一行代码:import math
。这行代码将math模块引入到您的程序中,使您能够使用其中的各种数学函数和常量,例如平方根、对数和圆周率等。
math模块中有哪些常用的数学函数?
math模块提供了多种实用的数学函数,包括但不限于:math.sqrt()
(计算平方根)、math.factorial()
(计算阶乘)、math.sin()
和math.cos()
(计算三角函数)、math.log()
(计算自然对数)以及常量math.pi
(圆周率)和math.e
(自然常数)。这些函数可以帮助您处理多种数学运算。
math模块与其他数学库相比有什么优势?
math模块是Python标准库的一部分,因此使用它不需要额外安装,适合进行基本的数学计算。与NumPy或SciPy等外部库相比,math模块更为轻量,适合小型项目或简单的数学运算。如果您的项目需要处理复杂的数组或矩阵运算,NumPy可能是更好的选择,但对于基本的数学功能,math模块已经足够。
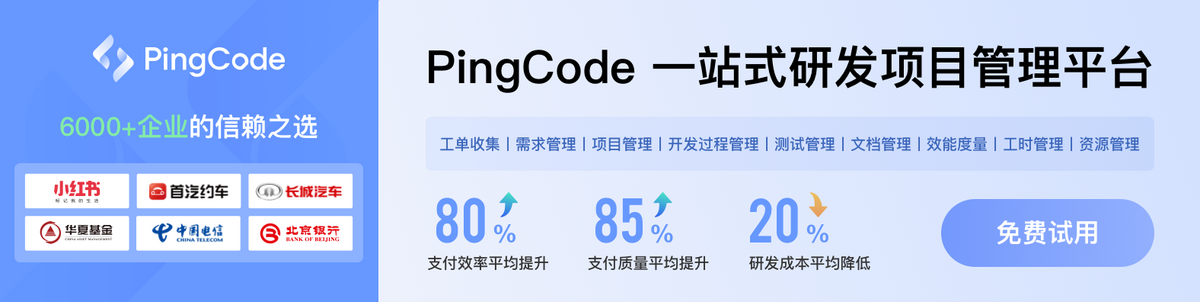