Python实现优先队列的方式有多种,常见的方式包括使用堆队列(heapq)模块、优先级队列(queue.PriorityQueue)类、自定义类等。通过heapq模块实现优先队列、通过PriorityQueue类实现线程安全的优先队列、通过自定义类实现更多功能是实现优先队列的几种方法。接下来将详细介绍如何使用这些方法在Python中实现优先队列。
一、HEAPQ模块
Python标准库中的heapq
模块提供了一组堆算法的实现,这使得我们可以方便地实现优先队列。
1. 堆队列基础
heapq
模块提供了一些函数来实现堆队列的功能,其中最常用的是heapq.heappush
和heapq.heappop
。heappush
用于将元素插入堆中,而heappop
用于从堆中删除最小元素。
import heapq
创建一个空列表来表示堆
heap = []
插入元素到堆中
heapq.heappush(heap, (1, 'task1'))
heapq.heappush(heap, (3, 'task3'))
heapq.heappush(heap, (2, 'task2'))
删除最小元素
task = heapq.heappop(heap)
print(task) # 输出: (1, 'task1')
2. 堆队列的应用
堆队列在优先队列中的应用非常广泛,比如在调度系统中,我们可以使用它来管理任务的优先级。
import heapq
class PriorityQueue:
def __init__(self):
self._queue = []
self._index = 0
def push(self, item, priority):
# 使用元组存储优先级和索引,保证优先级相同时按插入顺序排序
heapq.heappush(self._queue, (-priority, self._index, item))
self._index += 1
def pop(self):
return heapq.heappop(self._queue)[-1]
使用示例
pq = PriorityQueue()
pq.push('task1', 1)
pq.push('task2', 3)
pq.push('task3', 2)
print(pq.pop()) # 输出: task2
二、PRIORITYQUEUE类
Python的queue
模块提供了一个PriorityQueue
类,用于实现线程安全的优先队列。
1. 基本使用
PriorityQueue
类和heapq
模块类似,不过它是线程安全的,可以在多线程环境中使用。
from queue import PriorityQueue
pq = PriorityQueue()
pq.put((1, 'task1'))
pq.put((3, 'task3'))
pq.put((2, 'task2'))
while not pq.empty():
print(pq.get())
2. 线程安全的应用
在多线程环境中,可以使用PriorityQueue
来安全地管理任务的优先级。
from queue import PriorityQueue
from threading import Thread
def worker(pq):
while not pq.empty():
task = pq.get()
print(f"Processing {task[1]}")
pq.task_done()
pq = PriorityQueue()
pq.put((1, 'task1'))
pq.put((3, 'task3'))
pq.put((2, 'task2'))
threads = [Thread(target=worker, args=(pq,)) for _ in range(3)]
for thread in threads:
thread.start()
for thread in threads:
thread.join()
三、使用自定义类
如果需要实现更复杂的优先队列功能,可以通过自定义类来实现。
1. 自定义优先队列类
通过实现一个自定义类,可以更好地控制优先队列的行为,比如支持更新优先级、删除特定任务等。
import heapq
class CustomPriorityQueue:
def __init__(self):
self._queue = []
self._index = 0
def push(self, item, priority):
heapq.heappush(self._queue, (-priority, self._index, item))
self._index += 1
def pop(self):
return heapq.heappop(self._queue)[-1]
def remove(self, item):
self._queue = [(p, i, it) for (p, i, it) in self._queue if it != item]
heapq.heapify(self._queue)
def update(self, item, new_priority):
self.remove(item)
self.push(item, new_priority)
使用示例
cpq = CustomPriorityQueue()
cpq.push('task1', 1)
cpq.push('task2', 3)
cpq.push('task3', 2)
cpq.update('task1', 4)
print(cpq.pop()) # 输出: task1
2. 自定义类的应用
自定义优先队列类可以在复杂的系统中使用,例如实现定制化的任务调度、资源管理等功能。
四、应用场景
优先队列在很多应用场景中都能发挥重要作用。以下是一些常见的应用场景:
1. 任务调度
在任务调度系统中,优先队列可以用来管理任务的优先级,确保高优先级任务得到及时处理。
import heapq
import time
class TaskScheduler:
def __init__(self):
self._task_queue = []
self._index = 0
def add_task(self, task, priority):
heapq.heappush(self._task_queue, (-priority, self._index, task))
self._index += 1
def run(self):
while self._task_queue:
priority, index, task = heapq.heappop(self._task_queue)
print(f"Running task with priority {priority}: {task}")
time.sleep(1)
示例
scheduler = TaskScheduler()
scheduler.add_task('Email sending', 2)
scheduler.add_task('Data processing', 3)
scheduler.add_task('Backup', 1)
scheduler.run()
2. 图算法
在图算法中,优先队列常用于实现Dijkstra算法、A*算法等路径搜索算法。这些算法需要在每一步选择当前最优解,优先队列可以高效地实现这一点。
import heapq
def dijkstra(graph, start):
pq = []
heapq.heappush(pq, (0, start))
distances = {start: 0}
while pq:
current_distance, current_node = heapq.heappop(pq)
for neighbor, weight in graph[current_node].items():
distance = current_distance + weight
if neighbor not in distances or distance < distances[neighbor]:
distances[neighbor] = distance
heapq.heappush(pq, (distance, neighbor))
return distances
示例图
graph = {
'A': {'B': 1, 'C': 4},
'B': {'A': 1, 'C': 2, 'D': 5},
'C': {'A': 4, 'B': 2, 'D': 1},
'D': {'B': 5, 'C': 1}
}
运行Dijkstra算法
distances = dijkstra(graph, 'A')
print(distances)
五、总结
Python提供了多种方式来实现优先队列,包括heapq
模块和PriorityQueue
类等,这些工具都能高效地管理元素的优先级。在实际应用中,可以根据具体需求选择合适的方法来实现优先队列。此外,通过自定义类可以实现更复杂的优先队列功能,满足特殊场景的需求。无论是任务调度、图算法,还是其他需要管理优先级的场景,优先队列都能提供高效的解决方案。
相关问答FAQs:
优先队列在Python中是什么?
优先队列是一种特殊类型的队列,其中每个元素都有一个优先级。高优先级的元素会在低优先级元素之前被处理。在Python中,优先队列通常可以通过heapq
模块实现,该模块提供了一种高效的方式来管理优先级队列。
如何使用heapq模块来创建优先队列?
使用heapq
模块来实现优先队列非常简单。可以通过将元素放入一个列表中并使用heapq.heappush()
方法将元素按优先级插入队列。取出元素时,可以使用heapq.heappop()
方法,它会返回优先级最高的元素(即最小的元素)。示例代码如下:
import heapq
priority_queue = []
heapq.heappush(priority_queue, (1, '任务1')) # 优先级1
heapq.heappush(priority_queue, (3, '任务2')) # 优先级3
heapq.heappush(priority_queue, (2, '任务3')) # 优先级2
while priority_queue:
print(heapq.heappop(priority_queue))
如何在优先队列中处理相同优先级的元素?
在优先队列中,如果多个元素具有相同的优先级,heapq
会按照它们被添加到队列中的顺序来处理。这意味着先添加的元素会先被弹出。为了明确管理相同优先级的元素,可以在元组中加入一个序列号。例如:
import heapq
priority_queue = []
heapq.heappush(priority_queue, (1, '任务1', 1)) # 优先级1
heapq.heappush(priority_queue, (1, '任务2', 2)) # 优先级1
heapq.heappush(priority_queue, (3, '任务3', 3)) # 优先级3
while priority_queue:
print(heapq.heappop(priority_queue))
Python中的优先队列与其他数据结构相比有什么优势?
相较于其他数据结构,优先队列提供了高效的插入和删除操作。使用heapq
模块可以在O(log n)时间复杂度内完成插入和删除操作,这使得处理大量数据时性能优越。此外,优先队列非常适合用于需要频繁访问最高(或最低)优先级元素的场景,例如任务调度和图算法。
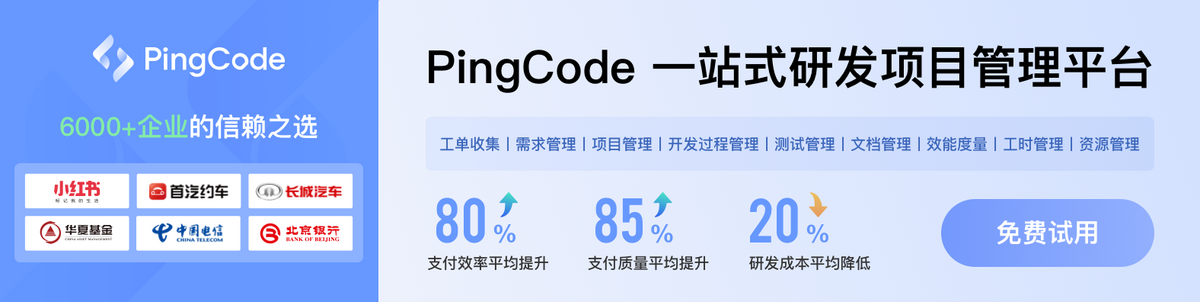