在Python中读取txt文件的方法有很多种,使用内置函数open()、利用with语句、使用read()、readline()、readlines()方法来读取文件内容。其中,使用with
语句和open()
函数是最常见且推荐的方法,因为它能自动处理文件的关闭操作,避免内存泄漏。下面我将详细介绍这些方法及其应用。
一、使用open()函数
open()
是Python内置的函数,用于打开一个文件并返回文件对象。基本用法是open(filename, mode)
,其中filename
是要打开的文件名,mode
是打开文件的模式,如读模式('r')、写模式('w')等。
1. 基本用法
file = open('example.txt', 'r')
content = file.read()
print(content)
file.close()
这种方法需要手动关闭文件,通过调用file.close()
,以确保资源的释放。
2. 使用with语句
with
语句可以自动管理文件的打开和关闭,非常推荐使用这种方式。
with open('example.txt', 'r') as file:
content = file.read()
print(content)
在with
块内,文件是打开的,离开块之后,文件会被自动关闭。
二、使用read()、readline()、readlines()方法
1. read()方法
read()
方法读取文件的所有内容,并将其作为字符串返回。
with open('example.txt', 'r') as file:
content = file.read()
print(content)
2. readline()方法
readline()
方法每次读取一行,返回一个字符串。
with open('example.txt', 'r') as file:
line = file.readline()
while line:
print(line, end='')
line = file.readline()
3. readlines()方法
readlines()
方法读取文件的所有行,并将其作为一个列表返回,每个元素是一行。
with open('example.txt', 'r') as file:
lines = file.readlines()
for line in lines:
print(line, end='')
三、逐行读取大文件
对于大文件,一次性读取所有内容会消耗大量内存,可以选择逐行读取。
with open('largefile.txt', 'r') as file:
for line in file:
print(line, end='')
这种方法使用了文件对象的迭代器特性,内存效率更高。
四、读取不同编码的文件
有些文件使用不同的字符编码,可以在open()
函数中指定编码。
with open('example.txt', 'r', encoding='utf-8') as file:
content = file.read()
print(content)
五、读取二进制文件
读取二进制文件需要以二进制模式打开文件。
with open('example.bin', 'rb') as file:
content = file.read()
print(content)
六、处理文件异常
在文件操作时,要处理可能的异常,如文件不存在、权限不足等。
try:
with open('example.txt', 'r') as file:
content = file.read()
print(content)
except FileNotFoundError:
print("The file does not exist")
except IOError:
print("An I/O error occurred")
七、总结
在Python中读取txt文件的方法多种多样,最常用的是使用with
语句和open()
函数结合的方式,可以有效地管理文件资源,避免内存泄漏。根据实际需要,可以选择读取文件的所有内容、逐行读取或读取成列表。处理大文件时,逐行读取的方式可以节省内存。还可以通过指定编码来读取不同编码的文件,确保读取内容的正确性。在文件读取操作中,适当的异常处理是必要的,以应对各种可能的错误情况。
相关问答FAQs:
如何在Python中读取文本文件的内容?
在Python中,可以使用内置的open()
函数来读取文本文件。打开文件后,可以使用read()
, readline()
, 或 readlines()
方法来读取文件内容。示例代码如下:
with open('filename.txt', 'r') as file:
content = file.read()
print(content)
这种方式可以确保文件在使用后自动关闭,避免资源泄露。
读取文本文件时有什么常见的错误需要注意?
在读取文本文件时,常见的错误包括文件未找到(FileNotFoundError)和权限错误(PermissionError)。确保文件路径正确且具有读取权限。如果文件路径包含特殊字符或空格,建议使用原始字符串(在路径前加r
)或正确转义字符。
如何读取大文件而不占用过多内存?
对于较大的文本文件,使用read()
一次性读取整个文件可能会导致内存不足。此时,可以逐行读取文件,使用for
循环或readline()
方法逐行处理内容。例如:
with open('largefile.txt', 'r') as file:
for line in file:
print(line.strip())
这种方法可以有效减少内存占用,适合处理大量数据的情况。
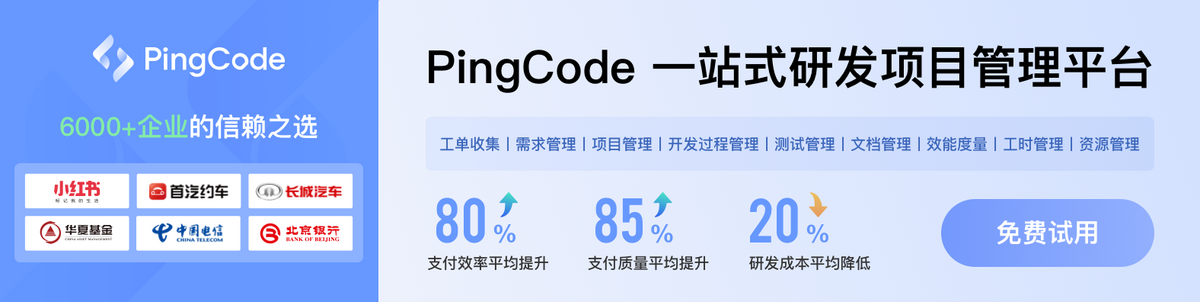