在Python中终止线程的方法有:使用线程标志、使用threading.Event
对象、使用concurrent.futures.ThreadPoolExecutor
、使用第三方库Thread
。在这些方法中,推荐使用线程标志的方式来安全地终止线程,因为直接强制终止线程可能会导致未释放资源、数据不一致等问题。下面将详细介绍这些方法。
一、使用线程标志
线程标志是一种比较安全和常用的方法。我们可以在线程中设置一个标志变量,在线程的循环中不断检查这个标志变量的值,如果标志变量变为True,线程就会安全地退出。
- 设置线程标志
在这种方法中,我们需要在线程中设置一个标志变量,并且在循环中不断检查这个标志变量的状态。当需要终止线程时,改变标志变量的值即可。这样可以确保线程在安全的情况下终止。
import threading
import time
class MyThread(threading.Thread):
def __init__(self):
super(MyThread, self).__init__()
self._stop_event = threading.Event()
def run(self):
while not self._stop_event.is_set():
print("Thread is running...")
time.sleep(1)
def stop(self):
self._stop_event.set()
使用
thread = MyThread()
thread.start()
time.sleep(5)
thread.stop()
thread.join()
在这个例子中,MyThread
类有一个_stop_event
属性,它是一个threading.Event
对象。在线程的run
方法中,使用is_set
方法检查这个事件是否被设置。如果是,则退出循环,从而安全地终止线程。stop
方法用于设置这个事件。
- 使用条件变量
条件变量可以用于线程之间的通信,帮助我们在某些条件下终止线程。
import threading
import time
class MyThread(threading.Thread):
def __init__(self):
super(MyThread, self).__init__()
self.condition = threading.Condition()
self.running = True
def run(self):
while self.running:
with self.condition:
self.condition.wait(timeout=1)
print("Thread is running...")
def stop(self):
with self.condition:
self.running = False
self.condition.notify()
使用
thread = MyThread()
thread.start()
time.sleep(5)
thread.stop()
thread.join()
在这个例子中,使用了threading.Condition
对象和notify
方法来通知线程终止。在run
方法中,通过wait
方法来等待终止信号,stop
方法中通过设置running
为False
并调用notify
方法来终止线程。
二、使用concurrent.futures.ThreadPoolExecutor
ThreadPoolExecutor
是Python标准库中的一个类,用于管理线程池。通过使用线程池,我们可以更好地控制线程的生命周期。
- 使用
ThreadPoolExecutor
管理线程
通过使用ThreadPoolExecutor
,我们可以轻松地管理多个线程的执行和终止。
from concurrent.futures import ThreadPoolExecutor
import time
def task():
while True:
print("Thread is running...")
time.sleep(1)
使用
executor = ThreadPoolExecutor(max_workers=1)
future = executor.submit(task)
time.sleep(5)
executor.shutdown(wait=False)
在这个例子中,ThreadPoolExecutor
被用来管理线程。通过submit
方法提交任务,并使用shutdown
方法来终止线程池中的所有线程。
三、使用第三方库Thread
Thread
是一个第三方库,支持更高级的线程控制功能。它提供了一种更加安全和简单的方式来终止线程。
- 使用
Thread
库
通过使用Thread
库,我们可以更加简便地控制线程的开始和终止。
from thread import Thread
import time
def task():
while True:
print("Thread is running...")
time.sleep(1)
使用
thread = Thread(target=task)
thread.start()
time.sleep(5)
thread.stop()
在这个例子中,Thread
库提供了一个简单的stop
方法来终止线程。通过调用stop
方法,可以安全地终止线程的执行。
四、总结
在Python中,终止线程的方法有很多种,包括使用线程标志、threading.Event
对象、concurrent.futures.ThreadPoolExecutor
和第三方库Thread
等。在实际开发中,推荐使用线程标志的方式来安全地终止线程。这种方法通过在循环中检查标志变量的状态,确保线程在安全的情况下终止,避免资源泄露和数据不一致的问题。希望通过本文的介绍,您能够更好地理解和使用Python中的线程终止技术。
相关问答FAQs:
在Python中,如何安全地停止一个正在运行的线程?
停止一个线程的最佳方法是使用标志位。创建一个全局变量作为标志,让线程在执行循环时检查这个标志。如果标志被设置为True,线程就可以安全退出。这样可以确保线程能够干净利落地结束,而不会造成资源泄露或数据不一致。
使用threading
模块时,如何管理线程的生命周期?
在使用threading
模块时,可以通过join()
方法等待线程完成。启动线程后,可以在主线程中调用join()
,这样主线程会阻塞,直到目标线程执行完成。此外,使用守护线程(daemon thread)也是一种方法,守护线程会在主程序结束时自动终止。
如果直接使用Thread
的_stop()
方法,可能会有什么风险?
直接调用_stop()
方法是不推荐的,因为这会导致线程立即停止,而不进行任何清理工作。这种方式可能会引发资源泄露、锁未释放等问题,进而影响程序的稳定性和数据的一致性。因此,安全的终止线程方式应始终优先考虑。
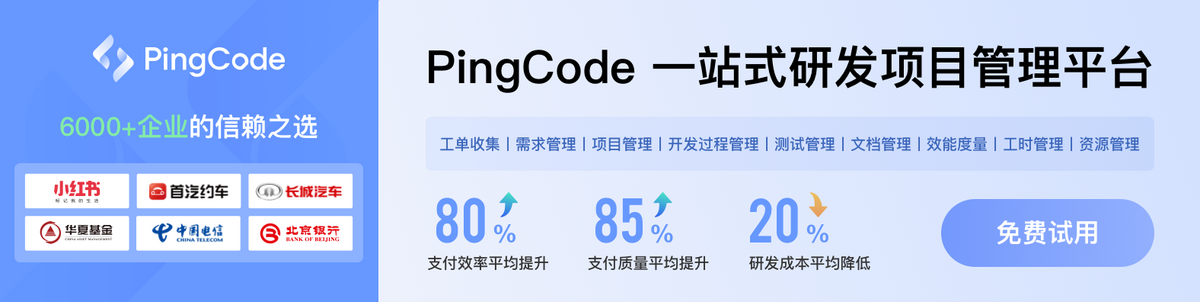