在Python中,去掉字符串中的特定字符、空白、或者其他内容是一个常见的操作。使用字符串的内置方法、正则表达式、列表解析都是实现这一需求的有效途径。接下来,我将详细描述使用这些方法的步骤和注意事项。
一、使用字符串内置方法
Python提供了一些内置方法,可以方便地操作字符串。这些方法包括strip()
、lstrip()
、rstrip()
、replace()
等。
1. 使用strip()
方法
strip()
用于去除字符串开头和结尾的空白字符。它也可以去除指定字符。
text = " hello world "
cleaned_text = text.strip()
输出: "hello world"
如果需要去掉特定字符,可以传入参数:
text = "xxhelloxyworldxx"
cleaned_text = text.strip('x')
输出: "helloxyworld"
2. 使用replace()
方法
replace()
可以用来替换字符串中的某些部分。
text = "hello world"
cleaned_text = text.replace('l', '')
输出: "heo word"
二、使用正则表达式
正则表达式是处理字符串的强大工具,Python的re
模块提供了丰富的正则表达式功能。
1. 基本用法
首先,需要导入re
模块。
import re
text = "hello 123 world"
cleaned_text = re.sub(r'\d+', '', text)
输出: "hello world"
以上代码去除了字符串中的数字。
2. 删除特定字符
text = "hello 123 world"
cleaned_text = re.sub(r'[0-9]', '', text)
输出: "hello world"
三、使用列表解析
列表解析结合join()
方法,可以高效地去除字符串中的特定字符。
1. 基本用法
text = "hello world"
cleaned_text = ''.join([char for char in text if char != 'l'])
输出: "heo word"
2. 去除多个字符
text = "hello world"
cleaned_text = ''.join([char for char in text if char not in ['l', 'o']])
输出: "he wrd"
四、应用场景和注意事项
1. 去除空白字符
在处理用户输入或解析文件数据时,去除多余的空白字符是很重要的。
text = " hello world "
cleaned_text = ' '.join(text.split())
输出: "hello world"
2. 性能考量
对于处理大型文本或频繁执行的操作,选择高效的方法尤为重要。正则表达式虽然强大,但在某些情况下性能较差。对于简单的替换或去除操作,replace()
或列表解析可能更为高效。
3. 处理非ASCII字符
在处理包含非ASCII字符的字符串时,要特别注意字符编码问题。Python 3 默认使用Unicode字符串,因此通常不需要额外处理。但在与外部系统交互时,可能需要注意编码转换。
五、总结
在Python中去除字符串中的特定字符或内容,可以通过多种方法实现。根据具体需求选择合适的工具,字符串内置方法适用于简单替换,正则表达式适合复杂匹配,列表解析则提供了一种灵活的方案。理解每种方法的适用场景和性能特点,将帮助你更高效地解决实际问题。
相关问答FAQs:
如何在Python中去除字符串中的空格?
在Python中,可以使用str.replace()
方法或者str.split()
和str.join()
组合来去除字符串中的空格。使用replace()
可以直接替换空格字符,而使用split()
和join()
方法可以去除所有空格,示例如下:
# 使用replace去除空格
string_with_spaces = "Hello World"
no_spaces = string_with_spaces.replace(" ", "")
print(no_spaces) # 输出: HelloWorld
# 使用split和join去除所有空格
string_with_spaces = " Hello World "
no_spaces = ''.join(string_with_spaces.split())
print(no_spaces) # 输出: HelloWorld
Python中是否有内置函数可以去除字符串的特定字符?
是的,Python中的str.strip()
、str.lstrip()
和str.rstrip()
方法可以用来去除字符串两端的特定字符。strip()
去除两端的字符,lstrip()
仅去除左侧字符,而rstrip()
则去除右侧字符。以下是相关示例:
example = "###Hello World###"
cleaned = example.strip("#")
print(cleaned) # 输出: Hello World
在Python中如何处理去除空格后的字符串呢?
去除空格后的字符串可以用于进一步的数据处理和分析。可以使用字符串的各种方法进行分割、查找或替换操作。比如,可以使用str.split()
方法将去除空格的字符串分割成单词列表,从而便于后续处理:
string_with_spaces = "Python is fun"
no_spaces = ''.join(string_with_spaces.split())
word_list = no_spaces.split()
print(word_list) # 输出: ['Python', 'is', 'fun']
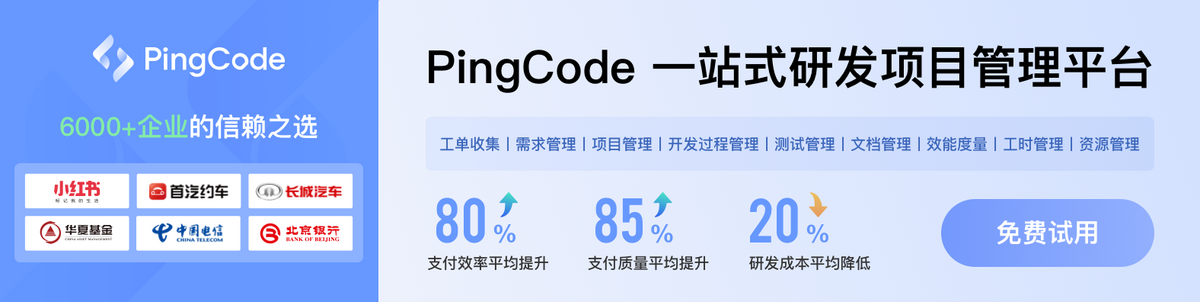