一、Python调用API接口的基本方法
Python调用API接口的方法有:使用requests库、使用http.client库、使用urllib库。其中,最常用和方便的是使用requests库,因为它提供了简洁易用的HTTP请求功能。使用requests库调用API接口的步骤主要包括:导入requests库、构造请求URL、发送请求、处理响应。通过requests库,你可以轻松处理GET、POST等多种HTTP请求方法,并解析JSON格式的响应数据。
下面我将详细展开如何使用requests库调用API接口。
二、使用requests库
requests库是Python中最受欢迎的HTTP请求库,它简化了HTTP请求的处理过程,支持各种HTTP请求方法,如GET、POST、PUT、DELETE等。
- 安装requests库
在使用requests库之前,需要确保它已经安装在你的Python环境中。可以通过以下命令安装:
pip install requests
- 发送GET请求
GET请求用于从服务器获取数据。以下是一个简单的GET请求示例:
import requests
url = 'https://api.example.com/data'
response = requests.get(url)
检查请求是否成功
if response.status_code == 200:
data = response.json() # 将响应数据解析为JSON格式
print(data)
else:
print(f"请求失败,状态码:{response.status_code}")
在这个示例中,我们首先导入了requests库,然后使用requests.get()
方法发送GET请求,并通过response.json()
方法解析响应数据为JSON格式。
- 发送POST请求
POST请求用于向服务器发送数据,通常用于提交表单数据或上传文件。以下是一个简单的POST请求示例:
import requests
url = 'https://api.example.com/update'
data = {'key1': 'value1', 'key2': 'value2'}
response = requests.post(url, data=data)
if response.status_code == 200:
print("数据提交成功")
else:
print(f"提交失败,状态码:{response.status_code}")
在这个示例中,我们使用requests.post()
方法发送POST请求,并通过data
参数传递要发送的数据。
- 处理请求头
有时,我们需要在请求中添加自定义的HTTP头,例如授权信息、用户代理等。可以通过headers
参数实现:
import requests
url = 'https://api.example.com/data'
headers = {'Authorization': 'Bearer YOUR_ACCESS_TOKEN'}
response = requests.get(url, headers=headers)
if response.status_code == 200:
data = response.json()
print(data)
else:
print(f"请求失败,状态码:{response.status_code}")
在这个示例中,我们通过headers
参数添加了一个授权头。
三、使用http.client库
http.client是Python标准库的一部分,提供了处理HTTP协议的功能。虽然不如requests库简洁,但在某些情况下它也是一个不错的选择。
- 发送GET请求
import http.client
import json
conn = http.client.HTTPSConnection("api.example.com")
conn.request("GET", "/data")
response = conn.getresponse()
if response.status == 200:
data = response.read().decode('utf-8')
json_data = json.loads(data)
print(json_data)
else:
print(f"请求失败,状态码:{response.status}")
conn.close()
在这个示例中,我们使用http.client.HTTPSConnection
创建了一个HTTPS连接,使用request()
方法发送请求,并通过getresponse()
方法获取响应。
- 发送POST请求
import http.client
import json
conn = http.client.HTTPSConnection("api.example.com")
headers = {'Content-type': 'application/json'}
payload = json.dumps({'key1': 'value1', 'key2': 'value2'})
conn.request("POST", "/update", body=payload, headers=headers)
response = conn.getresponse()
if response.status == 200:
print("数据提交成功")
else:
print(f"提交失败,状态码:{response.status}")
conn.close()
在这个示例中,我们通过headers
参数指定了请求头,并使用body
参数传递了JSON格式的请求体。
四、使用urllib库
urllib库也是Python标准库的一部分,提供了处理URL和HTTP请求的功能。
- 发送GET请求
import urllib.request
import json
url = 'https://api.example.com/data'
with urllib.request.urlopen(url) as response:
if response.status == 200:
data = response.read().decode('utf-8')
json_data = json.loads(data)
print(json_data)
else:
print(f"请求失败,状态码:{response.status}")
在这个示例中,我们使用urllib.request.urlopen()
方法打开URL并获取响应。
- 发送POST请求
import urllib.request
import json
url = 'https://api.example.com/update'
headers = {'Content-Type': 'application/json'}
data = json.dumps({'key1': 'value1', 'key2': 'value2'}).encode('utf-8')
req = urllib.request.Request(url, data=data, headers=headers, method='POST')
with urllib.request.urlopen(req) as response:
if response.status == 200:
print("数据提交成功")
else:
print(f"提交失败,状态码:{response.status}")
在这个示例中,我们使用urllib.request.Request
对象构造了POST请求,并通过urlopen()
方法发送请求。
五、处理API响应
在调用API接口时,处理响应是一个关键步骤,通常需要解析响应数据、检查状态码以及处理错误。
- 解析JSON响应
大多数API接口返回的数据都是JSON格式,可以使用json
库进行解析:
import requests
import json
response = requests.get('https://api.example.com/data')
if response.status_code == 200:
data = response.json() # 解析JSON响应
print(data)
else:
print(f"请求失败,状态码:{response.status_code}")
- 检查状态码
状态码可以帮助我们判断请求是否成功,常见的状态码有:
- 200:请求成功
- 400:请求错误
- 401:未授权
- 404:未找到
- 500:服务器错误
可以通过response.status_code
获取状态码:
if response.status_code == 200:
# 请求成功
pass
else:
# 处理错误
print(f"请求失败,状态码:{response.status_code}")
- 处理错误
在处理API响应时,可能会遇到各种错误,例如网络错误、超时错误等。可以使用异常处理机制捕获这些错误:
import requests
try:
response = requests.get('https://api.example.com/data')
response.raise_for_status() # 检查请求是否成功
data = response.json()
print(data)
except requests.exceptions.HTTPError as errh:
print(f"Http Error: {errh}")
except requests.exceptions.ConnectionError as errc:
print(f"Error Connecting: {errc}")
except requests.exceptions.Timeout as errt:
print(f"Timeout Error: {errt}")
except requests.exceptions.RequestException as err:
print(f"OOps: Something Else {err}")
通过以上步骤,你应该能够熟练地使用Python调用API接口,并处理各种请求和响应。希望这篇文章对你有所帮助。
相关问答FAQs:
如何使用Python发送HTTP请求来调用API接口?
在Python中,调用API接口通常通过发送HTTP请求来实现。最常用的库是requests
,它提供了简单易用的接口。你可以通过安装requests
库(使用命令pip install requests
),然后使用requests.get()
或requests.post()
等方法来发送请求。例如:
import requests
response = requests.get('https://api.example.com/data')
print(response.json())
这样就能获取API返回的数据。
API接口调用时需要注意哪些事项?
在调用API接口时,应该注意几个关键因素。首先,确保你了解API的文档,包括请求的方法(GET、POST等)、必需的参数、请求头以及返回的数据格式。其次,处理好错误和异常情况,例如网络问题或API返回错误代码,确保你的代码具有良好的容错性。此外,遵循API的使用限制,避免过于频繁的请求。
如何在Python中处理API返回的JSON数据?
许多API会返回JSON格式的数据,Python的requests
库可以非常方便地将其解析为字典对象。使用response.json()
方法可以直接将返回的JSON数据转换为Python对象。以下是一个示例:
import requests
response = requests.get('https://api.example.com/data')
data = response.json() # 将JSON数据转换为字典
print(data['key']) # 访问特定数据
这种方式使得处理和提取数据变得更加简单和高效。
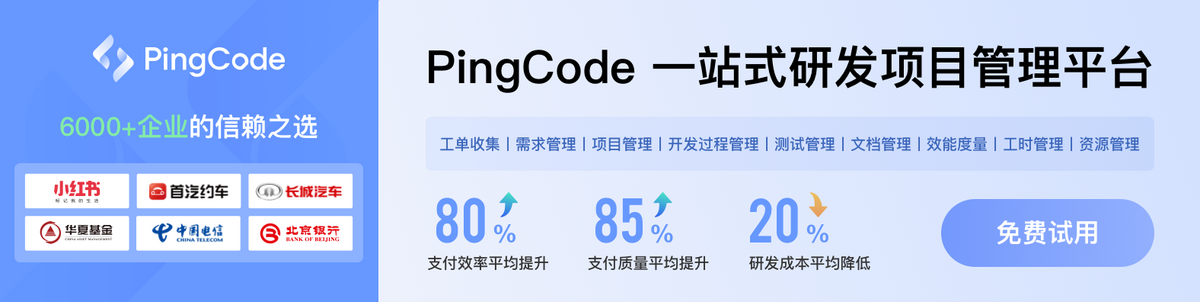