要在Python中绘制党旗,可以使用turtle库、matplotlib库或PIL库等。每种方法都有其独特的实现方式和优缺点。使用turtle库较为直观、使用matplotlib库绘制精细图形效果更好、使用PIL库可以进行更多图形处理。接下来,我们将详细介绍使用这三种库绘制党旗的过程。
一、使用TURTLE库绘制党旗
Turtle库是Python内置的一个非常适合入门的图形绘制库,特别适合用来绘制简单的图形和动画。
- 安装并设置Turtle库
Python自带turtle库,无需安装。可以通过以下代码导入并设置窗口大小与背景颜色:
import turtle
turtle.setup(800, 500)
turtle.bgcolor('red')
- 绘制五角星
中国共产党党旗上的主要元素是五角星,我们可以通过自定义函数来绘制五角星。
def draw_star(x, y, length):
turtle.penup()
turtle.goto(x, y)
turtle.pendown()
turtle.setheading(0)
turtle.forward(length)
angle = 144
for _ in range(5):
turtle.right(angle)
turtle.forward(length)
- 绘制党旗
接下来,通过控制五角星的位置和大小来绘制整个党旗。
def draw_party_flag():
turtle.color('yellow')
draw_star(-350, 150, 100) # 大五角星
turtle.setheading(0)
draw_star(-200, 200, 50) # 右上小五角星
draw_star(-150, 160, 50) # 右下小五角星
draw_star(-150, 90, 50) # 左上小五角星
draw_star(-200, 50, 50) # 左下小五角星
- 执行绘制
最后,我们调用绘制函数并显示图形。
turtle.speed(10)
draw_party_flag()
turtle.done()
二、使用MATPLOTLIB库绘制党旗
Matplotlib是Python中最常用的绘图库之一,适用于更精细的图形绘制。
- 安装并设置Matplotlib库
首先,需要确保安装了matplotlib库,可以通过pip安装:
pip install matplotlib
在代码中导入库并设置图形背景:
import matplotlib.pyplot as plt
import numpy as np
- 定义五角星函数
使用坐标变换绘制五角星:
def draw_star(ax, center, size):
ax.add_patch(plt.Polygon(
star_points(center, size),
edgecolor='yellow', facecolor='yellow'
))
def star_points(center, size):
x, y = center
points = []
for i in range(5):
angle = i * 144
points.append((x + size * np.cos(np.radians(angle)),
y + size * np.sin(np.radians(angle))))
return points
- 绘制党旗
通过调整五角星的中心坐标和大小绘制党旗:
fig, ax = plt.subplots()
ax.set_xlim(0, 800)
ax.set_ylim(0, 500)
ax.set_facecolor('red')
ax.set_aspect('equal')
draw_star(ax, (150, 350), 50) # 大五角星
draw_star(ax, (300, 400), 20) # 右上小五角星
draw_star(ax, (350, 350), 20) # 右下小五角星
draw_star(ax, (350, 250), 20) # 左上小五角星
draw_star(ax, (300, 200), 20) # 左下小五角星
plt.axis('off')
plt.show()
三、使用PIL库绘制党旗
PIL(Pillow)是Python的图像处理库,适合更复杂的图像处理任务。
- 安装并设置PIL库
首先,需要确保安装了Pillow库,可以通过pip安装:
pip install pillow
在代码中导入库并创建图形对象:
from PIL import Image, ImageDraw
img = Image.new('RGB', (800, 500), color='red')
draw = ImageDraw.Draw(img)
- 定义五角星函数
通过极坐标转换计算五角星的顶点位置:
def draw_star(draw, center, size):
cx, cy = center
points = []
for i in range(5):
angle = i * 144
x = cx + size * np.cos(np.radians(angle))
y = cy - size * np.sin(np.radians(angle))
points.append((x, y))
draw.polygon(points, fill='yellow')
- 绘制党旗
通过调整五角星的中心坐标和大小绘制党旗:
draw_star(draw, (150, 150), 100) # 大五角星
draw_star(draw, (300, 300), 50) # 右上小五角星
draw_star(draw, (350, 250), 50) # 右下小五角星
draw_star(draw, (350, 150), 50) # 左上小五角星
draw_star(draw, (300, 100), 50) # 左下小五角星
img.show()
通过以上方法,可以在Python中使用不同的库来绘制党旗。根据具体需求选择合适的库和方法,每种方法都有其独特的优势和适用场景。
相关问答FAQs:
如何使用Python绘制党旗的基本步骤是什么?
要使用Python绘制党旗,您可以使用图形库如Matplotlib或PIL(Pillow)。首先,确定党旗的颜色和形状,然后使用绘图函数绘制矩形、圆形等图形。通过设置合适的颜色和比例,可以实现党旗的效果。具体可以参考以下步骤:导入库、创建画布、绘制背景颜色、添加标志和文字,最后展示或保存图像。
在绘制党旗时,如何选择合适的颜色和尺寸?
选择颜色时,应参考党旗的官方色彩标准,以确保颜色的准确性。对于尺寸,可以根据最终使用的场景来决定,常见的比例有3:2或2:1等。在绘制时,确保图形元素的比例和位置符合党旗的设计规范,以体现其象征意义。
使用Python绘制党旗是否需要掌握高级编程技巧?
不需要掌握高级编程技巧。即使是初学者也能通过简单的代码实现党旗的绘制。了解基本的Python语法以及如何使用图形库就足够了。网络上有许多教程和示例代码,可以帮助您快速上手,逐步实现绘制党旗的目标。
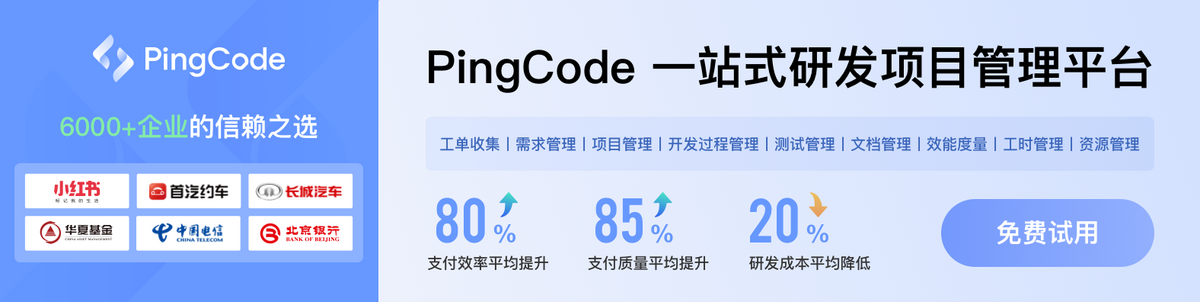