一、如何用Python操作PPT
用Python操作PPT可以通过使用python-pptx库、自动化生成和修改幻灯片、批量处理PPT文件、创建可视化报告等方法来实现。使用python-pptx库是其中比较常见和简单的方法,python-pptx是一个用于创建和修改PowerPoint文件的Python库,可以帮助用户快速生成和修改幻灯片内容。通过这个库,用户可以添加文本、图片、图表等元素到幻灯片中,并对其进行格式设置,从而实现自动化处理PPT文件的需求。
二、安装与基础使用
在使用python-pptx库之前,首先需要确保已安装该库。可以通过pip来安装:
pip install python-pptx
安装完成后,可以开始使用python-pptx来创建和修改PPT文件。首先,可以通过创建一个新的PPT对象来开始:
from pptx import Presentation
创建一个新的PPT对象
prs = Presentation()
创建PPT对象后,可以向其中添加幻灯片:
# 添加一张新的幻灯片
slide_layout = prs.slide_layouts[0] # 使用第一种幻灯片布局
slide = prs.slides.add_slide(slide_layout)
可以通过不同的布局选择来创建不同类型的幻灯片,例如只包含标题的幻灯片、包含标题和内容的幻灯片等。
三、添加文本和图片
在幻灯片中,文本和图片是最基本的元素,python-pptx提供了方便的方法来添加这些元素。
- 添加文本
可以通过幻灯片的shapes
对象来添加文本框,并设置文本内容:
# 添加标题
title = slide.shapes.title
title.text = "Hello, World!"
添加正文文本框
textbox = slide.shapes.add_textbox(Inches(2), Inches(2), Inches(5), Inches(1))
text_frame = textbox.text_frame
text_frame.text = "This is a text box."
- 添加图片
添加图片也非常简单,通过add_picture
方法可以将图片添加到幻灯片中:
from pptx.util import Inches
添加图片
img_path = 'path/to/image.jpg'
slide.shapes.add_picture(img_path, Inches(1), Inches(1), width=Inches(5), height=Inches(3))
四、修改幻灯片样式
除了添加文本和图片,python-pptx还支持修改幻灯片的样式,例如设置字体、颜色、对齐方式等。
- 设置字体和颜色
可以通过TextFrame
对象来设置文本的字体和颜色:
from pptx.dml.color import RGBColor
设置字体
text_frame.text = "Styled Text"
for paragraph in text_frame.paragraphs:
for run in paragraph.runs:
run.font.bold = True
run.font.size = Pt(24)
run.font.color.rgb = RGBColor(0x00, 0x00, 0xFF) # 设置为蓝色
- 设置对齐方式
同样可以通过TextFrame
来设置文本的对齐方式:
from pptx.util import Pt
text_frame.paragraphs[0].alignment = PP_ALIGN.CENTER # 设置为居中对齐
五、创建图表和表格
除了基本的文本和图片,python-pptx还支持创建复杂的图表和表格。
- 创建图表
可以通过使用ChartData
对象来定义图表的数据,并通过add_chart
方法将其添加到幻灯片中:
from pptx.chart.data import ChartData
from pptx.enum.chart import XL_CHART_TYPE
创建图表数据
chart_data = ChartData()
chart_data.categories = ['East', 'West', 'Midwest']
chart_data.add_series('Series 1', (19.2, 21.4, 16.7))
添加图表
x, y, cx, cy = Inches(2), Inches(2), Inches(5), Inches(3)
slide.shapes.add_chart(
XL_CHART_TYPE.COLUMN_CLUSTERED, x, y, cx, cy, chart_data
)
- 创建表格
添加表格可以通过add_table
方法来实现:
# 添加表格
rows, cols = 2, 2
left, top, width, height = Inches(2), Inches(2), Inches(4), Inches(1.5)
table = slide.shapes.add_table(rows, cols, left, top, width, height).table
设置表格内容
table.cell(0, 0).text = 'A'
table.cell(0, 1).text = 'B'
table.cell(1, 0).text = 'C'
table.cell(1, 1).text = 'D'
六、保存PPT文件
在完成所有的修改后,可以通过save
方法将PPT文件保存到指定路径:
prs.save('path/to/save/presentation.pptx')
七、批量处理PPT文件
在需要处理大量PPT文件时,可以编写脚本来自动化处理这些文件。例如,可以批量生成报告、修改内容或提取信息。
- 批量生成报告
假设需要根据一组数据生成多个报告,可以通过循环来实现:
data_list = [{'title': 'Report 1', 'content': 'Content 1'},
{'title': 'Report 2', 'content': 'Content 2'}]
for data in data_list:
prs = Presentation()
slide_layout = prs.slide_layouts[0]
slide = prs.slides.add_slide(slide_layout)
title = slide.shapes.title
title.text = data['title']
textbox = slide.shapes.add_textbox(Inches(2), Inches(2), Inches(5), Inches(1))
text_frame = textbox.text_frame
text_frame.text = data['content']
prs.save(f"{data['title']}.pptx")
- 批量修改内容
可以通过读取现有PPT文件并对其进行修改:
from pptx import Presentation
打开现有PPT文件
prs = Presentation('existing_presentation.pptx')
修改每个幻灯片的标题
for slide in prs.slides:
title = slide.shapes.title
if title:
title.text = "Updated Title"
保存修改后的文件
prs.save('updated_presentation.pptx')
八、创建可视化报告
通过Python脚本,可以从数据源生成可视化报告,并以PPT的形式输出。这在数据分析和商业报告中非常有用。
- 从数据生成图表
假设有一组销售数据,可以根据这些数据生成图表:
import pandas as pd
示例数据
data = {'Region': ['East', 'West', 'Midwest'], 'Sales': [200, 150, 300]}
df = pd.DataFrame(data)
创建图表数据
chart_data = ChartData()
chart_data.categories = df['Region']
chart_data.add_series('Sales', df['Sales'])
添加图表到PPT
prs = Presentation()
slide_layout = prs.slide_layouts[5] # 空白布局
slide = prs.slides.add_slide(slide_layout)
x, y, cx, cy = Inches(2), Inches(2), Inches(5), Inches(3)
slide.shapes.add_chart(
XL_CHART_TYPE.COLUMN_CLUSTERED, x, y, cx, cy, chart_data
)
prs.save('sales_report.pptx')
- 自定义报告格式
可以根据需要定制报告的格式,例如添加公司标志、设置统一的字体和颜色等。
# 添加公司标志
img_path = 'path/to/logo.png'
slide.shapes.add_picture(img_path, Inches(0.5), Inches(0.5), width=Inches(1.5))
设置统一的字体和颜色
for slide in prs.slides:
for shape in slide.shapes:
if not shape.has_text_frame:
continue
for paragraph in shape.text_frame.paragraphs:
for run in paragraph.runs:
run.font.name = 'Arial'
run.font.color.rgb = RGBColor(0x00, 0x00, 0x00) # 黑色
通过以上方法,Python可以灵活地操作PPT文件,实现自动化生成和修改幻灯片的需求。这种方法特别适合需要批量处理PPT文件或生成可视化报告的场景。
相关问答FAQs:
如何在Python中创建和编辑PPT文件?
使用Python操作PPT文件通常可以借助python-pptx
库。首先,确保安装该库,可以通过命令pip install python-pptx
来完成。安装后,您可以创建新的PPT文件,添加幻灯片、文本框、图片和其他元素。通过该库的各种方法,可以灵活地编辑现有PPT文件,例如修改文本、调整布局和应用样式。
使用Python操作PPT时需要注意哪些常见问题?
在使用Python操作PPT时,可能会遇到一些常见问题,例如文件格式不兼容、库的版本问题以及在不同操作系统下的表现差异。确保您的PPT文件为.pptx
格式,因为python-pptx
库不支持.ppt
格式。此外,确保使用最新版本的库,以避免因版本不一致而导致的错误。
如何在Python中插入图像到PPT幻灯片中?
在创建或编辑PPT时,可以轻松地插入图像。使用python-pptx
库的Slide.shapes.add_picture()
方法来实现。您需要提供图像的路径及其在幻灯片上的位置和大小。这样,您可以为幻灯片添加图像,使演示内容更加生动和吸引人。
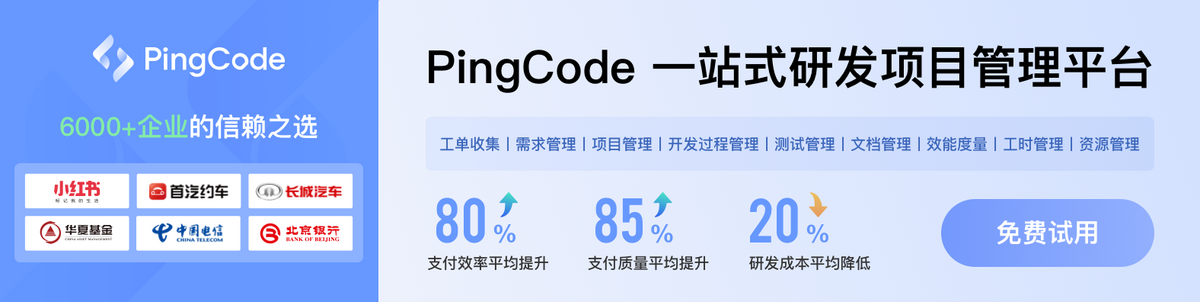