Python自动删除文件的方法包括:使用os模块、使用shutil模块、使用pathlib模块。通过os模块,您可以使用os.remove()函数删除文件;通过shutil模块,可以使用shutil.rmtree()来删除目录及其内容;通过pathlib模块,您可以使用Path对象的unlink()方法来删除文件。下面将详细介绍如何使用这些方法来实现自动删除文件。
一、使用OS模块
Python的os模块提供了一种简单的方法来与操作系统进行交互,其中包括删除文件。os.remove()函数可以用于删除指定路径的文件。
- os.remove()函数
os.remove()是一个内置函数,用于删除指定路径的文件。使用时需要提供文件的路径。如果文件不存在,则会引发FileNotFoundError异常。
import os
def delete_file(file_path):
try:
os.remove(file_path)
print(f"File {file_path} has been deleted successfully.")
except FileNotFoundError:
print(f"File {file_path} not found.")
except Exception as e:
print(f"Error occurred while deleting file: {e}")
示例
delete_file('path/to/your/file.txt')
- 删除目录
os模块还提供了os.rmdir()用于删除空目录。如果目录不为空,则需要使用shutil模块的rmtree()函数。
import os
def delete_directory(dir_path):
try:
os.rmdir(dir_path)
print(f"Directory {dir_path} has been deleted successfully.")
except FileNotFoundError:
print(f"Directory {dir_path} not found.")
except OSError:
print(f"Directory {dir_path} is not empty, cannot be deleted.")
except Exception as e:
print(f"Error occurred while deleting directory: {e}")
示例
delete_directory('path/to/your/directory')
二、使用SHUTIL模块
shutil模块提供了一些高级的文件操作功能,比如复制、移动和删除文件或目录。对于目录,shutil.rmtree()函数可以递归删除目录及其内容。
- shutil.rmtree()函数
shutil.rmtree()可以删除一个目录及其所有内容,即使目录不为空。
import shutil
def delete_directory_with_contents(dir_path):
try:
shutil.rmtree(dir_path)
print(f"Directory {dir_path} and all its contents have been deleted successfully.")
except FileNotFoundError:
print(f"Directory {dir_path} not found.")
except Exception as e:
print(f"Error occurred while deleting directory: {e}")
示例
delete_directory_with_contents('path/to/your/directory')
三、使用PATHLIB模块
pathlib模块是Python 3.4引入的新模块,提供了一种面向对象的方式来处理文件和目录路径。Path对象的unlink()方法可以用于删除文件。
- Path.unlink()方法
Path.unlink()方法用于删除文件。它是pathlib模块中Path对象的方法。
from pathlib import Path
def delete_file_with_pathlib(file_path):
path = Path(file_path)
try:
path.unlink()
print(f"File {file_path} has been deleted successfully.")
except FileNotFoundError:
print(f"File {file_path} not found.")
except Exception as e:
print(f"Error occurred while deleting file: {e}")
示例
delete_file_with_pathlib('path/to/your/file.txt')
- Path.rmdir()方法
Path.rmdir()方法用于删除空目录。如果目录不为空,则会引发OSError异常。
from pathlib import Path
def delete_empty_directory_with_pathlib(dir_path):
path = Path(dir_path)
try:
path.rmdir()
print(f"Directory {dir_path} has been deleted successfully.")
except FileNotFoundError:
print(f"Directory {dir_path} not found.")
except OSError:
print(f"Directory {dir_path} is not empty, cannot be deleted.")
except Exception as e:
print(f"Error occurred while deleting directory: {e}")
示例
delete_empty_directory_with_pathlib('path/to/your/directory')
四、错误处理与日志记录
在实际应用中,删除文件时可能会遇到各种错误,因此在代码中处理可能的异常是很重要的。同时,记录删除操作的日志有助于问题排查。
- 错误处理
在删除文件或目录时,常见的错误包括FileNotFoundError(文件或目录不存在)、PermissionError(权限不足)和OSError(删除非空目录时使用os.rmdir()引发)。
- 日志记录
可以使用Python的logging模块记录文件删除操作的日志信息。
import logging
import os
logging.basicConfig(filename='file_operations.log', level=logging.INFO)
def delete_file_with_logging(file_path):
try:
os.remove(file_path)
logging.info(f"File {file_path} has been deleted successfully.")
except FileNotFoundError:
logging.error(f"File {file_path} not found.")
except PermissionError:
logging.error(f"Permission denied to delete {file_path}.")
except Exception as e:
logging.error(f"Error occurred while deleting file: {e}")
示例
delete_file_with_logging('path/to/your/file.txt')
五、自动化任务
在实际应用中,自动删除文件可以通过调度任务来实现。例如,可以使用Python的schedule库或操作系统的计划任务功能来定期执行文件删除操作。
- 使用schedule库
schedule库可以用于调度定期任务。可以使用它来实现每天、每周或每月自动删除文件。
import schedule
import time
def job():
delete_file('path/to/your/file.txt')
每天的特定时间执行任务
schedule.every().day.at("10:30").do(job)
while True:
schedule.run_pending()
time.sleep(1)
- 使用操作系统的计划任务
在Windows上,可以使用任务计划程序(Task Scheduler)来定期执行Python脚本。在Linux上,可以使用cron作业来实现相同的功能。
综上所述,Python提供了多种方法来自动删除文件和目录。通过os模块、shutil模块和pathlib模块,您可以根据具体需求选择合适的方法。在实际应用中,结合错误处理、日志记录和自动化任务调度,可以更有效地管理文件删除操作。
相关问答FAQs:
如何使用Python实现文件自动删除的功能?
在Python中,可以使用内置的os
模块和os.remove()
函数来实现文件的自动删除。通过编写一个简单的脚本,指定需要删除的文件路径,程序就能够在满足特定条件时自动删除该文件。此外,使用os.path.exists()
函数可以确保在删除文件之前先检查文件是否存在,从而避免可能的错误。
Python中是否有定时删除文件的解决方案?
是的,可以结合time
模块和循环结构实现定时删除文件的功能。通过设置一个循环,指定删除文件的时间间隔,例如每隔一小时检查一次文件是否需要被删除。还可以结合datetime
模块来设置特定的日期和时间进行删除操作,从而实现更精确的控制。
在文件删除过程中如何处理异常情况?
在删除文件时,可能会遇到权限不足、文件不存在等异常情况。可以使用try-except
语句来捕捉这些异常,确保程序在运行时不会中断。例如,如果尝试删除一个不存在的文件,程序可以捕捉到FileNotFoundError
,并输出相应的错误信息,而不是让程序崩溃。通过这种方式,可以提高代码的健壮性和用户体验。
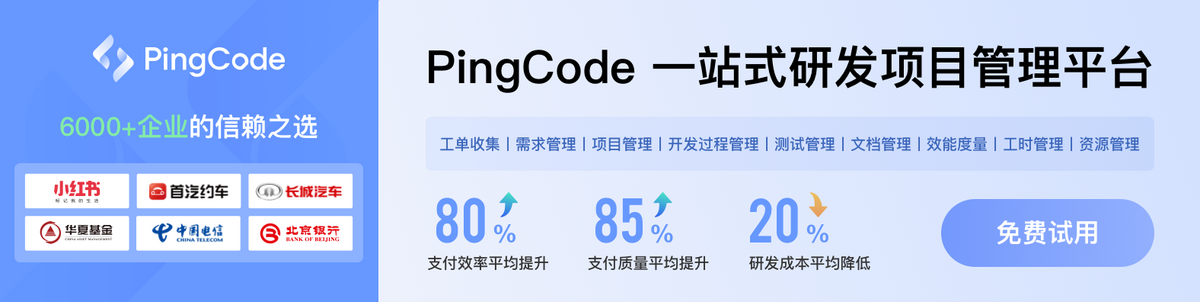