一、在Python中清理指定字符的方法
在Python中,清理字符串中特定字符的方法有多种,主要包括:使用字符串的replace()方法、使用正则表达式、使用自定义函数。其中,使用字符串的replace()方法是最为简单和直接的方式,而正则表达式则提供了更为强大的功能,适合复杂的字符清理需求。例如,使用replace()方法时,只需调用字符串对象的replace()方法,将指定的字符替换为空字符串即可完成清理。接下来,我们将详细探讨这些方法。
二、使用字符串的replace()方法
使用replace()方法是清理字符串中特定字符的最简单方法。这个方法直接调用字符串对象的replace()方法,将指定字符替换为其他字符(通常为空字符串)。例如:
text = "Hello, World!"
cleaned_text = text.replace(",", "")
print(cleaned_text) # 输出: Hello World!
- replace()的基本用法
replace()方法的基本用法是replace(old, new[, count]),其中old为要替换的旧字符串,new为替换后的新字符串,count为可选参数,指定替换的次数。如果不指定count,则替换所有出现的old字符串。
- 替换多个字符
要替换多个不同的字符,可以多次调用replace()方法。虽然这种方法简单直接,但当字符集较大时,代码可读性较差。
text = "Hello, World!"
cleaned_text = text.replace(",", "").replace("!", "")
print(cleaned_text) # 输出: Hello World
三、使用正则表达式
正则表达式是处理字符串中复杂匹配和替换任务的强大工具。在Python中,re模块提供了对正则表达式的支持。可以使用re.sub()函数替换符合特定模式的字符。
- re.sub()的基本用法
re.sub(pattern, repl, string, count=0, flags=0)用于替换字符串中符合正则表达式的部分。pattern是正则表达式模式,repl是替换的新字符串,string是要处理的字符串,count是替换次数,flags是正则表达式的标志位。
import re
text = "Hello, World!"
cleaned_text = re.sub(r"[,!]", "", text)
print(cleaned_text) # 输出: Hello World
- 复杂字符清理
正则表达式可以匹配复杂的字符模式,例如清理所有非字母字符:
cleaned_text = re.sub(r"[^a-zA-Z]", "", text)
print(cleaned_text) # 输出: HelloWorld
四、自定义函数进行清理
对于一些特定需求,可以编写自定义函数来处理字符清理。自定义函数提供了更高的灵活性,适合需要多次使用或逻辑复杂的场景。
- 基本自定义函数
可以编写一个简单的函数,循环遍历字符串中的每个字符,并根据条件决定是否保留。
def clean_string(s, chars_to_remove):
return ''.join(c for c in s if c not in chars_to_remove)
text = "Hello, World!"
cleaned_text = clean_string(text, ",!")
print(cleaned_text) # 输出: Hello World
- 扩展自定义函数
可以扩展自定义函数,支持更多功能,例如忽略大小写、支持替换为其他字符等:
def clean_string(s, chars_to_remove, ignore_case=False):
if ignore_case:
chars_to_remove = chars_to_remove.lower()
return ''.join(c for c in s if c.lower() not in chars_to_remove)
else:
return ''.join(c for c in s if c not in chars_to_remove)
text = "Hello, World!"
cleaned_text = clean_string(text, ",!", ignore_case=True)
print(cleaned_text) # 输出: Hello World
五、使用str.translate()方法
Python的str.translate()方法结合str.maketrans()可以高效地替换字符。这种方法适合处理简单的字符替换需求。
- 基本用法
首先,通过str.maketrans()创建一个翻译表,然后使用translate()方法应用该表。
text = "Hello, World!"
translation_table = str.maketrans("", "", ",!")
cleaned_text = text.translate(translation_table)
print(cleaned_text) # 输出: Hello World
- 处理复杂字符集
translate()方法可以高效处理大字符集的替换,比多次调用replace()性能更优。
六、结合各方法的优缺点
-
replace()方法适合简单字符替换,代码简单易读,但不适合处理复杂模式和大字符集。
-
正则表达式功能强大,适合复杂模式匹配和替换,但学习曲线较陡峭,可能在性能上不如专门的方法。
-
自定义函数提供了最高的灵活性,适合复杂逻辑和多次使用的场景,但实现成本较高。
-
translate()方法在替换大字符集时性能优异,但不如正则表达式灵活。
七、实际应用场景
-
数据预处理:在数据分析和机器学习中,清理数据集中的噪声字符是常见任务之一。
-
用户输入验证:在Web和桌面应用中,清理用户输入是提高安全性的重要措施。
-
日志分析:在日志分析中,清理无关字符有助于提高分析准确性。
八、性能比较与优化
- 性能测试
可以使用timeit模块对不同方法进行性能测试,选择最优方法。
import timeit
text = "Hello, World!" * 1000
setup = "from __main__ import clean_string, re; text='Hello, World!' * 1000"
print(timeit.timeit("text.replace(',', '').replace('!', '')", setup=setup, number=1000))
print(timeit.timeit("re.sub(r'[,!]', '', text)", setup=setup, number=1000))
print(timeit.timeit("clean_string(text, ',!')", setup=setup, number=1000))
- 优化建议
对于大数据集,尽量使用内置方法(如translate())以提高性能;对于复杂模式,正则表达式是最佳选择;在高频调用的场景中,编译正则表达式提高效率。
通过以上方法,Python提供了多种灵活的方式来清理字符串中的指定字符,可以根据具体需求选择适合的方法。
相关问答FAQs:
如何使用Python删除字符串中的特定字符?
在Python中,可以使用str.replace()
方法来删除字符串中的特定字符。只需将要删除的字符作为第一个参数传入,第二个参数留空即可。例如,要删除字符串中的字符'a'
,可以使用以下代码:
original_string = "banana"
cleaned_string = original_string.replace('a', '')
print(cleaned_string) # 输出: "bnn"
此外,使用str.translate()
与str.maketrans()
结合也能有效清理多个字符。
是否可以使用正则表达式来清理字符?
当然可以!Python的re
模块提供了强大的正则表达式功能,可以用来匹配并删除特定字符。例如,以下代码将删除所有数字字符:
import re
original_string = "abc123"
cleaned_string = re.sub(r'\d+', '', original_string)
print(cleaned_string) # 输出: "abc"
通过正则表达式,您可以灵活地定义要删除的字符模式,满足更复杂的需求。
能否清理多个不同的字符?
清理多个不同字符是完全可行的。可以使用str.replace()
多次调用,或者使用re.sub()
结合字符类。例如,想要同时删除字符'a'
和'b'
,可以使用如下代码:
original_string = "banana"
cleaned_string = original_string.replace('a', '').replace('b', '')
print(cleaned_string) # 输出: "nn"
另外,通过正则表达式可以使用[ab]
来表示要删除的字符集,例如:
import re
original_string = "banana"
cleaned_string = re.sub(r'[ab]', '', original_string)
print(cleaned_string) # 输出: "nn"
这种方法更为简洁高效。
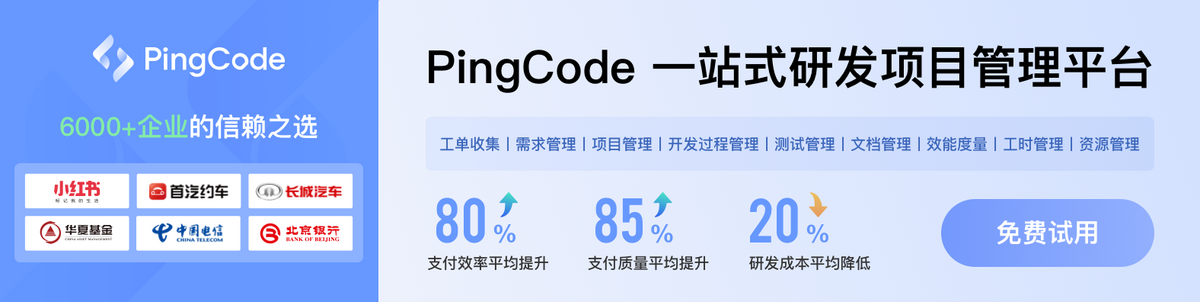