在Python中显示购物菜单的方法包括:使用列表或字典存储商品信息、通过循环输出菜单、提供用户友好的界面。使用字典存储商品信息可以让数据更有结构化,更方便后续的查询和更新。接下来,我们将详细探讨如何使用Python创建和显示购物菜单。
一、使用列表和字典存储商品信息
在创建购物菜单时,首先需要存储商品的信息。常用的数据结构是列表和字典。使用字典可以更直观地表示商品的属性。
# 使用字典存储商品信息
products = {
1: {"name": "苹果", "price": 3.5},
2: {"name": "香蕉", "price": 2.5},
3: {"name": "橘子", "price": 4.0}
}
在这个例子中,我们使用一个字典products
,键是商品的编号,值是另一个字典,其中包含商品的名称和价格。这种结构便于后续的操作,比如查询、增加或删除商品。
二、通过循环输出菜单
为了让用户看到购物菜单,我们需要遍历商品信息,并以友好的格式输出。
# 输出购物菜单
def display_menu(products):
print("购物菜单:")
for product_id, product_info in products.items():
print(f"{product_id}. {product_info['name']} - ${product_info['price']}")
display_menu(products)
这个函数display_menu
使用了一个for循环来遍历字典中的商品,并输出每个商品的编号、名称和价格。这种方式简单明了,用户可以快速浏览可购买的商品。
三、提供用户友好的界面
在购物系统中,用户界面是非常重要的一部分。我们可以通过一些简单的交互提升用户体验。
- 输入提示:提示用户输入以选择商品。
def get_user_choice():
try:
choice = int(input("请输入商品编号以选择商品(或输入0退出):"))
return choice
except ValueError:
print("无效输入,请输入数字。")
return get_user_choice()
choice = get_user_choice()
在这个函数中,我们使用input
获取用户的输入,并通过try-except
结构处理可能的输入错误。
- 处理用户选择:根据用户输入的编号,执行相应的操作。
def handle_choice(choice, products):
if choice == 0:
print("退出购物。")
elif choice in products:
product = products[choice]
print(f"您选择了 {product['name']},价格为 ${product['price']}")
else:
print("无效的商品编号。")
handle_choice(choice, products)
这个函数根据用户输入的编号,输出相应的商品信息。如果输入的编号无效,给予友好的提示。
四、综合示例
结合以上步骤,我们可以编写一个完整的示例,展示如何在Python中实现一个简单的购物菜单系统。
def display_menu(products):
print("购物菜单:")
for product_id, product_info in products.items():
print(f"{product_id}. {product_info['name']} - ${product_info['price']}")
def get_user_choice():
try:
choice = int(input("请输入商品编号以选择商品(或输入0退出):"))
return choice
except ValueError:
print("无效输入,请输入数字。")
return get_user_choice()
def handle_choice(choice, products):
if choice == 0:
print("退出购物。")
elif choice in products:
product = products[choice]
print(f"您选择了 {product['name']},价格为 ${product['price']}")
else:
print("无效的商品编号。")
def main():
products = {
1: {"name": "苹果", "price": 3.5},
2: {"name": "香蕉", "price": 2.5},
3: {"name": "橘子", "price": 4.0}
}
while True:
display_menu(products)
choice = get_user_choice()
if choice == 0:
break
handle_choice(choice, products)
if __name__ == "__main__":
main()
这个示例展示了一个完整的购物菜单系统,用户可以查看菜单、选择商品并获取反馈。通过这种方式,我们可以轻松实现一个简单的购物菜单界面。
相关问答FAQs:
如何在Python中创建一个简单的购物菜单?
要在Python中创建一个简单的购物菜单,可以使用字典来存储商品及其价格,并通过循环让用户选择商品。以下是一个基本的示例代码:
menu = {
"苹果": 3.0,
"香蕉": 2.0,
"橙子": 4.0
}
print("欢迎来到购物菜单!")
for item, price in menu.items():
print(f"{item}: ${price}")
选择 = input("请选择您想购买的商品:")
if 选择 in menu:
print(f"您选择的商品是:{选择},价格为:${menu[选择]}")
else:
print("该商品不在菜单中。")
如何在购物菜单中添加更多功能,如数量选择和总价计算?
可以通过增加一个输入步骤来让用户选择购买的数量,并计算总价。以下是修改后的代码示例:
数量 = int(input("请输入购买数量:"))
总价 = menu[选择] * 数量
print(f"您选择的商品是:{选择},数量为:{数量},总价为:${总价}")
通过这样的方式,用户可以更好地体验购物过程。
在Python中如何美化购物菜单的展示效果?
美化购物菜单的展示可以通过使用格式化字符串和其他库来实现。可以考虑使用prettytable
库来生成更整齐的表格,或者使用ANSI转义序列来增加颜色。以下是使用prettytable
的示例:
from prettytable import PrettyTable
table = PrettyTable()
table.field_names = ["商品", "价格"]
for item, price in menu.items():
table.add_row([item, price])
print(table)
通过这种方式,购物菜单会更具吸引力,提升用户体验。
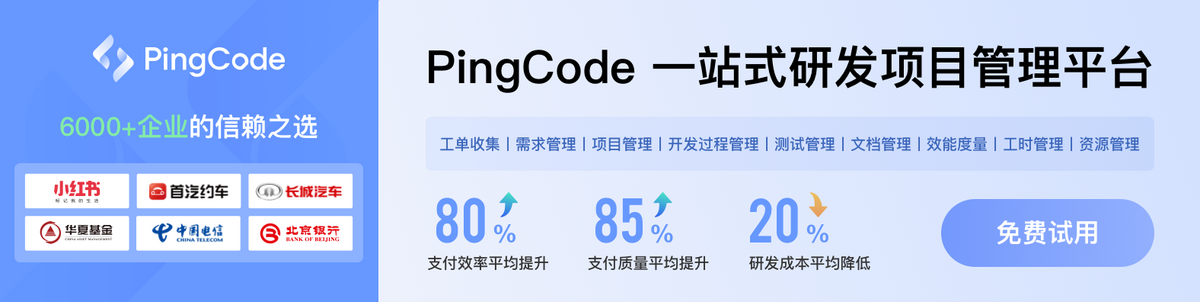