在Python中统计词频可以通过多种方法实现,如使用字典、collections模块中的Counter类、正则表达式等。其中,使用collections.Counter类、字典、自然语言处理工具包(如NLTK)是常见的方法。本文将详细介绍这些方法并提供代码示例。
在使用collections模块的Counter类时,首先需要将文本分割成单词列表,然后调用Counter类进行统计,这是最简单且高效的方法之一。Counter会自动统计每个单词出现的次数,并返回一个字典形式的数据结构,其中键是单词,值是出现的次数。通过这种方式,可以快速了解到文本中各个单词的频率。
一、使用字典统计词频
使用字典是统计词频的基本方法之一。这种方法直接、清晰,适合初学者。
1.1 创建字典并统计
首先,需要将文本分割成单词列表。然后,遍历这个列表,将每个单词作为键存入字典中,同时更新其出现次数。
def count_word_frequency(text):
words = text.split()
frequency = {}
for word in words:
if word in frequency:
frequency[word] += 1
else:
frequency[word] = 1
return frequency
text = "this is a test. this test is only a test."
print(count_word_frequency(text))
1.2 处理不同形式的单词
在统计词频时,需要考虑大小写和标点符号的影响。通常会将所有单词转换为小写,并去除标点符号。
import string
def clean_text(text):
# 将文本转换为小写,并去除标点符号
text = text.lower()
return text.translate(str.maketrans('', '', string.punctuation))
def count_word_frequency(text):
text = clean_text(text)
words = text.split()
frequency = {}
for word in words:
if word in frequency:
frequency[word] += 1
else:
frequency[word] = 1
return frequency
text = "This is a test. This test is only a test!"
print(count_word_frequency(text))
二、使用collections.Counter统计词频
Counter类是collections模块中的一个子类,用于计数可哈希对象。它是统计词频的高效工具。
2.1 使用Counter统计
Counter类接受一个可迭代对象作为输入,并返回一个字典形式的对象,其中键是可迭代对象中的元素,值是元素出现的次数。
from collections import Counter
def count_word_frequency(text):
words = text.split()
return Counter(words)
text = "this is a test. this test is only a test."
print(count_word_frequency(text))
2.2 使用Counter处理复杂文本
与字典方法类似,在处理复杂文本时,需要先清理文本,然后使用Counter进行统计。
import string
from collections import Counter
def clean_text(text):
text = text.lower()
return text.translate(str.maketrans('', '', string.punctuation))
def count_word_frequency(text):
text = clean_text(text)
words = text.split()
return Counter(words)
text = "This is a test. This test is only a test!"
print(count_word_frequency(text))
三、使用正则表达式统计词频
正则表达式是处理文本的强大工具,适合用于复杂文本的清理和分割。
3.1 使用正则表达式分割文本
正则表达式可以用于分割文本为单词列表,同时去除不必要的字符。
import re
from collections import Counter
def count_word_frequency(text):
# 使用正则表达式将文本分割成单词
words = re.findall(r'\w+', text.lower())
return Counter(words)
text = "This is a test. This test is only a test!"
print(count_word_frequency(text))
3.2 处理复杂的文本格式
对于包含数字、特殊字符等复杂格式的文本,正则表达式能够灵活地提取出有意义的单词。
import re
from collections import Counter
def count_word_frequency(text):
# \w+ 匹配任意字母、数字及下划线的序列
words = re.findall(r'\w+', text.lower())
return Counter(words)
text = "Python 3.8 is great, isn't it? Yes, it's great!"
print(count_word_frequency(text))
四、使用自然语言处理工具包(NLTK)
NLTK(Natural Language Toolkit)是一个强大的自然语言处理库,提供了丰富的工具用于文本分析。
4.1 使用NLTK进行词频统计
NLTK提供了分词、去停用词、词形还原等多种功能,能够更准确地统计词频。
import nltk
from nltk.tokenize import word_tokenize
from nltk.corpus import stopwords
from collections import Counter
下载必要的NLTK资源
nltk.download('punkt')
nltk.download('stopwords')
def count_word_frequency(text):
# 分词
words = word_tokenize(text.lower())
# 去停用词
words = [word for word in words if word.isalpha() and word not in stopwords.words('english')]
return Counter(words)
text = "This is a test. This test is only a test!"
print(count_word_frequency(text))
4.2 处理词形还原
词形还原(Lemmatization)是将词的不同形态还原到基态,这对统计词频非常有用。
import nltk
from nltk.tokenize import word_tokenize
from nltk.corpus import stopwords
from nltk.stem import WordNetLemmatizer
from collections import Counter
下载必要的NLTK资源
nltk.download('punkt')
nltk.download('stopwords')
nltk.download('wordnet')
def count_word_frequency(text):
lemmatizer = WordNetLemmatizer()
words = word_tokenize(text.lower())
words = [lemmatizer.lemmatize(word) for word in words if word.isalpha() and word not in stopwords.words('english')]
return Counter(words)
text = "This is a test. This test is only a test!"
print(count_word_frequency(text))
五、总结
统计词频是文本分析中的基本步骤,可以通过多种方法实现。使用collections.Counter是最为简洁高效的方法,而正则表达式和NLTK提供了更多的文本处理能力。选择合适的方法取决于具体的应用场景和文本复杂度。无论选择哪种方法,首先要进行文本的预处理,包括去除标点符号、大小写统一、去停用词等,以保证统计结果的准确性。
相关问答FAQs:
如何使用Python进行词频统计?
在Python中,可以使用内置的collections
模块中的Counter
类来统计文本中的词频。首先,需要将文本分割成单词,然后使用Counter
来计算每个单词出现的次数。以下是一个简单的示例代码:
from collections import Counter
text = "这是一个简单的文本,文本中包含一些重复的单词。"
words = text.split() # 将文本分割成单词
word_count = Counter(words) # 统计单词频率
print(word_count)
处理中文文本时需要注意什么?
在处理中文文本时,直接使用split()
方法可能无法有效分割单词。可以使用第三方库如jieba
来进行中文分词。使用jieba
可以提高词频统计的准确性,示例如下:
import jieba
from collections import Counter
text = "这是一个简单的文本,文本中包含一些重复的单词。"
words = jieba.cut(text) # 使用jieba进行中文分词
word_count = Counter(words)
print(word_count)
如何将词频统计结果保存到文件中?
将词频统计结果保存到文件中,可以使用Python的文件操作功能。可以将词频结果写入一个文本文件,格式可以根据需求进行调整。示例如下:
with open('word_frequency.txt', 'w', encoding='utf-8') as f:
for word, count in word_count.items():
f.write(f"{word}: {count}\n")
通过这些方法,您可以轻松地使用Python统计文本中的词频,并进行进一步的数据分析。
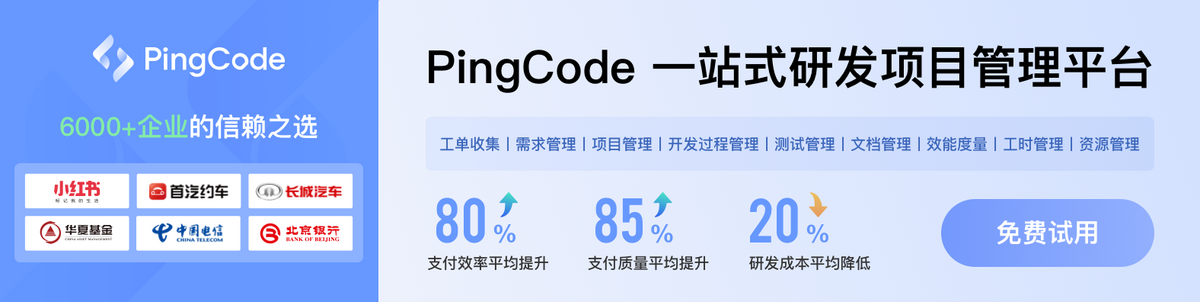