在Python中表示时间的方式有多种,常用的库包括datetime
、time
和calendar
。每个库都有其特定的用途,例如,datetime
提供了一个更高级别和更直观的方法来处理日期和时间,而time
则更侧重于处理和格式化时间戳。对于更复杂的日期操作,calendar
可以帮助生成日历和处理日期范围。下面将详细介绍datetime
模块的用法。
datetime
模块是Python中处理日期和时间的标准库。它提供了日期(date)、时间(time)和日期时间(datetime)三种基本类型。datetime
模块允许我们进行日期和时间的算术运算,格式化显示等。以下是使用datetime
模块表示和操作时间的几个关键步骤:
一、DATETIME模块的基础
Python的datetime
模块是处理日期和时间的强大工具。在使用该模块之前,需要进行一些基本的了解。
1、date
对象
date
对象表示一个日期(年、月、日)。可以通过datetime.date(year, month, day)
构造一个日期对象。例如:
from datetime import date
today = date.today()
print("Today's date:", today)
2、time
对象
time
对象表示一个时间(时、分、秒、微秒)。可以通过datetime.time(hour, minute, second, microsecond)
构造一个时间对象。例如:
from datetime import time
current_time = time(14, 30, 0)
print("Current time:", current_time)
3、datetime
对象
datetime
对象表示一个日期和时间的组合。可以通过datetime.datetime(year, month, day, hour, minute, second, microsecond)
构造。例如:
from datetime import datetime
now = datetime.now()
print("Current date and time:", now)
二、时间格式化与解析
处理时间时,格式化和解析是非常重要的功能。datetime
模块提供了多种方法来格式化和解析日期时间。
1、格式化日期时间
使用strftime
方法可以格式化日期时间,将日期时间对象转换为字符串。例如:
formatted_date = now.strftime("%Y-%m-%d %H:%M:%S")
print("Formatted date and time:", formatted_date)
2、解析日期时间字符串
使用strptime
方法可以解析日期时间字符串,将字符串转换为日期时间对象。例如:
date_string = "2023-10-15 14:30:00"
parsed_date = datetime.strptime(date_string, "%Y-%m-%d %H:%M:%S")
print("Parsed date and time:", parsed_date)
三、时间的算术运算
datetime
模块不仅可以表示时间,还可以进行时间的算术运算,比如日期的加减。
1、时间差计算
可以使用timedelta
对象计算时间差。例如,计算两个日期之间的差值:
from datetime import timedelta
yesterday = today - timedelta(days=1)
print("Yesterday's date:", yesterday)
2、增加或减少时间
可以通过timedelta
对象增加或减少时间。例如,增加一小时:
one_hour_later = now + timedelta(hours=1)
print("One hour later:", one_hour_later)
四、TIME模块的使用
time
模块提供了对时间戳的处理功能,时间戳是从1970年1月1日UTC开始经过的秒数。
1、获取当前时间戳
可以使用time.time()
获取当前的时间戳:
import time
current_timestamp = time.time()
print("Current timestamp:", current_timestamp)
2、将时间戳转换为日期时间
使用time.localtime()
或time.gmtime()
可以将时间戳转换为结构化时间对象,然后用time.strftime()
进行格式化:
local_time = time.localtime(current_timestamp)
formatted_local_time = time.strftime("%Y-%m-%d %H:%M:%S", local_time)
print("Formatted local time:", formatted_local_time)
五、CALENDAR模块的使用
calendar
模块提供了对日期进行高级操作的功能,如生成日历、判断闰年等。
1、生成日历
可以使用calendar.month()
生成一个月的日历:
import calendar
year = 2023
month = 10
month_calendar = calendar.month(year, month)
print("October 2023 calendar:\n", month_calendar)
2、判断闰年
使用calendar.isleap()
可以判断某一年是否为闰年:
is_leap_year = calendar.isleap(2024)
print("Is 2024 a leap year?", is_leap_year)
六、总结
在Python中表示和操作时间的方式多种多样,主要集中在datetime
、time
和calendar
三个模块。datetime
模块是最常用的,因为它提供了强大的日期和时间操作功能,包括格式化、解析和算术运算。time
模块主要用于处理时间戳,而calendar
模块可以帮助生成日历和判断闰年等。通过合理选择和组合这些模块的功能,可以满足大多数时间处理需求。
相关问答FAQs:
在Python中有哪些常用的时间表示方法?
Python提供了多种方式来表示时间,最常用的包括datetime
模块、time
模块和calendar
模块。datetime
模块提供了datetime
、date
、time
和timedelta
等类,可以用来表示和操作日期和时间。使用time
模块可以获取当前时间的时间戳,而calendar
模块则用于处理与日历相关的功能。
如何在Python中获取当前时间和日期?
使用datetime
模块中的datetime.now()
方法可以轻松获取当前的日期和时间。例如,通过from datetime import datetime
导入后,调用datetime.now()
即可返回一个datetime
对象,代表当前时刻。对于只需要当前日期或时间的情况,可以使用datetime.today()
或者datetime.now().date()
和datetime.now().time()
方法。
如何将字符串转换为时间对象?
在Python中,可以使用datetime.strptime()
方法将字符串转换为时间对象。这个方法需要两个参数:待转换的字符串和格式字符串。格式字符串定义了输入字符串的日期时间格式,例如,"%Y-%m-%d %H:%M:%S"
表示“年-月-日 时:分:秒”的格式。这样,用户可以灵活地解析不同格式的日期和时间字符串。
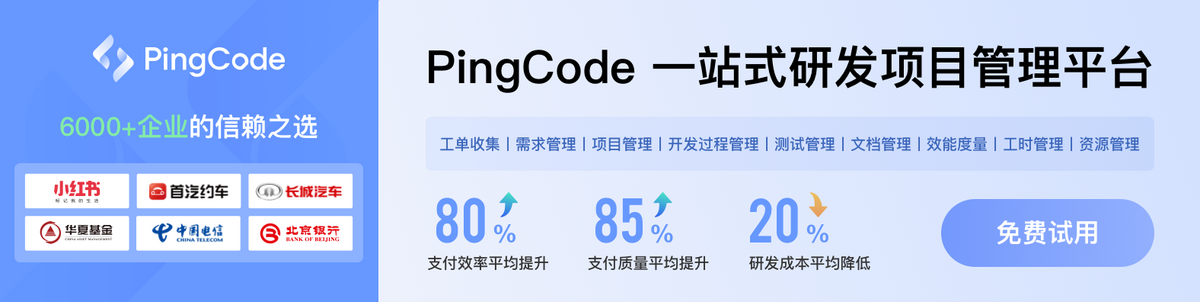