Python绘制树的方式有多种,可以使用图形库matplotlib、graphviz等工具来实现。通过使用这些工具,可以轻松绘制出树状结构、灵活自定义树的样式、多样的可视化效果。其中,matplotlib是一个非常流行的绘图库,适用于生成静态、交互式、动画图形;graphviz则是一个专门用于创建图形的工具,尤其擅长绘制复杂的有向图和无向图。下面我们将详细介绍如何在Python中使用这些工具绘制树。
一、MATPLOTLIB绘制树
Matplotlib是Python中最常用的绘图库之一,适用于各种图形绘制。虽然它不是专门为树结构设计的,但通过一些技巧,我们可以使用它来绘制简单的树。
- 基本概念和准备工作
在使用matplotlib绘制树之前,首先需要了解树的数据结构。在Python中,树通常可以用嵌套的列表或字典来表示。每个节点可以是一个字典,包含节点的名称以及其子节点的列表。
tree_data = {
'Root': {
'Left': {},
'Right': {
'Right-Left': {},
'Right-Right': {}
}
}
}
在安装matplotlib库后,我们就可以开始绘制树了。
- 绘制树的实现
为了绘制树,我们需要定义一个递归函数,遍历树的每个节点,并使用matplotlib的绘图函数来绘制节点和边。
import matplotlib.pyplot as plt
def plot_node(node_txt, center_pt, parent_pt, node_type):
plt.annotate(node_txt, xy=parent_pt, xycoords='axes fraction',
xytext=center_pt, textcoords='axes fraction',
va="center", ha="center", bbox=node_type, arrowprops=dict(arrowstyle="<-"))
def plot_tree(my_tree, parent_pt, node_txt):
num_leafs = get_num_leafs(my_tree)
depth = get_tree_depth(my_tree)
first_str = list(my_tree.keys())[0]
cntr_pt = (plot_tree.x_off + (1.0 + float(num_leafs)) / 2.0 / plot_tree.total_w, plot_tree.y_off)
plot_node(first_str, cntr_pt, parent_pt, decision_node)
second_dict = my_tree[first_str]
plot_tree.y_off = plot_tree.y_off - 1.0 / plot_tree.total_d
for key in second_dict.keys():
if type(second_dict[key]).__name__ == 'dict':
plot_tree(second_dict[key], cntr_pt, str(key))
else:
plot_tree.x_off = plot_tree.x_off + 1.0 / plot_tree.total_w
plot_node(second_dict[key], (plot_tree.x_off, plot_tree.y_off), cntr_pt, leaf_node)
plot_mid_text((plot_tree.x_off, plot_tree.y_off), cntr_pt, str(key))
plot_tree.y_off = plot_tree.y_off + 1.0 / plot_tree.total_d
def create_plot(in_tree):
fig = plt.figure(1, facecolor='white')
fig.clf()
axprops = dict(xticks=[], yticks=[])
create_plot.ax1 = plt.subplot(111, frameon=False, axprops)
plot_tree.total_w = float(get_num_leafs(in_tree))
plot_tree.total_d = float(get_tree_depth(in_tree))
plot_tree.x_off = -0.5 / plot_tree.total_w
plot_tree.y_off = 1.0
plot_tree(in_tree, (0.5, 1.0), '')
plt.show()
定义节点格式
decision_node = dict(boxstyle="sawtooth", fc="0.8")
leaf_node = dict(boxstyle="round4", fc="0.8")
create_plot(tree_data)
- 优化树的样式
通过调整节点的样式和颜色,可以使树的外观更加美观。使用matplotlib的各种参数可以自定义节点的形状、颜色、箭头样式等。
decision_node = dict(boxstyle="sawtooth", fc="0.8", edgecolor='black')
leaf_node = dict(boxstyle="round4", fc="0.8", edgecolor='blue')
二、GRAPHVIZ绘制树
Graphviz是一个更适合用于绘制树和图形的工具。它可以轻松生成高质量的图形,并且支持多种输出格式。
- 安装和基本使用
首先,需要安装graphviz和相应的Python接口。可以通过pip安装:
pip install graphviz
安装完成后,可以使用graphviz来绘制树。
- 定义和绘制树
Graphviz使用DOT语言来定义图形。我们可以通过Python接口来生成DOT文件,然后渲染成图形。
from graphviz import Digraph
def draw_tree(tree, graph=None, parent_name=None):
if graph is None:
graph = Digraph()
for name, subtree in tree.items():
graph.node(name)
if parent_name is not None:
graph.edge(parent_name, name)
draw_tree(subtree, graph, name)
return graph
tree_data = {
'Root': {
'Left': {},
'Right': {
'Right-Left': {},
'Right-Right': {}
}
}
}
graph = draw_tree(tree_data)
graph.render('tree', format='png', cleanup=True)
- 定制图的样式
Graphviz提供了丰富的样式选项,可以定制节点和边的样式。
graph.node(name, shape='box', style='filled', color='lightblue')
graph.edge(parent_name, name, color='red', style='dashed')
三、其他工具和方法
除了matplotlib和graphviz,还有其他方法可以在Python中绘制树。例如,使用第三方库如networkx可以更方便地处理复杂的图形结构。或者通过开发GUI应用程序来实现动态的树可视化。
- NetworkX库
NetworkX是一个用于创建、操作和研究复杂网络结构的Python库。它可以与matplotlib结合使用,绘制出复杂的图形和网络。
import matplotlib.pyplot as plt
import networkx as nx
G = nx.DiGraph()
tree_data = {
'Root': ['Left', 'Right'],
'Right': ['Right-Left', 'Right-Right']
}
for parent, children in tree_data.items():
for child in children:
G.add_edge(parent, child)
pos = nx.spring_layout(G)
nx.draw(G, pos, with_labels=True, node_size=2000, node_color='lightblue', font_size=10, font_weight='bold')
plt.show()
- Tkinter库
Tkinter是Python的标准GUI库,可以用于创建简单的图形用户界面。通过Tkinter绘制树,可以实现更为动态和交互式的可视化效果。
总结来说,在Python中绘制树可以使用多种工具和方法。选择适合的工具取决于具体需求,如图形复杂度、输出格式、交互性等。通过合理的选择和使用这些工具,可以有效地实现树的可视化,并从中获得直观的结构和数据关系的展示。
相关问答FAQs:
如何在Python中绘制树形结构?
在Python中,可以使用多种库来绘制树形结构,例如Matplotlib、NetworkX和Graphviz等。Matplotlib可以通过递归绘制树的节点和边,NetworkX则提供了方便的图形表示和布局算法,而Graphviz可以生成高质量的图形输出。您可以根据自己的需求选择合适的库。
使用哪个库来绘制树最简单?
对于初学者来说,Matplotlib可能是最简单的选择,因为它具有直观的API和丰富的文档支持。通过简单的代码,您可以快速绘制出基本的树形结构。如果需要处理更复杂的图形,NetworkX和Graphviz提供了更强大的功能,但学习曲线相对陡峭。
如何处理树节点之间的关系?
在绘制树形结构时,树的节点与节点之间的关系通常通过边来表示。您可以使用字典或类的实例来表示节点及其子节点的关系。对于复杂树形结构,使用递归函数来遍历树并绘制节点和边是一种有效的方法。这种方法可以确保树的层次结构得到准确体现。
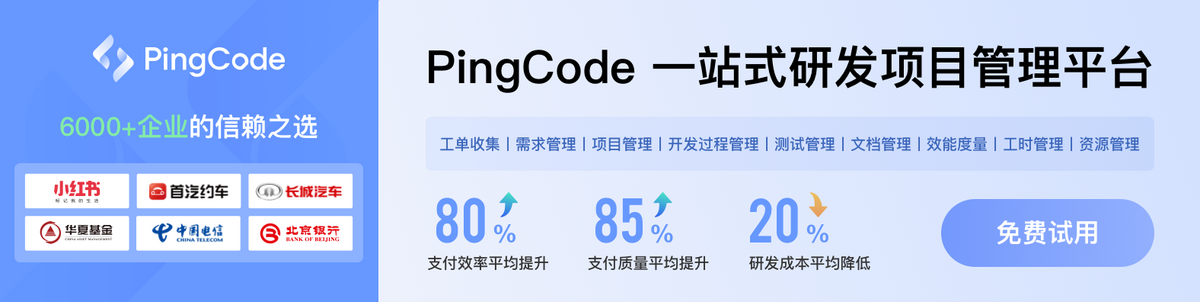