在Python中删除图片的方式有多种,其中常用的方法包括使用os模块、使用shutil模块、使用pathlib模块。接下来,我们将详细介绍这几种方法,并讨论它们的应用场景。
一、使用OS模块
os模块是Python标准库中的一个模块,提供了与操作系统进行交互的功能。删除文件是其中的一项基本功能。
import os
def delete_image_with_os(image_path):
try:
os.remove(image_path)
print(f"Image {image_path} successfully deleted.")
except FileNotFoundError:
print(f"Image {image_path} not found.")
except PermissionError:
print(f"Permission denied to delete {image_path}.")
except Exception as e:
print(f"An error occurred: {e}")
Example usage:
delete_image_with_os('path/to/your/image.jpg')
使用os模块删除图片的优点是它非常简单直接,适合处理单个文件的删除操作。然而,它的局限性在于对于需要更多文件操作功能的场景(如批量删除、文件移动等),可能需要结合其他模块使用。
二、使用SHUTIL模块
shutil模块提供了对文件和文件集合的高层次操作功能。虽然它主要用于复制和移动文件,但也可以用于删除文件。
import shutil
def delete_image_with_shutil(image_path):
try:
shutil.os.remove(image_path)
print(f"Image {image_path} successfully deleted.")
except FileNotFoundError:
print(f"Image {image_path} not found.")
except PermissionError:
print(f"Permission denied to delete {image_path}.")
except Exception as e:
print(f"An error occurred: {e}")
Example usage:
delete_image_with_shutil('path/to/your/image.jpg')
shutil模块的优势是当你需要进行更复杂的文件操作(如复制和移动)时,它可以与删除操作结合使用。然而,它的缺点是对于单纯的删除操作,这个模块可能显得有些多余。
三、使用PATHLIB模块
pathlib模块是Python 3.4引入的一个模块,提供了一种面向对象的方式来处理文件和目录。它不仅可以用于删除文件,还可以用于其他文件操作。
from pathlib import Path
def delete_image_with_pathlib(image_path):
file_path = Path(image_path)
try:
file_path.unlink()
print(f"Image {image_path} successfully deleted.")
except FileNotFoundError:
print(f"Image {image_path} not found.")
except PermissionError:
print(f"Permission denied to delete {image_path}.")
except Exception as e:
print(f"An error occurred: {e}")
Example usage:
delete_image_with_pathlib('path/to/your/image.jpg')
pathlib模块的优点在于其面向对象的设计,使得文件操作更加直观和易于阅读。此外,它还提供了强大的路径管理功能,这对于需要处理复杂路径操作的项目来说非常有用。
四、批量删除图片
在一些应用场景中,可能需要删除目录下的所有图片或特定类型的图片。可以结合os模块或pathlib模块实现批量删除。
使用os模块批量删除
import os
def delete_images_in_directory_with_os(directory_path, extension=".jpg"):
try:
for filename in os.listdir(directory_path):
if filename.endswith(extension):
os.remove(os.path.join(directory_path, filename))
print(f"Deleted: {filename}")
except Exception as e:
print(f"An error occurred: {e}")
Example usage:
delete_images_in_directory_with_os('path/to/your/directory', '.jpg')
使用pathlib模块批量删除
from pathlib import Path
def delete_images_in_directory_with_pathlib(directory_path, extension=".jpg"):
directory = Path(directory_path)
try:
for file_path in directory.glob(f'*{extension}'):
file_path.unlink()
print(f"Deleted: {file_path.name}")
except Exception as e:
print(f"An error occurred: {e}")
Example usage:
delete_images_in_directory_with_pathlib('path/to/your/directory', '.jpg')
批量删除的优势在于可以极大地提高效率,尤其是在处理大量图片时。然而,需要注意的是,批量删除操作风险较高,建议在执行前备份重要文件。
五、删除图片的安全性考虑
在删除文件时,安全性是一个重要的考虑因素。误删文件可能造成数据丢失,因此在执行删除操作时需特别小心。
- 确认路径:在删除文件之前,确保文件路径正确,以避免误删其他重要文件。
- 权限管理:确保程序具有足够的权限删除目标文件,避免因权限不足导致删除失败。
- 数据备份:在执行批量删除操作之前,建议备份重要数据,以防止误操作带来的数据丢失。
通过以上介绍,我们可以看到,在Python中删除图片有多种途径,每种方法都有其适用的场景和优缺点。选择合适的方法,不仅可以提高开发效率,还能更好地管理文件安全。
相关问答FAQs:
如何在Python中安全地删除图片文件?
在Python中,删除图片文件可以使用内置的os
模块来实现。首先,确保您已经确认要删除的文件路径是正确的。使用os.remove(file_path)
方法可以删除指定路径的文件。为了避免错误,建议在删除前检查文件是否存在,可以使用os.path.exists(file_path)
来确认。
删除图片时需要注意哪些事项?
在删除图片文件时,需要确保该文件不在其他程序中被占用。此外,请确保您拥有删除该文件的权限。为防止误删,可以考虑在删除前备份文件,或者使用shutil
模块中的shutil.move()
方法将文件移动到回收站,而不是永久删除。
如何批量删除多个图片文件?
如果需要删除多个图片文件,可以使用循环遍历文件列表,并逐一调用os.remove()
方法。在删除之前,可以使用os.listdir()
方法获取目录下所有文件名,并对文件进行筛选,确保只删除需要的图片文件。此外,使用异常处理(try-except
语句)可以处理可能出现的错误,比如文件不存在等情况,从而确保程序的稳定性。
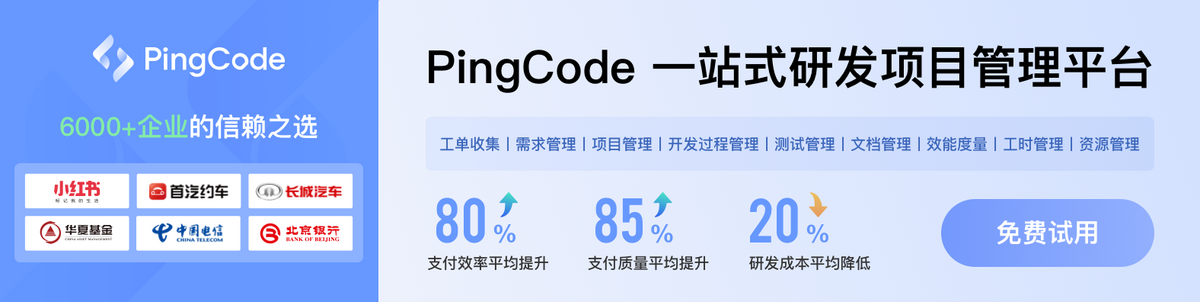