在Python中,使用Matplotlib库的subplots
函数可以方便地创建子图、使用subplot2grid
函数可以实现更复杂的布局、使用GridSpec
类可以实现灵活的网格布局。 其中,subplots
函数是最常用的方法,它允许用户在一个窗口中创建多个子图,并通过简单的索引方式进行管理。下面将详细介绍这几种方法的使用。
一、使用SUBPLOTS函数创建子图
subplots
函数是Matplotlib中最简便的方法之一,用于创建一组子图。它允许我们在一个图形窗口中布置多个图表。
import matplotlib.pyplot as plt
创建2x2的子图
fig, axs = plt.subplots(2, 2)
绘制在第一个子图
axs[0, 0].plot([1, 2, 3], [1, 4, 9])
axs[0, 0].set_title('First Subplot')
绘制在第二个子图
axs[0, 1].plot([1, 2, 3], [1, 2, 3])
axs[0, 1].set_title('Second Subplot')
绘制在第三个子图
axs[1, 0].plot([1, 2, 3], [9, 4, 1])
axs[1, 0].set_title('Third Subplot')
绘制在第四个子图
axs[1, 1].plot([1, 2, 3], [3, 2, 1])
axs[1, 1].set_title('Fourth Subplot')
调整子图之间的间距
plt.tight_layout()
显示图形
plt.show()
subplots
函数的参数说明
subplots(nrows, ncols)
:指定子图的行数和列数。
fig, axs = plt.subplots(...)
:返回一个Figure对象和一个包含子图Axes的数组。
- 自定义子图的大小和间距
subplots
函数还可以通过figsize
参数来设置整个图形的大小,而通过plt.tight_layout()
可以自动调整子图之间的间距。
fig, axs = plt.subplots(2, 2, figsize=(10, 8))
plt.tight_layout(pad=2.0)
二、使用SUBPLOT2GRID函数创建子图
subplot2grid
函数提供了更灵活的方式来创建子图,它允许我们在一个更复杂的网格中放置子图。
import matplotlib.pyplot as plt
创建一个3x3的网格
plt.subplot2grid((3, 3), (0, 0), colspan=3)
plt.title('Title of the Plot')
plt.plot([0, 1, 2], [0, 1, 0])
plt.subplot2grid((3, 3), (1, 0), colspan=2)
plt.plot([0, 1, 2], [2, 1, 0])
plt.subplot2grid((3, 3), (1, 2), rowspan=2)
plt.plot([0, 1, 2], [1, 2, 3])
plt.subplot2grid((3, 3), (2, 0))
plt.plot([0, 1, 2], [0, 1, 0])
plt.subplot2grid((3, 3), (2, 1))
plt.plot([0, 1, 2], [3, 2, 1])
plt.show()
subplot2grid
的参数说明
subplot2grid(shape, loc, rowspan, colspan)
:
shape
:网格的整体形状。loc
:子图的起始位置。rowspan
和colspan
:子图跨越的行数和列数。
三、使用GRIDSPEC创建子图
GridSpec
类提供了一种灵活的方法,用于在网格中放置子图。它允许对子图布局进行更精细的控制。
import matplotlib.pyplot as plt
import matplotlib.gridspec as gridspec
创建一个3x3的网格
gs = gridspec.GridSpec(3, 3)
fig = plt.figure()
ax1 = fig.add_subplot(gs[0, :])
ax1.plot([0, 1, 2], [0, 1, 0])
ax1.set_title('GridSpec Subplot 1')
ax2 = fig.add_subplot(gs[1, :-1])
ax2.plot([0, 1, 2], [2, 1, 0])
ax2.set_title('GridSpec Subplot 2')
ax3 = fig.add_subplot(gs[1:, -1])
ax3.plot([0, 1, 2], [1, 2, 3])
ax3.set_title('GridSpec Subplot 3')
ax4 = fig.add_subplot(gs[-1, 0])
ax4.plot([0, 1, 2], [0, 1, 0])
ax4.set_title('GridSpec Subplot 4')
ax5 = fig.add_subplot(gs[-1, -2])
ax5.plot([0, 1, 2], [3, 2, 1])
ax5.set_title('GridSpec Subplot 5')
plt.tight_layout()
plt.show()
GridSpec
的参数说明
GridSpec(nrows, ncols)
:指定网格的行数和列数。
通过gs[row, col]
来指定子图的位置。
- 灵活的子图布局
GridSpec
允许我们通过指定子图的行和列来实现灵活的布局,并能够通过fig.add_subplot
来为每个子图添加内容。
四、子图中的共享轴
在创建子图时,可能需要共享轴以便于对比不同子图的数据。Matplotlib允许我们共享子图的x轴或y轴。
fig, axs = plt.subplots(2, 2, sharex=True, sharey=True)
axs[0, 0].plot([1, 2, 3], [1, 4, 9])
axs[0, 1].plot([1, 2, 3], [1, 2, 3])
axs[1, 0].plot([1, 2, 3], [9, 4, 1])
axs[1, 1].plot([1, 2, 3], [3, 2, 1])
plt.tight_layout()
plt.show()
sharex=True
:共享x轴。sharey=True
:共享y轴。
五、为每个子图添加独立的图例和标签
在复杂的子图布局中,为每个子图添加独立的图例和标签可以帮助更好地理解每个子图所表示的数据。
fig, axs = plt.subplots(2, 2)
axs[0, 0].plot([1, 2, 3], [1, 4, 9], label='Line 1')
axs[0, 0].set_title('First Subplot')
axs[0, 0].legend()
axs[0, 1].plot([1, 2, 3], [1, 2, 3], label='Line 2')
axs[0, 1].set_title('Second Subplot')
axs[0, 1].legend()
axs[1, 0].plot([1, 2, 3], [9, 4, 1], label='Line 3')
axs[1, 0].set_title('Third Subplot')
axs[1, 0].legend()
axs[1, 1].plot([1, 2, 3], [3, 2, 1], label='Line 4')
axs[1, 1].set_title('Fourth Subplot')
axs[1, 1].legend()
plt.tight_layout()
plt.show()
在每个子图上使用set_title
设置标题,使用legend
添加图例。
六、总结
通过使用Matplotlib的subplots
、subplot2grid
和GridSpec
,可以在Python中创建多种布局的子图。这些工具提供了灵活的方法来组织和展示数据。通过共享轴和为每个子图添加独立的图例和标签,可以进一步增强图形的可读性和专业性。在实际应用中,根据数据展示的需求选择合适的子图布局方法,以便更好地传达信息。
相关问答FAQs:
如何在Python中创建多个子图?
在Python中,可以使用Matplotlib库来创建多个子图。使用plt.subplots()
函数可以很方便地设置多个子图的行和列。例如,fig, axs = plt.subplots(2, 2)
会生成一个2×2的子图布局。每个子图都可以通过axs
索引进行访问,从而实现独立绘图。
使用Seaborn绘制子图时,有哪些技巧?
Seaborn是建立在Matplotlib之上的高级绘图库,支持更加美观的图表。当使用Seaborn绘制子图时,可以利用facetgrid
和pairplot
等方法来轻松实现。例如,通过sns.FacetGrid(data, col='column_name')
可以自动生成多个子图,以便于比较不同类别的数据。
如何自定义子图的外观和布局?
在创建子图后,可以通过fig.tight_layout()
来自动调整子图之间的间距,以避免重叠。同时,可以使用set_title()
、set_xlabel()
和set_ylabel()
等方法为每个子图添加标题和标签。此外,调整子图的大小和比例也可以通过figsize
参数来实现,例如plt.subplots(figsize=(10, 5))
。
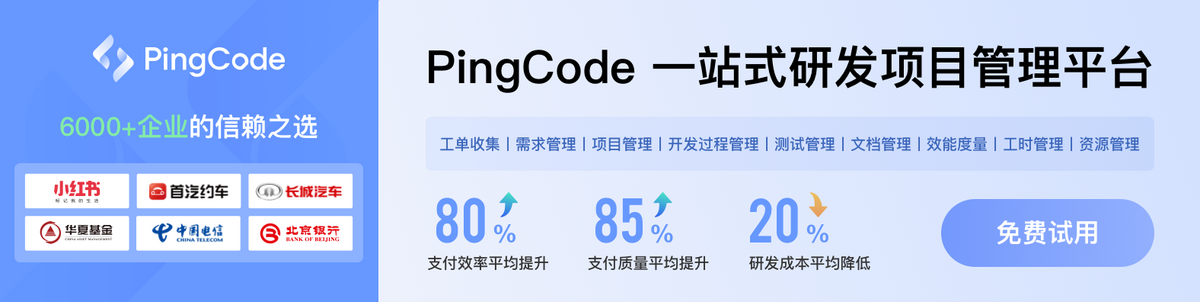