在Python中引入sin函数非常简单,主要通过使用Python的数学库——math模块来实现。要引入sin函数,你需要导入math模块、使用math.sin()函数、传递参数以计算正弦值。下面将详细描述这一过程。
首先,你需要在Python脚本中导入math模块。math模块是Python的标准库之一,因此不需要额外安装,只需在代码开头使用import math
即可。接下来,你可以使用math.sin()
来计算一个角度的正弦值。需要注意的是,math.sin()函数的参数是以弧度为单位的,而不是度数,所以在使用时可能需要将角度转换为弧度,这可以通过math.radians()
函数来实现。例如,若要计算30度的正弦值,可以先将30度转换为弧度,再传递给math.sin()
函数。
import math
将角度转换为弧度
angle_in_degrees = 30
angle_in_radians = math.radians(angle_in_degrees)
计算正弦值
sin_value = math.sin(angle_in_radians)
print(f"The sine of {angle_in_degrees} degrees is {sin_value}")
通过这种方式,你就可以在Python中引入并使用sin函数来计算三角函数的正弦值。
一、MATH模块介绍
math模块是Python中一个强大的数学库,提供了许多数学函数和常量,广泛用于科学计算、工程计算、数据分析等领域。math模块不仅包括基本的算术运算,还提供了一系列高级数学函数。除了sin函数外,math模块还包括cos、tan、asin、acos、atan等三角函数,以及其他函数如对数函数、指数函数、平方根函数等。
- 导入math模块
使用math模块中的函数之前,必须先导入该模块。这可以通过import math
来实现。导入后,就可以使用模块中的各种数学函数。
import math
- math模块中的常用函数
- math.sin(x):返回x的正弦值,x以弧度为单位。
- math.cos(x):返回x的余弦值,x以弧度为单位。
- math.tan(x):返回x的正切值,x以弧度为单位。
- math.radians(degrees):将角度转换为弧度。
- math.degrees(radians):将弧度转换为角度。
二、使用MATH.SIN函数
math.sin()函数是用于计算给定角度的正弦值的函数。角度必须以弧度为单位传递给函数。正弦函数在许多领域有着广泛的应用,比如信号处理、波动分析、振动分析等。
- 计算弧度角的正弦值
当你已经有一个弧度值时,可以直接将其传递给math.sin()函数来计算正弦值。
import math
radians = 1.0
sin_value = math.sin(radians)
print(f"The sine of {radians} radians is {sin_value}")
- 将角度转换为弧度后计算正弦值
如果你有一个角度值而不是弧度值,则需要首先使用math.radians()将其转换为弧度。
import math
degrees = 30
radians = math.radians(degrees)
sin_value = math.sin(radians)
print(f"The sine of {degrees} degrees is {sin_value}")
三、SIN函数的应用
sin函数不仅用于简单的数学计算,还在计算机图形学、信号处理、物理模拟等领域有着广泛的应用。
- 信号处理
在信号处理领域,sin函数用于生成正弦波信号。正弦波是一种基本的周期信号,广泛应用于模拟信号和数字信号处理中。
import numpy as np
import matplotlib.pyplot as plt
生成一个正弦波信号
t = np.linspace(0, 2 * np.pi, 1000)
signal = np.sin(t)
plt.plot(t, signal)
plt.title("Sine Wave")
plt.xlabel("Time")
plt.ylabel("Amplitude")
plt.grid(True)
plt.show()
- 计算机图形学
在计算机图形学中,sin函数用于生成波浪、震动等效果。例如,在动画中可以使用sin函数来模拟摆动或波动的运动效果。
import matplotlib.pyplot as plt
import numpy as np
创建一个摆动效果的动画
x = np.linspace(0, 4 * np.pi, 1000)
y = np.sin(x)
plt.plot(x, y)
plt.title("Sine Wave in Graphics")
plt.xlabel("x")
plt.ylabel("sin(x)")
plt.grid(True)
plt.show()
四、角度转换与精度处理
在使用sin函数时,角度转换和精度处理是两个需要注意的问题。
- 角度转换
当你手头的角度值是以度数为单位时,必须将其转换为弧度,因为math.sin()函数只接受弧度值。这可以通过math.radians()函数来实现。
import math
degrees = 90
radians = math.radians(degrees)
print(f"{degrees} degrees is {radians} radians")
- 精度处理
由于计算机在处理浮点数时有精度限制,因此在使用sin函数时可能会遇到精度问题。通常情况下,Python的浮点数精度已经足够满足大多数应用需求,但在极高精度计算中,可能需要额外的处理。
例如,可以使用Python的decimal模块来提高计算精度。
import math
from decimal import Decimal, getcontext
设置Decimal模块的精度
getcontext().prec = 50
使用Decimal计算sin的高精度值
angle_in_degrees = 30
angle_in_radians = Decimal(math.radians(angle_in_degrees))
sin_value = Decimal(math.sin(float(angle_in_radians)))
print(f"The high precision sine of {angle_in_degrees} degrees is {sin_value}")
五、Python中的其他三角函数
除了sin函数,Python的math模块还提供了其他常用的三角函数,这些函数同样需要弧度作为输入。
- 余弦函数(cos)
math.cos()函数用于计算一个角度的余弦值。
import math
angle_in_radians = math.pi / 3
cos_value = math.cos(angle_in_radians)
print(f"The cosine of {angle_in_radians} radians is {cos_value}")
- 正切函数(tan)
math.tan()函数用于计算一个角度的正切值。
import math
angle_in_radians = math.pi / 4
tan_value = math.tan(angle_in_radians)
print(f"The tangent of {angle_in_radians} radians is {tan_value}")
- 反正弦函数(asin)
math.asin()函数用于计算一个值的反正弦值,返回值为弧度。
import math
value = 0.5
asin_value = math.asin(value)
print(f"The arcsine of {value} is {asin_value} radians")
- 反余弦函数(acos)
math.acos()函数用于计算一个值的反余弦值,返回值为弧度。
import math
value = 0.5
acos_value = math.acos(value)
print(f"The arccosine of {value} is {acos_value} radians")
- 反正切函数(atan)
math.atan()函数用于计算一个值的反正切值,返回值为弧度。
import math
value = 1.0
atan_value = math.atan(value)
print(f"The arctangent of {value} is {atan_value} radians")
六、总结
在Python中引入和使用sin函数非常简单,只需导入math模块并使用math.sin()函数即可。需要注意的是,math.sin()函数的参数必须是弧度值,因此在处理角度时,需要使用math.radians()函数进行转换。sin函数在许多领域都有广泛应用,如信号处理和计算机图形学中。此外,Python的math模块还提供了其他常用的三角函数,可以用于更复杂的数学计算和应用。通过理解和掌握这些函数的使用,可以更好地应用Python进行科学计算和数据分析。
相关问答FAQs:
引入sin函数需要哪些步骤?
要在Python中使用sin函数,首先需要导入math模块。可以通过在代码的开始部分添加import math
来完成。这样就可以通过math.sin()
来调用sin函数。
sin函数的输入参数是什么?
sin函数接受一个浮点数作为输入,这个参数是以弧度为单位的角度值。如果你需要以角度为单位进行计算,可以使用math.radians()
函数将角度转换为弧度。
在Python中如何绘制sin函数的图形?
可以使用matplotlib库来绘制sin函数图形。首先,确保安装了matplotlib库,然后通过生成一系列弧度值,计算对应的sin值并使用plt.plot()绘制图形。示例代码如下:
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(0, 2 * np.pi, 100)
y = np.sin(x)
plt.plot(x, y)
plt.title('Sine Function')
plt.xlabel('Angle in Radians')
plt.ylabel('Sine Value')
plt.grid()
plt.show()
sin函数的常见应用有哪些?
sin函数在科学和工程领域有广泛应用,特别是在波动、振动、周期性现象等方面。它通常用于计算周期性运动的幅度和相位,或者在信号处理和音频合成中生成波形。
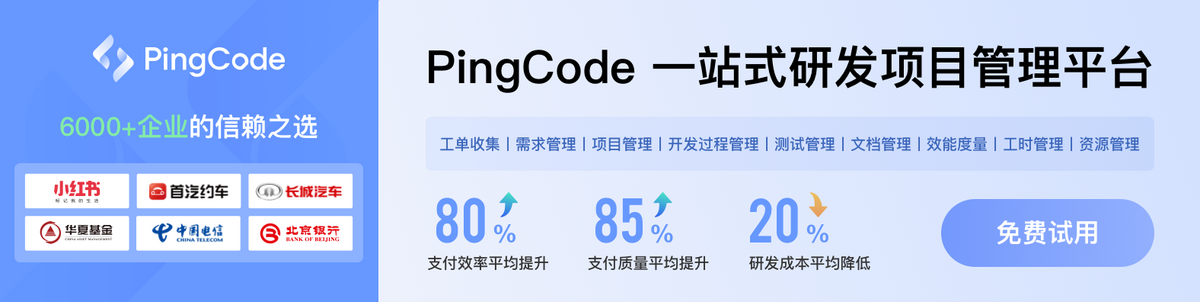