开头段落:
Python批量导入图片的方法包括使用os模块遍历文件夹、借助PIL库进行图像处理、结合glob模块进行文件模式匹配。其中,os模块遍历文件夹是最基础的方法,它通过操作系统的文件管理功能,能够轻松实现对文件夹中所有图片的批量导入。使用os模块,可以轻松获取文件夹中的文件列表,并通过循环逐一读取图片文件。此外,结合PIL库(Pillow),还可以对导入的图片进行处理,比如调整大小、格式转换等。而glob模块则可以通过文件模式匹配,快速选择特定格式的图片进行导入。接下来,将详细介绍这些方法及其在实际应用中的具体实现。
一、OS模块遍历文件夹
os模块是Python中用于操作系统功能的标准库之一,它提供了与文件系统交互的多种方法。通过os模块,我们可以轻松获取文件夹中的文件列表,从而实现批量导入图片。
- 获取文件列表
使用os模块中的os.listdir()
方法,可以获取指定目录下的所有文件和文件夹的名称。结合os.path
模块,可以进一步筛选出特定格式的文件(如.jpg、.png等)。
import os
def get_image_files(directory):
return [f for f in os.listdir(directory) if os.path.isfile(os.path.join(directory, f)) and f.endswith(('.jpg', '.png'))]
image_files = get_image_files('/path/to/directory')
- 读取图片文件
通过循环遍历文件列表,可以使用PIL库或其他图像处理库来逐一读取图片文件。
from PIL import Image
for image_file in image_files:
image_path = os.path.join('/path/to/directory', image_file)
image = Image.open(image_path)
# 对图片进行操作
image.show()
二、PIL库进行图像处理
PIL(Python Imaging Library)是Python的一个强大的图像处理库,其现代版本为Pillow。PIL库不仅可以帮助我们读取图片,还可以对图片进行多种处理。
- 安装Pillow库
要使用PIL库,我们需要先安装Pillow库,可以通过以下命令安装:
pip install Pillow
- 图像读取与操作
使用Pillow,我们可以轻松读取图片,并进行多种图像操作,例如调整大小、旋转、裁剪等。
from PIL import Image
image = Image.open('/path/to/image.jpg')
调整大小
resized_image = image.resize((800, 600))
旋转图片
rotated_image = image.rotate(90)
保存修改后的图片
rotated_image.save('/path/to/rotated_image.jpg')
三、GLOB模块进行文件模式匹配
glob模块是Python标准库中用于文件模式匹配的工具,它允许我们使用通配符来匹配特定格式的文件,非常适合用于批量导入特定格式的图片。
- 使用glob模块获取图片文件列表
通过使用glob模块,我们可以轻松获取指定目录下符合特定模式的文件列表。
import glob
image_files = glob.glob('/path/to/directory/*.jpg') + glob.glob('/path/to/directory/*.png')
- 批量处理图片
获取文件列表后,可以结合PIL库对图片进行批量处理。
from PIL import Image
for image_file in image_files:
image = Image.open(image_file)
# 对图片进行操作
image.show()
四、结合多种方法实现批量导入与处理
在实际应用中,我们可以结合os模块、PIL库和glob模块,实现更加灵活和高效的批量导入与处理图片的功能。
- 批量调整图片大小
通过os模块或glob模块获取图片列表后,可以使用Pillow库对所有图片进行批量调整大小。
from PIL import Image
import os
def batch_resize_images(directory, size=(800, 600)):
image_files = [f for f in os.listdir(directory) if f.endswith(('.jpg', '.png'))]
for image_file in image_files:
image_path = os.path.join(directory, image_file)
image = Image.open(image_path)
resized_image = image.resize(size)
resized_image.save(image_path)
batch_resize_images('/path/to/directory')
- 批量格式转换
可以通过Pillow库将图片批量转换为其他格式,例如将所有.jpg图片转换为.png格式。
from PIL import Image
import os
def batch_convert_images(directory, target_format='png'):
image_files = [f for f in os.listdir(directory) if f.endswith('.jpg')]
for image_file in image_files:
image_path = os.path.join(directory, image_file)
image = Image.open(image_path)
new_image_path = os.path.splitext(image_path)[0] + f'.{target_format}'
image.save(new_image_path, target_format.upper())
batch_convert_images('/path/to/directory')
五、处理大批量图片的性能优化
在处理大批量图片时,可能会遇到性能瓶颈。我们可以采取一些措施来优化性能,提高程序的执行效率。
- 使用多线程或多进程
通过使用Python的concurrent.futures
模块,可以轻松实现多线程或多进程处理图片,从而提高处理速度。
from concurrent.futures import ThreadPoolExecutor
from PIL import Image
import os
def process_image(image_path):
image = Image.open(image_path)
# 对图片进行操作
image.show()
def batch_process_images(directory):
image_files = [os.path.join(directory, f) for f in os.listdir(directory) if f.endswith(('.jpg', '.png'))]
with ThreadPoolExecutor() as executor:
executor.map(process_image, image_files)
batch_process_images('/path/to/directory')
- 优化I/O操作
批量处理图片时,I/O操作可能成为瓶颈。可以通过减少不必要的I/O操作,或使用更高效的存储介质(如SSD)来提高性能。
总之,Python提供了多种工具和库,可以轻松实现批量导入图片的功能。在实际应用中,可以根据需求选择合适的方法,并结合多种方法进行优化,以提高程序的效率和灵活性。
相关问答FAQs:
如何在Python中批量导入图片?
在Python中,批量导入图片通常涉及使用库如PIL(Pillow)或OpenCV。这些库可以帮助你读取、处理和保存图片。首先,需要安装相关库,例如使用pip install Pillow
。然后,可以通过遍历文件夹中的图片文件,使用Image.open()
函数来逐一导入每张图片。
可以使用哪些库来处理批量导入的图片?
处理批量导入图片的常用库包括Pillow、OpenCV和scikit-image。Pillow适合处理常见的图像格式并进行基本的图像处理;OpenCV则更加强大,适合进行复杂的图像处理和计算机视觉任务;而scikit-image则适用于科学计算和图像分析。根据你的需求选择适合的库将会更高效。
如何确保导入的图片格式兼容?
在批量导入图片时,确保格式兼容是很重要的。可以使用Pillow库中的Image.open()
来打开图片,并在处理之前检查图片的格式。使用img.format
可以获取当前图片的格式信息。如果需要转换格式,可以使用img.save('output_image.jpg')
来将图片保存为所需的格式,从而确保兼容性。
如何处理批量导入的图片并保存?
处理批量导入的图片可以通过循环遍历每个文件,使用图像处理函数进行操作,比如调整大小、旋转或应用滤镜。处理完毕后,可以使用img.save()
方法将每张处理后的图片保存到指定目录。可以结合使用os
库来创建文件夹和管理路径,使得处理和保存过程更加高效。
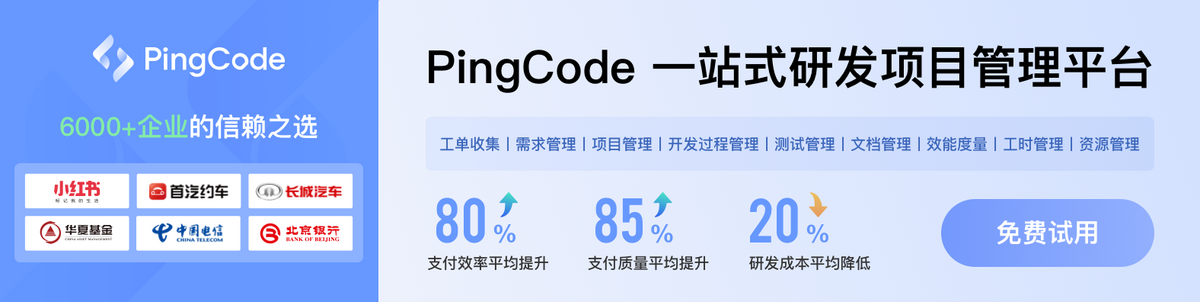