在Python中删除重复单词可以通过多种方法实现,例如使用集合(set)数据结构、字典(dict)或者正则表达式(regex)。集合是最直接的方法,因为集合本身不能包含重复元素;字典则可以通过键值对的唯一性来去重;正则表达式可用于更复杂的字符串处理。下面将详细介绍使用集合的方法。
一、使用集合去除重复单词
集合是一种无序的数据结构,其最大的特点就是不允许重复元素。利用这一特性,我们可以很方便地去除字符串中的重复单词。
1.1 将字符串转换为集合
首先,将字符串按照空格分割成单词列表,然后将列表转换为集合。这样,重复的单词会自动被去除。
def remove_duplicates_with_set(input_string):
words = input_string.split()
unique_words = set(words)
return " ".join(unique_words)
示例
input_string = "Python is great and Python is dynamic"
output_string = remove_duplicates_with_set(input_string)
print(output_string)
1.2 保持原有顺序
由于集合是无序的,如果需要保持单词的原有顺序,可以使用字典来实现。Python 3.7之后,字典也保持插入顺序。
def remove_duplicates_keep_order(input_string):
words = input_string.split()
seen = {}
for word in words:
if word not in seen:
seen[word] = True
return " ".join(seen.keys())
示例
input_string = "Python is great and Python is dynamic"
output_string = remove_duplicates_keep_order(input_string)
print(output_string)
二、使用字典去除重复单词
字典在Python中的应用非常广泛,它可以用来去除重复单词并保持顺序。
2.1 字典的唯一性
字典的键具有唯一性,这可以用来去除重复。
def remove_duplicates_with_dict(input_string):
words = input_string.split()
unique_words = dict.fromkeys(words)
return " ".join(unique_words)
示例
input_string = "Python is great and Python is dynamic"
output_string = remove_duplicates_with_dict(input_string)
print(output_string)
三、使用正则表达式去除重复单词
正则表达式是一种强大的字符串处理工具,适用于复杂的文本处理场景。
3.1 使用正则表达式匹配单词
通过正则表达式可以检测并去除重复的连续单词。
import re
def remove_consecutive_duplicates(input_string):
return re.sub(r'\b(\w+)( \1\b)+', r'\1', input_string)
示例
input_string = "Python is is great and and Python is dynamic"
output_string = remove_consecutive_duplicates(input_string)
print(output_string)
四、优化与扩展
4.1 考虑大小写
在某些情况下,"Python"和"python"应该被视为相同的单词。可以将字符串转换为统一大小写来处理。
def remove_duplicates_case_insensitive(input_string):
words = input_string.lower().split()
unique_words = dict.fromkeys(words)
return " ".join(unique_words)
示例
input_string = "Python is great and python is dynamic"
output_string = remove_duplicates_case_insensitive(input_string)
print(output_string)
4.2 考虑标点符号
标点符号可能会干扰单词的识别,需要在处理前去除。
import string
def remove_duplicates_ignore_punctuation(input_string):
translator = str.maketrans('', '', string.punctuation)
stripped_input = input_string.translate(translator)
words = stripped_input.split()
unique_words = dict.fromkeys(words)
return " ".join(unique_words)
示例
input_string = "Python, is great! And Python is dynamic."
output_string = remove_duplicates_ignore_punctuation(input_string)
print(output_string)
五、性能和复杂性分析
在选择去重方法时,性能可能是一个考虑因素。集合和字典在去重方面的复杂性较低,通常为O(n),其中n是单词数。这使得它们在大多数情况下是理想的选择。正则表达式虽然强大,但在复杂度和可读性上可能不如前两者。
六、应用场景
6.1 文本分析
在文本分析中,去除重复单词有助于提高文本的可读性和分析效率。例如,在自然语言处理(NLP)任务中,预处理步骤通常包括去重。
6.2 数据清洗
对于从网络抓取的数据或用户输入的数据,去除重复单词可以帮助提高数据质量。
七、总结
去除重复单词在Python中可以通过多种方式实现,选择合适的方法取决于具体需求和数据特点。无论是使用集合、字典还是正则表达式,每种方法都有其独特的优势和适用场景。理解每种方法的工作原理可以帮助我们在实践中更高效地解决问题。
相关问答FAQs:
如何使用Python有效地识别和删除文本中的重复单词?
在Python中,可以使用集合(set)来识别和删除重复单词。首先,将文本分割成单词列表,然后将其转换为集合以移除重复项。接着,可以将集合转回列表并根据原始顺序排序。此外,使用dict.fromkeys()
方法也是一个不错的选择,它可以保持单词的顺序。
在处理大文本文件时,如何高效删除重复单词?
对于大文本文件,可以逐行读取文件内容,并使用集合来存储唯一单词。逐行处理可以有效地减少内存占用,同时使用集合的特性确保每个单词只被存储一次。处理完成后,将集合转换为列表,并根据需要输出或保存结果。
有没有现成的Python库可以帮助删除文本中的重复单词?
确实存在一些Python库,如nltk
和pandas
,可以简化文本处理任务。使用nltk
可以轻松处理自然语言中的重复项,而pandas
提供了强大的数据处理功能,可以将文本数据转换为DataFrame并去除重复单词。这些库不仅提供了去重功能,还能进行更多文本分析和处理。
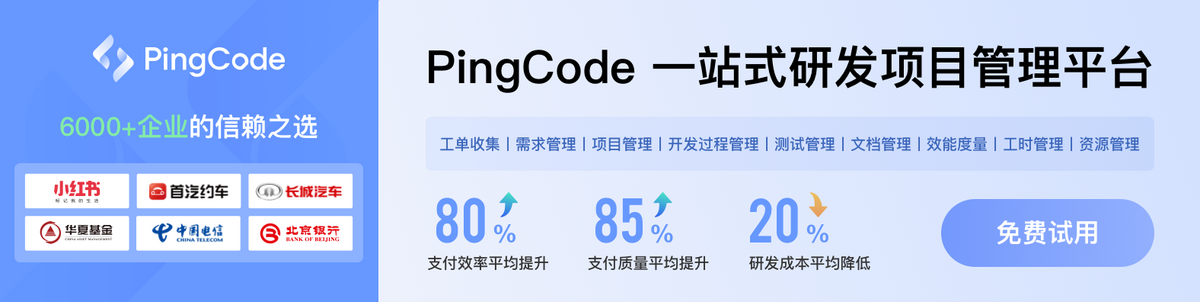