在Python中,sqrt函数用于计算一个数的平方根。要使用sqrt函数,通常需要导入math模块,因为sqrt是math模块中的一个函数。sqrt函数是通过调用math.sqrt(x)来实现的,其中x是您想要计算平方根的数。为了更好地理解,我们将详细讨论如何在Python中定义和使用sqrt函数。
一、导入math模块
在Python中,math模块提供了许多数学函数和常量,包括平方根函数sqrt。在使用sqrt函数之前,必须先导入math模块。可以通过以下方式导入:
import math
此行代码将math模块导入到您的Python程序中,使您可以访问math模块中的所有函数和常量。
二、使用math.sqrt函数
- 计算正数的平方根
要计算正数的平方根,可以直接使用math.sqrt函数。例如:
import math
number = 16
square_root = math.sqrt(number)
print(f"The square root of {number} is {square_root}")
在这个例子中,math.sqrt(16)返回4.0,这就是16的平方根。注意,math.sqrt返回一个浮点数。
- 计算小数的平方根
math.sqrt函数同样适用于小数。例如:
import math
decimal_number = 7.5
square_root = math.sqrt(decimal_number)
print(f"The square root of {decimal_number} is {square_root}")
在这个例子中,math.sqrt(7.5)返回大约2.7386127875258306。
三、处理负数
- math.sqrt函数不支持负数
当试图对负数使用math.sqrt函数时,Python会抛出一个ValueError。这是因为在实数范围内,负数没有平方根:
import math
negative_number = -9
try:
square_root = math.sqrt(negative_number)
except ValueError as e:
print(f"Error: {e}")
在上述代码中,math.sqrt(-9)将抛出ValueError。
- 使用cmath模块计算负数的平方根
如果需要计算负数的平方根,可以使用cmath模块,该模块支持复数数学运算:
import cmath
negative_number = -9
square_root = cmath.sqrt(negative_number)
print(f"The square root of {negative_number} is {square_root}")
在这个例子中,cmath.sqrt(-9)返回3j,表示9的平方根是3i(虚数单位)。
四、其他相关功能
- 定义自定义平方根函数
可以定义自己的平方根函数,使用牛顿法等算法来计算平方根:
def custom_sqrt(x, tolerance=1e-10):
if x < 0:
raise ValueError("Cannot compute the square root of a negative number")
estimate = x
while True:
better_estimate = (estimate + x / estimate) / 2
if abs(better_estimate - estimate) < tolerance:
return better_estimate
estimate = better_estimate
print(custom_sqrt(16))
- 使用numpy模块
numpy模块也提供了一个sqrt函数,可以对数组进行逐元素平方根计算:
import numpy as np
arr = np.array([1, 4, 9, 16])
square_roots = np.sqrt(arr)
print(square_roots)
在这个例子中,np.sqrt(arr)返回一个数组,其中包含输入数组每个元素的平方根。
五、应用场景
- 科学计算
平方根在许多科学计算中被广泛使用,例如物理学中的计算速度、能量和波动。
- 统计分析
在统计分析中,平方根转换用于处理数据的正态分布和方差稳定化。
- 计算几何
在计算几何中,平方根用于计算距离、面积和其他几何属性。
六、注意事项
- 精度问题
使用math.sqrt时,结果是浮点数,可能会出现精度误差。在需要高精度计算时,考虑使用Decimal模块。
- 性能考虑
对于大规模数组运算,使用numpy的sqrt函数比循环逐个计算性能更好。
- 复数运算
在涉及复数运算的场景下,使用cmath模块而不是math模块。
综上所述,Python中的sqrt函数是一个强大而灵活的工具,适用于多种应用场景。通过合理选择math、cmath和numpy模块,可以满足不同的计算需求。
相关问答FAQs:
在Python中,如何使用sqrt函数计算平方根?
在Python中,可以通过导入math模块来使用sqrt函数。使用方法非常简单,只需调用math.sqrt(x)
,其中x
是你想要计算平方根的非负数。例如,math.sqrt(16)
将返回4.0。
可以在Python中计算负数的平方根吗?
在Python的标准库中,math.sqrt()
不支持负数输入,因为负数的平方根是复数。如果需要计算负数的平方根,可以使用cmath
模块中的sqrt
函数。示例代码如下:
import cmath
result = cmath.sqrt(-16)
print(result) # 输出: 4j
是否有其他方法可以计算平方根?
除了使用math.sqrt()
和cmath.sqrt()
,Python还允许使用幂运算符<strong>
来计算平方根。你可以通过将数值提升到0.5的幂来实现。例如,16 </strong> 0.5
也会返回4.0。这种方法同样适用于正数和负数。对负数使用时,结果将是复数形式。
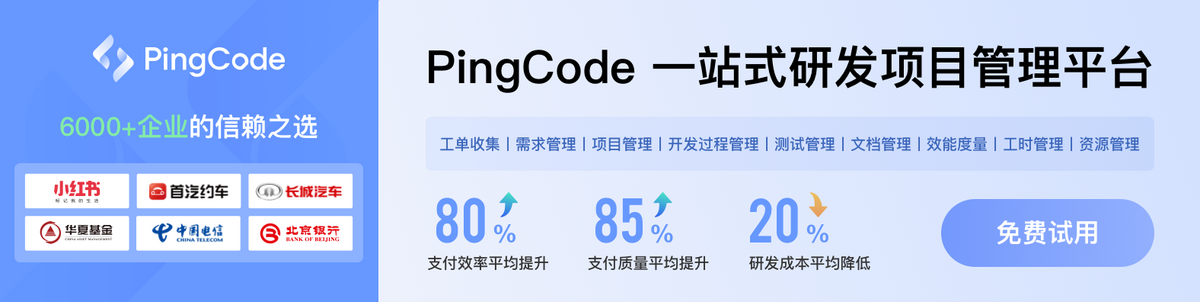