Python中可以通过使用循环、使用input()函数、结合条件判断等方法来实现连续输入指令。在Python编程中,连续输入指令通常用于需要多次读取用户输入的场景,如交互式脚本、数据录入等。其中,while循环是实现连续输入指令的常用方式,通过设置适当的退出条件,可以让程序根据用户的需求持续运行,直到满足条件时退出。接下来,我将详细展开如何在Python中实现连续输入指令的几种方法。
一、使用WHILE循环和INPUT()函数
在Python中,使用while
循环结合input()
函数是最常见的实现连续输入的方式。while
循环可以让程序在满足某个条件时持续执行,而input()
函数用于读取用户输入。通过在while
循环中调用input()
函数,可以实现连续输入指令。
- 基本实现
在最简单的情况下,可以使用一个无限循环来实现连续输入,用户通过输入特定的指令来退出循环。
while True:
command = input("Enter your command (type 'exit' to quit): ")
if command.lower() == 'exit':
print("Exiting the program.")
break
else:
print(f"Command received: {command}")
在这个例子中,程序会持续要求用户输入指令,直到用户输入'exit'
命令时程序才会退出。使用lower()
方法可以确保用户输入大小写不敏感。
- 添加输入验证
为了提高程序的鲁棒性,可以在读取用户输入后添加验证逻辑,以确保输入的有效性。这样可以避免程序因不当输入而崩溃。
while True:
command = input("Enter your command (type 'exit' to quit): ")
if not command.strip():
print("Empty input, please enter a command.")
continue
if command.lower() == 'exit':
print("Exiting the program.")
break
else:
print(f"Command received: {command}")
在这里,通过strip()
方法去除空格来检查输入是否为空,并提示用户重新输入。
二、结合条件判断实现特定功能
通过结合条件判断和循环,可以在输入不同指令时执行不同的操作。这种方法常用于需要根据用户输入执行特定任务的场景。
- 基于输入执行不同任务
def execute_command(command):
if command == 'print_hello':
print("Hello, World!")
elif command == 'show_date':
from datetime import datetime
print(f"Current date and time: {datetime.now()}")
else:
print("Unknown command.")
while True:
command = input("Enter your command (type 'exit' to quit): ")
if command.lower() == 'exit':
print("Exiting the program.")
break
else:
execute_command(command)
在这个例子中,根据用户输入的指令执行不同的任务。用户可以输入'print_hello'
来打印“Hello, World!”或者输入'show_date'
来显示当前日期和时间。
- 使用字典映射指令到函数
使用字典可以更加优雅地实现指令与功能的映射,减少大量的if-elif
语句。
def print_hello():
print("Hello, World!")
def show_date():
from datetime import datetime
print(f"Current date and time: {datetime.now()}")
commands = {
'print_hello': print_hello,
'show_date': show_date
}
while True:
command = input("Enter your command (type 'exit' to quit): ")
if command.lower() == 'exit':
print("Exiting the program.")
break
elif command in commands:
commands[command]()
else:
print("Unknown command.")
在这个例子中,使用字典将指令映射到相应的函数,使得代码更具可读性和可扩展性。
三、应用案例:简单的计算器
为了更好地理解如何连续输入指令,我们可以实现一个简单的交互式计算器。用户可以输入算术表达式,程序将计算结果并返回。
- 实现简单计算器
def calculate(expression):
try:
result = eval(expression)
print(f"Result: {result}")
except Exception as e:
print(f"Error: {e}")
while True:
expression = input("Enter an expression to evaluate (type 'exit' to quit): ")
if expression.lower() == 'exit':
print("Exiting the calculator.")
break
else:
calculate(expression)
这个简单的计算器允许用户输入任意的算术表达式,然后使用eval()
函数计算并输出结果。需要注意的是,eval()
函数在执行用户输入时存在安全风险,因此仅适用于可信输入。
- 增强计算器功能
为了提高安全性和功能性,我们可以使用ast
模块来解析表达式,并限制支持的操作。
import ast
import operator
定义支持的操作符
operators = {
ast.Add: operator.add,
ast.Sub: operator.sub,
ast.Mult: operator.mul,
ast.Div: operator.truediv,
ast.Pow: operator.pow
}
def eval_expr(expr):
try:
tree = ast.parse(expr, mode='eval')
return eval_(tree.body)
except Exception as e:
print(f"Error: {e}")
def eval_(node):
if isinstance(node, ast.BinOp):
op = operators[type(node.op)]
return op(eval_(node.left), eval_(node.right))
elif isinstance(node, ast.Num):
return node.n
else:
raise TypeError(node)
while True:
expression = input("Enter an expression to evaluate (type 'exit' to quit): ")
if expression.lower() == 'exit':
print("Exiting the calculator.")
break
else:
result = eval_expr(expression)
if result is not None:
print(f"Result: {result}")
在这个增强版的计算器中,我们使用ast
模块来解析用户输入的算术表达式,并仅支持指定的操作符。这样可以有效防止不安全的代码执行。
四、总结
连续输入指令在Python编程中是一个常见的需求,通过使用循环、input()
函数以及条件判断,可以灵活实现不同的功能场景。while循环结合input()函数是实现连续输入的基础方法,通过添加输入验证和条件判断,可以提高程序的鲁棒性和可扩展性。在实现复杂功能时,可以通过函数映射和模块化设计来保持代码的清晰和可维护性。特别是在涉及到用户输入的场景时,始终要注意输入的安全性,以防止恶意输入导致程序崩溃或安全漏洞。
相关问答FAQs:
在Python中如何实现多行输入?
在Python中,您可以使用三重引号('''或""")来输入多行字符串,或者使用循环和列表来收集多行输入。通过input()
函数,可以在每次调用时获取一行输入,并存储在列表中。以下是一个简单示例:
lines = []
while True:
line = input("请输入一行(输入空行结束):")
if line == "":
break
lines.append(line)
这样,您可以连续输入多行指令,直到输入空行为止。
如何在Python中执行用户输入的指令?
可以使用exec()
函数来执行用户输入的Python代码。请注意,使用exec()
时要非常小心,确保输入不会包含恶意代码。以下是一个例子:
while True:
command = input("请输入Python指令(输入'quit'退出):")
if command.lower() == 'quit':
break
exec(command)
在这个示例中,用户输入的指令会被执行,直到输入'quit'以结束程序。
是否可以通过脚本文件连续执行指令?
是的,您可以将多个Python指令写入一个脚本文件,并使用命令行或Python解释器来执行该文件。通过在命令行中输入python your_script.py
,Python将依次执行文件中的所有指令。这种方法特别适合需要重复执行的任务或长指令序列。
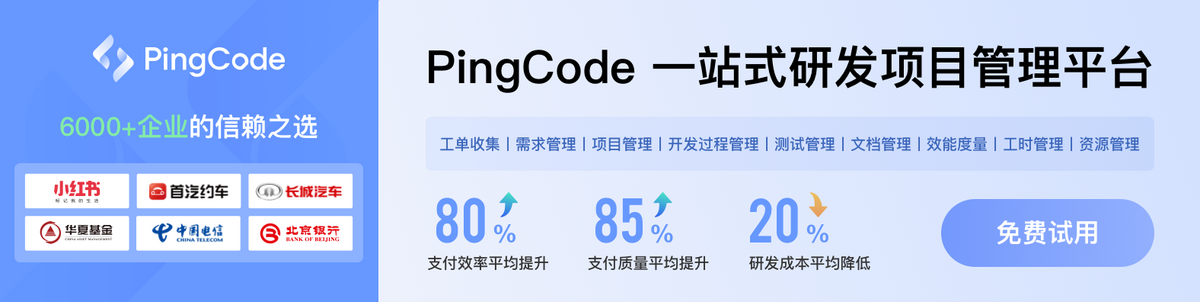