在Python中,匹配多条件可以通过使用逻辑运算符、列表解析、过滤函数如filter()等方法实现、Python提供了灵活且强大的工具来实现复杂条件匹配、通过构建自定义函数和使用第三方库可以进一步扩展匹配能力。 下面详细介绍其中的一种方法,即使用逻辑运算符进行多条件匹配。
一、使用逻辑运算符进行多条件匹配
Python中最基本的多条件匹配方式是通过逻辑运算符,如and
、or
和not
。这些运算符可以帮助我们在一个条件语句中组合多个条件。例如,如果我们想同时检查一个变量是否大于10并且小于20,我们可以使用如下代码:
x = 15
if x > 10 and x < 20:
print("x is between 10 and 20")
1.1 使用 and
逻辑运算符
and
运算符用于连接多个条件,如果所有条件都为真,整体结果才为真。它在数据筛选和条件判断中非常常用。以下是一个示例:
age = 25
income = 50000
if age > 18 and income > 30000:
print("Eligible for loan")
在这个例子中,只有当age
大于18和income
大于30000时,才会打印出“Eligible for loan”。
1.2 使用 or
逻辑运算符
or
运算符用于连接多个条件,只要有一个条件为真,整体结果就为真。它适用于需要至少一个条件成立的情况:
day = "Saturday"
if day == "Saturday" or day == "Sunday":
print("It's the weekend!")
在这个示例中,只要day
是“Saturday”或“Sunday”,就会输出"It's the weekend!"。
1.3 使用 not
逻辑运算符
not
运算符用于反转条件的真假值,适用于需要排除某些条件的情况:
is_raining = False
if not is_raining:
print("Let's go for a walk!")
在这个例子中,如果is_raining
为假(即没有下雨),就会输出"Let's go for a walk!"。
二、使用列表解析和条件表达式
列表解析是一种简洁而强大的Python语法,可以用于创建新的列表。它可以结合条件表达式,实现多条件过滤和匹配:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
filtered_numbers = [x for x in numbers if x > 2 and x < 8]
print(filtered_numbers)
在这个例子中,filtered_numbers
将包含所有大于2且小于8的数字。
2.1 结合多个条件使用
列表解析可以结合多个条件,通过and
或or
等逻辑运算符实现复杂条件的过滤:
words = ["apple", "banana", "cherry", "date"]
selected_words = [word for word in words if len(word) > 5 or 'a' in word]
print(selected_words)
在此示例中,selected_words
包含所有长度大于5或包含字母'a'的单词。
2.2 嵌套条件
列表解析还支持嵌套条件,使得可以在一个表达式中进行更复杂的匹配:
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
flatten = [num for row in matrix for num in row if num % 2 == 0]
print(flatten)
在这个例子中,flatten
是一个包含所有偶数的列表,这些偶数是从一个二维矩阵中提取出来的。
三、使用过滤函数(filter)
filter()
函数是Python内置的高阶函数,用于根据指定条件过滤可迭代对象。它可以与lambda表达式结合使用,以实现简洁的条件匹配:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
filtered_numbers = list(filter(lambda x: x % 2 == 0, numbers))
print(filtered_numbers)
在这个例子中,filtered_numbers
将包含所有偶数。
3.1 结合多个条件
filter()
函数可以结合多个条件,通过在lambda表达式中使用逻辑运算符实现:
people = [{'name': 'Alice', 'age': 25}, {'name': 'Bob', 'age': 30}, {'name': 'Charlie', 'age': 35}]
selected_people = list(filter(lambda person: person['age'] > 25 and 'a' in person['name'].lower(), people))
print(selected_people)
在这个示例中,selected_people
将包含所有名字中包含字母'a'且年龄大于25的人。
3.2 自定义过滤条件
除了简单的条件表达式,filter()
函数还可以结合自定义函数,实现更复杂的条件匹配:
def is_prime(n):
if n <= 1:
return False
for i in range(2, n):
if n % i == 0:
return False
return True
numbers = range(1, 20)
prime_numbers = list(filter(is_prime, numbers))
print(prime_numbers)
在这个例子中,prime_numbers
将包含所有素数,这些素数是通过自定义的is_prime
函数筛选出来的。
四、使用第三方库进行复杂条件匹配
对于更复杂的条件匹配需求,Python的第三方库如pandas
和numpy
提供了强大的数据处理和分析能力,可以有效地处理多条件匹配。
4.1 使用 pandas
进行数据过滤
pandas
是一个用于数据分析的强大库,提供了灵活的数据筛选和处理方法。以下是一个基于pandas
的示例:
import pandas as pd
data = {'Name': ['Alice', 'Bob', 'Charlie', 'David'],
'Age': [25, 30, 35, 40],
'Salary': [50000, 60000, 70000, 80000]}
df = pd.DataFrame(data)
筛选年龄大于30且工资大于60000的行
filtered_df = df[(df['Age'] > 30) & (df['Salary'] > 60000)]
print(filtered_df)
在这个例子中,filtered_df
将包含所有年龄大于30且工资大于60000的行。
4.2 使用 numpy
进行条件匹配
numpy
是一个用于科学计算的库,提供了强大的数组处理功能。以下是一个基于numpy
的条件匹配示例:
import numpy as np
array = np.array([10, 15, 20, 25, 30, 35, 40])
condition = (array > 20) & (array < 35)
filtered_array = array[condition]
print(filtered_array)
在这个例子中,filtered_array
将包含所有大于20且小于35的元素。
五、构建自定义函数和类进行条件匹配
对于需要高度定制化的条件匹配,构建自定义函数和类是一个有效的方法。这种方法可以将条件逻辑封装在函数或类中,便于重用和维护。
5.1 构建自定义函数
通过定义自定义函数,可以将复杂的条件逻辑封装在一个函数中,提高代码的可读性和重用性:
def is_eligible_for_discount(customer):
return customer['loyalty_points'] > 1000 and customer['years_with_company'] > 5
customers = [{'name': 'Alice', 'loyalty_points': 1200, 'years_with_company': 6},
{'name': 'Bob', 'loyalty_points': 800, 'years_with_company': 4},
{'name': 'Charlie', 'loyalty_points': 1500, 'years_with_company': 7}]
eligible_customers = filter(is_eligible_for_discount, customers)
print(list(eligible_customers))
在这个例子中,is_eligible_for_discount
函数用于判断客户是否符合折扣条件。
5.2 构建自定义类
通过定义自定义类,可以将条件逻辑和数据结构结合在一起,便于复杂条件的管理:
class Customer:
def __init__(self, name, loyalty_points, years_with_company):
self.name = name
self.loyalty_points = loyalty_points
self.years_with_company = years_with_company
def is_eligible_for_discount(self):
return self.loyalty_points > 1000 and self.years_with_company > 5
customers = [Customer('Alice', 1200, 6), Customer('Bob', 800, 4), Customer('Charlie', 1500, 7)]
eligible_customers = [customer for customer in customers if customer.is_eligible_for_discount()]
print([customer.name for customer in eligible_customers])
在这个例子中,Customer
类封装了客户信息和折扣条件逻辑,并提供了is_eligible_for_discount
方法用于条件判断。
通过以上方法,Python可以灵活地实现多条件匹配,满足不同场景下的需求。无论是简单的逻辑运算,还是复杂的数据分析,Python都提供了强大的工具和库,帮助开发者高效地进行条件匹配和数据处理。
相关问答FAQs:
在Python中,如何使用逻辑运算符实现多条件匹配?
在Python中,可以通过逻辑运算符and
、or
和not
来实现多条件匹配。例如,如果你想检查一个数字是否在特定范围内,你可以使用and
运算符将多个条件结合在一起。示例代码如下:
number = 10
if number > 5 and number < 15:
print("数字在范围内")
通过这种方式,可以实现复杂的条件判断。
使用Python的正则表达式如何进行多条件匹配?
正则表达式是处理字符串匹配的强大工具。在Python中,可以使用re
模块来实现多条件匹配。例如,如果想要匹配以字母“a”开头并包含数字的字符串,可以这样写:
import re
pattern = r'^a.*\d'
string = 'abc123'
if re.match(pattern, string):
print("字符串匹配成功")
这种方式非常适合处理复杂的字符串匹配需求。
是否可以使用列表或字典来实现多条件匹配?
当然可以!使用列表或字典可以使代码更加简洁明了。例如,可以使用列表来存储多个条件,并通过循环或列表推导式进行匹配。以下是一个示例:
conditions = [1, 2, 3]
value = 2
if value in conditions:
print("值在条件列表中")
这种方法在处理动态条件时特别有用,提高了代码的灵活性。
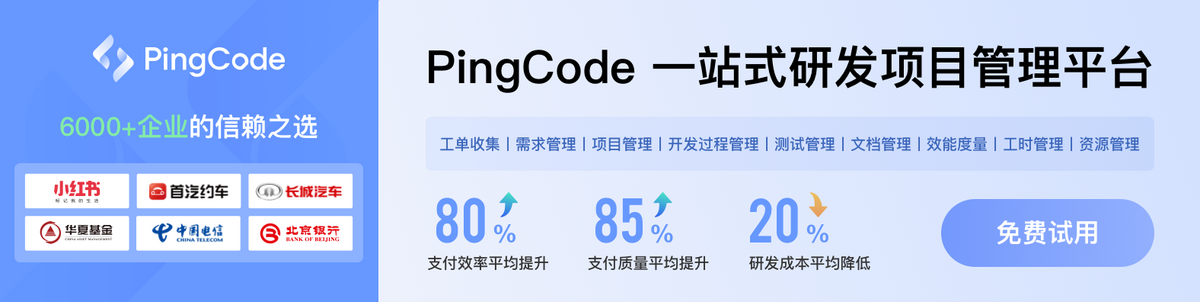