要在Python中打印购物小票,您可以使用以下几种方法:直接打印文本、使用库生成格式化文本、生成PDF或图像格式的小票、连接打印机打印。为了实现这一目标,您可以结合Python的文本处理能力和第三方库,生成格式化的小票,并通过合适的方式输出。接下来,我将详细介绍如何使用这些方法逐步实现购物小票的打印。
一、文本格式化打印
-
基本文本格式化
使用Python的字符串操作功能,您可以创建一个基本的文本格式化购物小票。这种方法最简单,但在格式美观和功能上有局限。
def print_receipt(items, total):
print("==== 购物小票 ====")
for item, price in items.items():
print(f"{item}: \t¥{price}")
print("===================")
print(f"总计: \t¥{total}")
items = {'苹果': 5, '香蕉': 3, '牛奶': 10}
total = sum(items.values())
print_receipt(items, total)
-
使用字符串模板
Python的
str.format()
或f-string
可以用来更灵活地格式化字符串:def format_receipt(items, total):
receipt = "==== 购物小票 ====\n"
for item, price in items.items():
receipt += f"{item}: \t¥{price}\n"
receipt += "===================\n"
receipt += f"总计: \t¥{total}\n"
return receipt
items = {'苹果': 5, '香蕉': 3, '牛奶': 10}
total = sum(items.values())
print(format_receipt(items, total))
二、使用库生成格式化文本
-
prettytable库
如果需要更美观的文本格式化,您可以使用第三方库,如
prettytable
。pip install prettytable
from prettytable import PrettyTable
def pretty_table_receipt(items):
table = PrettyTable()
table.field_names = ["商品", "价格"]
for item, price in items.items():
table.add_row([item, price])
return table
items = {'苹果': 5, '香蕉': 3, '牛奶': 10}
print(pretty_table_receipt(items))
三、生成PDF或图像格式的小票
-
fpdf库生成PDF
使用
fpdf
库可以生成PDF格式的小票,适合需要保存或分享的场景。pip install fpdf
from fpdf import FPDF
def pdf_receipt(filename, items, total):
pdf = FPDF()
pdf.add_page()
pdf.set_font("Arial", size=12)
pdf.cell(200, 10, txt="购物小票", ln=True, align='C')
for item, price in items.items():
pdf.cell(200, 10, txt=f"{item}: ¥{price}", ln=True, align='L')
pdf.cell(200, 10, txt=f"总计: ¥{total}", ln=True, align='L')
pdf.output(filename)
items = {'苹果': 5, '香蕉': 3, '牛奶': 10}
total = sum(items.values())
pdf_receipt("receipt.pdf", items, total)
-
PIL库生成图像
生成图像格式的小票可以用于电子收据或其他需要图形化的场景。
pip install pillow
from PIL import Image, ImageDraw, ImageFont
def image_receipt(filename, items, total):
width, height = 300, 200
image = Image.new('RGB', (width, height), color = (255, 255, 255))
draw = ImageDraw.Draw(image)
font = ImageFont.load_default()
y_text = 20
draw.text((10, y_text), "购物小票", font=font, fill=(0, 0, 0))
y_text += 20
for item, price in items.items():
draw.text((10, y_text), f"{item}: ¥{price}", font=font, fill=(0, 0, 0))
y_text += 20
draw.text((10, y_text), f"总计: ¥{total}", font=font, fill=(0, 0, 0))
image.save(filename)
items = {'苹果': 5, '香蕉': 3, '牛奶': 10}
total = sum(items.values())
image_receipt("receipt.png", items, total)
四、连接打印机打印
-
直接打印到打印机
在Windows上,您可以使用
win32print
库直接将文本输出到打印机。pip install pywin32
import win32print
import win32ui
def send_to_printer(text):
printer_name = win32print.GetDefaultPrinter()
hprinter = win32print.OpenPrinter(printer_name)
hjob = win32print.StartDocPrinter(hprinter, 1, ("Receipt", None, "RAW"))
win32print.StartPagePrinter(hprinter)
win32print.WritePrinter(hprinter, text.encode())
win32print.EndPagePrinter(hprinter)
win32print.EndDocPrinter(hprinter)
win32print.ClosePrinter(hprinter)
items = {'苹果': 5, '香蕉': 3, '牛奶': 10}
total = sum(items.values())
receipt_text = format_receipt(items, total)
send_to_printer(receipt_text)
-
使用CUPS在Linux上打印
在Linux上,CUPS(Common UNIX Printing System)是一个常用的打印系统。可以通过命令行工具或Python库与之交互。
lp -d printer_name file_to_print
或者使用Python的
os
库调用:import os
def print_with_cups(filename):
os.system(f"lp {filename}")
items = {'苹果': 5, '香蕉': 3, '牛奶': 10}
total = sum(items.values())
pdf_receipt("receipt.pdf", items, total)
print_with_cups("receipt.pdf")
通过以上这些方法,您可以选择适合自己的方式生成和打印购物小票。这些方法各有优劣,具体选择哪种方式可以根据实际需求和环境来决定。
相关问答FAQs:
如何使用Python生成购物小票的格式?
要生成购物小票的格式,可以使用Python的字符串格式化功能。你可以创建一个模板,包含商品名称、数量、单价和总价等信息。使用f-string
或str.format()
方法,将具体数据插入到模板中,确保小票的可读性和整齐性。
在Python中如何打印购物小票到打印机?
要将购物小票直接打印到打印机,可以使用win32print
库(Windows系统)或cups
库(Linux系统)。你需要设置打印机的连接,创建一个字符串或PDF文件,然后调用相应的打印命令来输出小票。确保在打印前预览小票以检查格式。
可以使用哪些Python库来美化购物小票的输出?
美化购物小票的输出可以使用Pillow
库来处理图像,或者使用reportlab
库生成PDF格式的小票。此外,prettytable
库也能帮助你创建美观的表格格式。通过这些库,可以添加边框、背景颜色、字体样式等,使小票看起来更加专业。
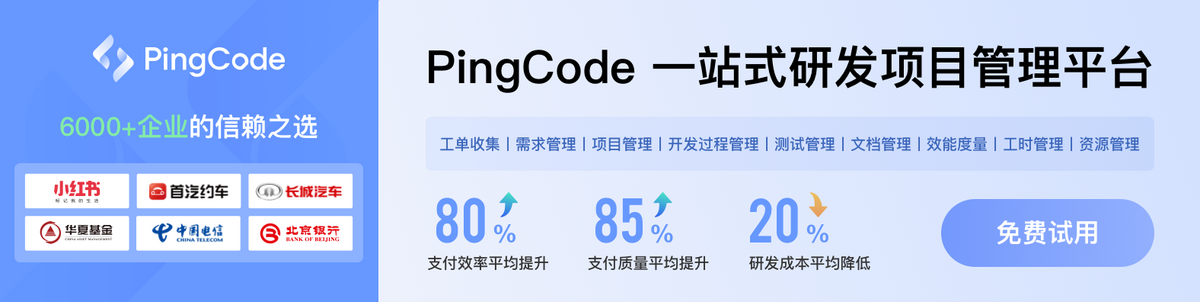