Python可以通过多线程、多进程和异步编程等方式并发执行Shell命令。 其中,多线程适合I/O密集型任务、多进程适合CPU密集型任务,而异步编程则可以在处理大量I/O操作时提高效率。使用subprocess
模块结合concurrent.futures
库是实现并发执行Shell命令的常见方法之一。下面将详细介绍这几种方法,并比较其优缺点。
一、多线程执行Shell命令
Python的threading
模块可以创建多个线程来并发执行任务。虽然Python的全局解释器锁(GIL)限制了多线程的CPU并行性能,但在I/O密集型任务中,多线程仍能提升效率。
- 使用ThreadPoolExecutor
concurrent.futures.ThreadPoolExecutor
可以轻松管理线程池并发执行任务。
import subprocess
from concurrent.futures import ThreadPoolExecutor
def run_command(command):
result = subprocess.run(command, shell=True, stdout=subprocess.PIPE, stderr=subprocess.PIPE)
return result.stdout, result.stderr
commands = ["ls", "echo 'Hello World'", "pwd"]
with ThreadPoolExecutor(max_workers=3) as executor:
futures = [executor.submit(run_command, cmd) for cmd in commands]
for future in futures:
stdout, stderr = future.result()
print("STDOUT:", stdout.decode())
print("STDERR:", stderr.decode())
在这个例子中,我们创建了一个线程池,执行多个Shell命令,并收集输出。
- 适用场景
多线程适用于I/O密集型任务,例如网络请求、文件操作等。由于GIL的限制,多线程不适合CPU密集型任务。
二、多进程执行Shell命令
多进程可以绕过GIL限制,充分利用多核CPU的能力。
- 使用ProcessPoolExecutor
concurrent.futures.ProcessPoolExecutor
可以创建进程池来并发执行任务。
import subprocess
from concurrent.futures import ProcessPoolExecutor
def run_command(command):
result = subprocess.run(command, shell=True, stdout=subprocess.PIPE, stderr=subprocess.PIPE)
return result.stdout, result.stderr
commands = ["ls", "echo 'Hello World'", "pwd"]
with ProcessPoolExecutor(max_workers=3) as executor:
futures = [executor.submit(run_command, cmd) for cmd in commands]
for future in futures:
stdout, stderr = future.result()
print("STDOUT:", stdout.decode())
print("STDERR:", stderr.decode())
在这个例子中,ProcessPoolExecutor
创建了一个进程池,执行Shell命令,并获取输出。
- 适用场景
多进程适合CPU密集型任务,如数据处理、计算密集型操作等。
三、异步编程执行Shell命令
异步编程通过asyncio
库可以有效地处理大量I/O操作。
- 使用asyncio和subprocess
asyncio
结合subprocess
模块可以异步执行Shell命令。
import asyncio
async def run_command(command):
proc = await asyncio.create_subprocess_shell(
command,
stdout=asyncio.subprocess.PIPE,
stderr=asyncio.subprocess.PIPE
)
stdout, stderr = await proc.communicate()
return stdout, stderr
async def main():
commands = ["ls", "echo 'Hello World'", "pwd"]
tasks = [run_command(cmd) for cmd in commands]
results = await asyncio.gather(*tasks)
for stdout, stderr in results:
print("STDOUT:", stdout.decode())
print("STDERR:", stderr.decode())
asyncio.run(main())
在这个例子中,我们使用asyncio.create_subprocess_shell
异步执行Shell命令。
- 适用场景
异步编程适合需要处理大量I/O操作的场景,如网络编程、文件读取等。
四、比较与选择
-
性能
- 多线程:适合I/O密集型任务,但受GIL限制。
- 多进程:适合CPU密集型任务,无GIL限制。
- 异步编程:适合大量I/O操作,性能较优。
-
复杂性
- 多线程:相对简单,但需注意线程安全。
- 多进程:较复杂,需管理进程间通信。
- 异步编程:需要理解异步编程模型,代码结构变化较大。
-
资源消耗
- 多线程:资源消耗较少。
- 多进程:消耗更多内存和CPU资源。
- 异步编程:资源消耗低,适合高并发。
根据具体需求选择合适的并发执行方式。在I/O密集型任务中,多线程和异步编程是不错的选择,而在CPU密集型任务中,多进程可能更具优势。理解每种方法的特点和适用场景,有助于编写高效的并发程序。
相关问答FAQs:
1. 如何在Python中实现并发执行Shell命令?
在Python中,可以使用subprocess
模块结合concurrent.futures
模块来实现并发执行Shell命令。通过创建一个线程池或进程池,可以同时运行多个Shell命令。例如,使用ThreadPoolExecutor
可以很方便地提交多个任务,并在后台并行执行这些Shell命令。代码示例如下:
import subprocess
from concurrent.futures import ThreadPoolExecutor
def run_command(command):
result = subprocess.run(command, shell=True, capture_output=True, text=True)
return result.stdout
commands = ["ls", "pwd", "echo 'Hello World'"]
with ThreadPoolExecutor() as executor:
results = executor.map(run_command, commands)
for output in results:
print(output)
2. 使用Python并发执行Shell命令时,如何处理错误和异常?
在并发执行Shell命令时,处理错误和异常非常重要。可以在run_command
函数中添加异常处理,捕获subprocess.CalledProcessError
或其他相关异常,并根据需要记录错误信息或进行重试。例如:
def run_command(command):
try:
result = subprocess.run(command, shell=True, check=True, capture_output=True, text=True)
return result.stdout
except subprocess.CalledProcessError as e:
return f"Error executing {command}: {e.stderr}"
3. 并发执行Shell命令对系统资源的影响如何评估?
并发执行Shell命令可能会消耗大量系统资源,特别是在命令执行时间较长或资源消耗较高的情况下。可以使用psutil
库来监控系统的CPU和内存使用情况。建议在执行高并发任务时,合理设置线程或进程的数量,以避免系统过载。监控资源使用的代码示例如下:
import psutil
def monitor_resources():
cpu_usage = psutil.cpu_percent(interval=1)
memory_info = psutil.virtual_memory()
return cpu_usage, memory_info.percent
# 在执行命令前后调用 monitor_resources() 进行监控
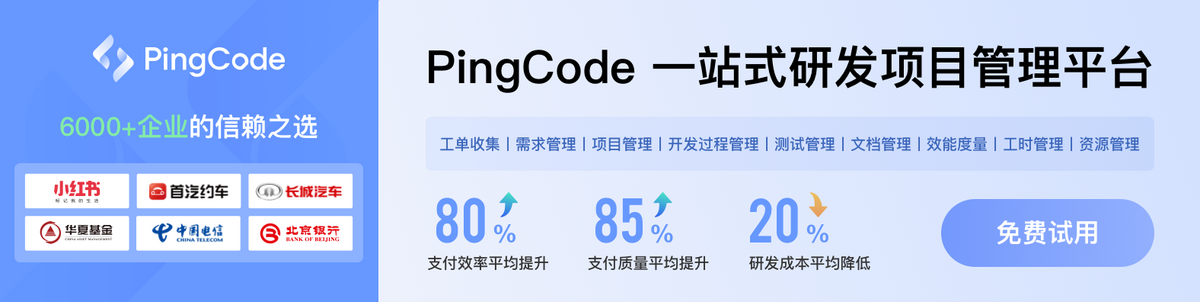