Python访问API的方式有多种,其中常用的有:使用requests库进行HTTP请求、使用第三方库处理特定API、使用异步库提高效率。其中,requests库是最常用的方式,因为它简单易用,适用于大多数HTTP请求。接下来,我们将详细介绍如何通过requests库访问API,并探讨其他方式。
一、使用REQUESTS库进行HTTP请求
requests库是Python中最流行的HTTP库之一,提供了简单的API来发送HTTP请求并处理响应。
- 安装requests库
首先,确保安装了requests库。可以通过以下命令安装:
pip install requests
- 发送GET请求
GET请求是最常用的HTTP请求方法之一,通常用于从服务器获取数据。
import requests
url = 'https://api.example.com/data'
response = requests.get(url)
if response.status_code == 200:
data = response.json()
print(data)
else:
print(f"Request failed with status code {response.status_code}")
在这个例子中,我们发送了一个GET请求到指定的URL,并检查响应的状态码。如果状态码为200,表示请求成功,我们可以使用response.json()
方法将响应内容解析为JSON格式的数据。
- 发送POST请求
POST请求通常用于向服务器提交数据。
import requests
url = 'https://api.example.com/submit'
payload = {'key1': 'value1', 'key2': 'value2'}
response = requests.post(url, data=payload)
if response.status_code == 200:
print("Data submitted successfully")
else:
print(f"Submission failed with status code {response.status_code}")
在这个例子中,我们发送了一个POST请求,提交了一个字典作为数据负载。
- 添加请求头
有时候需要在请求中添加额外的头信息,例如API密钥或用户代理。
import requests
url = 'https://api.example.com/protected'
headers = {'Authorization': 'Bearer YOUR_API_KEY'}
response = requests.get(url, headers=headers)
if response.status_code == 200:
data = response.json()
print(data)
else:
print(f"Request failed with status code {response.status_code}")
在这个例子中,我们在请求头中添加了一个授权头,以便访问受保护的资源。
二、使用第三方库处理特定API
有时,我们需要访问特定的API,这些API可能有相应的第三方库提供更高层次的封装。
- Google API
Google提供了一系列API,用于访问其服务(如Google Drive、Google Sheets等)。可以使用google-api-python-client
库访问这些API。
pip install google-api-python-client
然后根据具体API的文档进行配置和访问。
- Twitter API
Twitter提供了Twitter API,用于访问其数据。可以使用tweepy
库进行访问。
pip install tweepy
然后根据Twitter API的文档进行配置和访问。
三、使用异步库提高效率
在处理需要大量请求的场景下,使用异步库可以提高效率。aiohttp是一个流行的异步HTTP客户端库。
- 安装aiohttp
pip install aiohttp
- 使用aiohttp发送异步请求
import aiohttp
import asyncio
async def fetch_data(url):
async with aiohttp.ClientSession() as session:
async with session.get(url) as response:
return await response.json()
async def main():
url = 'https://api.example.com/data'
data = await fetch_data(url)
print(data)
loop = asyncio.get_event_loop()
loop.run_until_complete(main())
在这个例子中,我们使用aiohttp
库发送异步GET请求,并使用asyncio
管理异步任务。
四、错误处理和异常捕获
在访问API时,处理错误和异常是非常重要的。以下是一些常见的错误处理策略:
- 检查HTTP响应状态码
总是检查HTTP响应的状态码,以确保请求成功。
if response.status_code != 200:
print(f"Error: Received status code {response.status_code}")
- 捕获请求异常
使用try-except块捕获请求异常。
import requests
try:
response = requests.get('https://api.example.com/data')
response.raise_for_status() # Raises an HTTPError for bad responses
except requests.exceptions.RequestException as e:
print(f"Request failed: {e}")
五、API速率限制与重试机制
许多API都有速率限制,限制客户端在特定时间内可以发送的请求数量。为了避免超出速率限制,可以实现重试机制。
- 了解速率限制
阅读API文档,了解速率限制的具体规则。
- 实现重试机制
使用time.sleep()
函数在请求失败时进行重试。
import time
import requests
url = 'https://api.example.com/data'
for _ in range(3): # Retry up to 3 times
try:
response = requests.get(url)
response.raise_for_status()
break # Exit loop if request is successful
except requests.exceptions.RequestException as e:
print(f"Request failed: {e}, retrying...")
time.sleep(2) # Wait 2 seconds before retrying
六、处理API响应数据
根据API返回的数据格式,选择合适的方法进行解析。
- JSON格式
许多API返回的数据是JSON格式,可以使用response.json()
方法进行解析。
data = response.json()
- XML格式
如果API返回的是XML格式,可以使用xml.etree.ElementTree
库进行解析。
import xml.etree.ElementTree as ET
tree = ET.fromstring(response.content)
七、总结
Python提供了多种方式来访问API,无论是通过简单的requests库,还是使用异步库或特定API的第三方库。在实际开发中,选择合适的工具和方法至关重要。此外,处理错误、实现重试机制以及解析响应数据都是成功访问API的关键。通过本文的介绍,您应该能够在各种场景下有效地使用Python访问API。
相关问答FAQs:
如何使用Python进行API请求?
使用Python访问API通常依赖于requests
库。首先确保已安装该库,可以通过命令pip install requests
进行安装。随后,可以通过以下基本示例发起GET请求:
import requests
response = requests.get('https://api.example.com/data')
if response.status_code == 200:
data = response.json()
print(data)
else:
print(f"Error: {response.status_code}")
这个例子展示了如何获取API的数据,并检查请求的状态。
在Python中如何处理API的身份验证?
许多API需要身份验证才能访问数据。可以通过在请求中添加头部信息来实现。以下是一个使用Bearer Token的示例:
headers = {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN'
}
response = requests.get('https://api.example.com/secure-data', headers=headers)
确保将YOUR_ACCESS_TOKEN
替换为实际的访问令牌。
如何在Python中处理API返回的错误?
处理API返回错误是确保程序稳定性的重要环节。可以通过检查HTTP状态码来判断请求是否成功。例如:
if response.status_code == 200:
data = response.json()
elif response.status_code == 404:
print("Error: Resource not found.")
elif response.status_code == 500:
print("Error: Server error.")
else:
print(f"Error: {response.status_code}")
这种方法能够帮助你快速定位问题并采取相应措施。
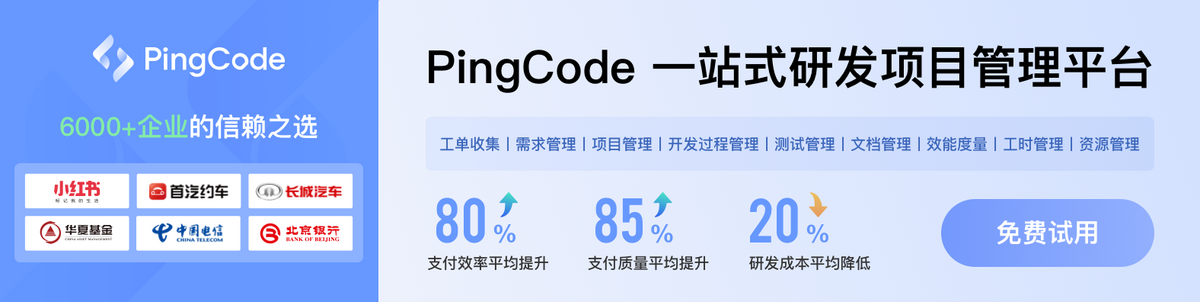