在Python中获取索引的方法有多种,使用enumerate()函数、通过列表的index()方法、使用字典的键值对、以及使用NumPy库中的where()函数等。使用enumerate()函数可以同时获取列表中的值及其对应的索引,这是最常用和简洁的方法之一。通过enumerate(),你可以在遍历列表时轻松地获取到每个元素的索引。下面我们将详细讨论这些方法及其应用场景。
一、使用ENUMERATE()函数
enumerate()函数是Python内置函数之一,它允许你在遍历列表时同时获取元素及其索引。这在需要同时访问元素和其位置时非常有用。
-
基本用法
使用enumerate()函数时,你可以通过for循环来遍历列表,并同时获取每个元素及其索引。该函数返回一个包含索引和值的元组。
fruits = ['apple', 'banana', 'cherry']
for index, fruit in enumerate(fruits):
print(f"Index: {index}, Fruit: {fruit}")
在这个例子中,输出将是:
Index: 0, Fruit: apple
Index: 1, Fruit: banana
Index: 2, Fruit: cherry
-
设置起始索引
enumerate()函数还允许你通过第二个参数设置起始索引。这在需要特定的索引起始值时非常有用。
for index, fruit in enumerate(fruits, start=1):
print(f"Index: {index}, Fruit: {fruit}")
这样,输出将会是:
Index: 1, Fruit: apple
Index: 2, Fruit: banana
Index: 3, Fruit: cherry
二、通过列表的INDEX()方法
当你知道一个元素的值并想要查找其索引时,列表的index()方法是一个理想选择。
-
基本用法
index()方法用于返回列表中第一个匹配项的索引。如果该项不存在,则会引发ValueError。
fruits = ['apple', 'banana', 'cherry']
index_of_banana = fruits.index('banana')
print(f"The index of banana is: {index_of_banana}")
输出为:
The index of banana is: 1
-
使用范围查找
index()方法还允许你指定查找的起始和结束位置,以便在列表的特定子集中查找。
numbers = [1, 2, 3, 2, 5]
index_of_two = numbers.index(2, 2) # 从索引2开始查找
print(f"The index of the second occurrence of 2 is: {index_of_two}")
输出为:
The index of the second occurrence of 2 is: 3
三、使用字典的键值对
在Python中,字典提供了一种使用键值对存储数据的方式。在某些情况下,字典结构可以帮助你快速检索数据的索引。
-
通过反向字典查找
假设你有一个字典,你可以通过创建一个反向字典来实现从值到键的快速查找。
fruit_prices = {'apple': 3, 'banana': 2, 'cherry': 5}
price_to_fruit = {v: k for k, v in fruit_prices.items()}
print(f"The fruit with price 2 is: {price_to_fruit[2]}")
输出为:
The fruit with price 2 is: banana
四、使用NumPy库中的WHERE()函数
NumPy是一个强大的科学计算库,它提供了许多便捷的数组操作函数,其中where()函数可以用于获取数组中满足特定条件的索引。
-
基本用法
在NumPy数组中,你可以使用where()函数来获取满足条件的元素索引。
import numpy as np
arr = np.array([10, 15, 20, 25, 30])
indices = np.where(arr > 20)
print(f"Indices of elements greater than 20: {indices}")
输出为:
Indices of elements greater than 20: (array([3, 4]),)
-
多条件查找
where()函数还支持多条件查找,可以结合逻辑操作符来实现更复杂的条件。
indices = np.where((arr > 10) & (arr < 30))
print(f"Indices of elements between 10 and 30: {indices}")
输出为:
Indices of elements between 10 and 30: (array([1, 2, 3]),)
五、其他方法与注意事项
除了上述方法,Python中还有其他技巧和注意事项可以帮助你更好地获取索引。
-
避免使用循环查找
虽然可以通过循环逐个比较元素来查找索引,但这种方法效率低下,特别是在大列表中。因此,建议优先使用enumerate()和index()等内置方法。
-
处理不存在的元素
在使用index()方法时,如果元素不存在于列表中,将引发ValueError。为避免程序崩溃,可以使用异常处理机制:
try:
index = fruits.index('orange')
except ValueError:
index = -1
print(f"Index of orange: {index}")
输出为:
Index of orange: -1
-
列表中的重复元素
如果列表中有重复元素,index()方法只会返回第一个匹配项的索引。要获取所有匹配项的索引,可以结合列表推导式:
numbers = [1, 2, 3, 2, 1]
indices_of_two = [i for i, x in enumerate(numbers) if x == 2]
print(f"Indices of 2: {indices_of_two}")
输出为:
Indices of 2: [1, 3]
通过这些方法和技巧,Python中获取索引变得更加简单和高效。根据具体应用场景选择合适的方法,可以大大提高代码的可读性和性能。
相关问答FAQs:
如何在Python中获取列表元素的索引?
在Python中,可以使用list.index()
方法来获取列表中某个元素的索引。例如,my_list.index(value)
将返回value
在my_list
中的第一个出现位置的索引。如果元素不存在于列表中,将抛出ValueError
异常,因此在使用之前最好确认元素是否存在。
在字典中如何获取键的索引?
虽然字典是无序的,但可以使用list(dict.keys()).index(key)
来找到特定键的“索引”。不过,建议使用字典的特性直接通过键访问值,而不是依赖于索引,因为在字典中,键的顺序不再重要。
如何使用enumerate()函数获取索引?enumerate()
函数可以让你在遍历列表时同时获取索引和元素。使用方法为for index, value in enumerate(my_list):
。这种方式不仅简洁,而且能提高代码的可读性,非常适合在需要索引的情况下使用。
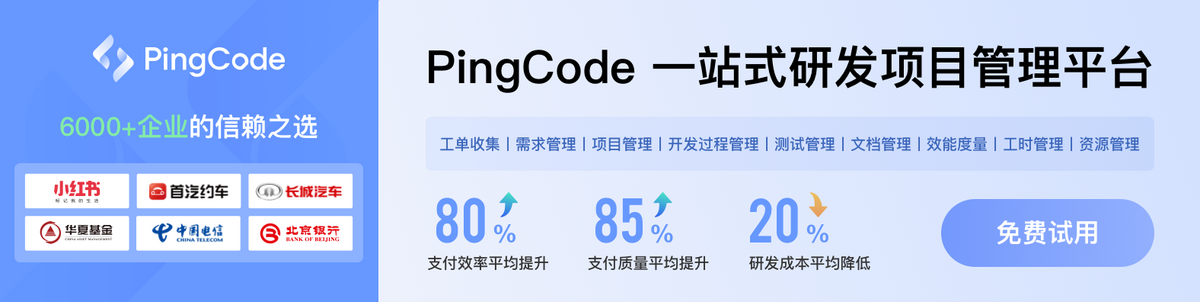