在Python中循环查找文本可以通过使用循环结构(如for或while)以及文本查找方法(如str.find()、正则表达式)来实现。通过使用这些方法,可以在文本中搜索特定的子字符串、关键词或模式,并执行相应的操作。下面,我们将详细介绍如何在Python中循环查找文本,包括使用字符串内置方法、正则表达式和迭代器等技术。
一、使用字符串内置方法
Python提供了一些字符串内置方法,可以方便地在字符串中查找子字符串。这些方法包括str.find()和str.index()等。
- 使用str.find()
str.find()方法用于查找子字符串在字符串中的位置。它返回子字符串的第一个字符的索引,如果没有找到,则返回-1。可以使用循环来查找文本中的所有匹配项。
def find_all_occurrences(text, sub):
occurrences = []
index = text.find(sub)
while index != -1:
occurrences.append(index)
index = text.find(sub, index + 1)
return occurrences
text = "This is a test text for testing text search."
sub = "text"
print(find_all_occurrences(text, sub))
在这个例子中,find_all_occurrences函数返回所有出现子字符串的起始索引。
- 使用str.index()
str.index()方法与str.find()类似,但在没有找到子字符串时会引发ValueError异常。这可能在某些情况下更有用,因为它可以强制处理未找到的情况。
def find_all_occurrences_with_index(text, sub):
occurrences = []
try:
index = text.index(sub)
while index != -1:
occurrences.append(index)
index = text.index(sub, index + 1)
except ValueError:
pass
return occurrences
text = "This is a test text for testing text search."
sub = "text"
print(find_all_occurrences_with_index(text, sub))
二、使用正则表达式
正则表达式是一个强大的工具,用于在文本中查找复杂的模式。Python的re模块提供了对正则表达式的支持。
- re.finditer()
re.finditer()函数返回一个迭代器,生成匹配对象,可以在文本中查找所有匹配的模式。
import re
def find_with_regex(text, pattern):
matches = []
for match in re.finditer(pattern, text):
matches.append((match.start(), match.group()))
return matches
text = "This is a test text for testing text search."
pattern = r"text"
print(find_with_regex(text, pattern))
在这个例子中,我们使用re.finditer()查找所有出现的“text”模式,并返回它们的起始索引和匹配内容。
- re.findall()
re.findall()函数返回一个列表,包含所有匹配的字符串。
def find_all_with_regex(text, pattern):
return re.findall(pattern, text)
text = "This is a test text for testing text search."
pattern = r"text"
print(find_all_with_regex(text, pattern))
虽然re.findall()提供了所有匹配项的列表,但它不提供索引信息。对于需要知道匹配位置的情况,re.finditer()更为合适。
三、使用迭代器和生成器
在某些情况下,使用迭代器和生成器可以使代码更为简洁和高效。
- 生成器函数
生成器函数可以用于查找文本中的所有匹配项,并在需要时生成结果。
def find_occurrences_generator(text, sub):
index = text.find(sub)
while index != -1:
yield index
index = text.find(sub, index + 1)
text = "This is a test text for testing text search."
sub = "text"
for occurrence in find_occurrences_generator(text, sub):
print(occurrence)
生成器函数find_occurrences_generator在查找匹配项时使用yield返回结果,使其在内存使用上更为高效。
- 使用itertools模块
itertools模块提供了生成器工具,可以用于创建复杂的循环逻辑。
import itertools
def find_all_with_itertools(text, sub):
return list(itertools.takewhile(lambda x: x != -1, (text.find(sub, i) for i in itertools.count())))
text = "This is a test text for testing text search."
sub = "text"
print(find_all_with_itertools(text, sub))
在这个例子中,itertools.count()用于生成一个无限递增的索引序列,结合takewhile用于查找所有出现的子字符串。
四、优化查找性能
在处理大量数据或长文本时,优化查找性能非常重要。以下是一些优化技巧:
- 减少搜索范围
如果已知子字符串只能出现在文本的某个部分,可以使用字符串切片来减少查找范围。
text = "This is a test text for testing text search."
sub = "text"
start, end = 10, 40 # 只在文本的某个范围内查找
print(find_all_occurrences(text[start:end], sub))
- 使用更高效的数据结构
在某些情况下,将文本转换为更高效的数据结构(如字典或集合)可以加快查找速度。
- 提前终止循环
如果只需要找到第一个匹配项,或者在找到足够数量的匹配项后,可以提前终止查找循环。
def find_first_occurrence(text, sub):
index = text.find(sub)
return index if index != -1 else "Not found"
text = "This is a test text for testing text search."
sub = "text"
print(find_first_occurrence(text, sub))
通过以上多种方法,可以在Python中高效地实现循环查找文本的功能,根据具体需求选择合适的技术和优化策略。
相关问答FAQs:
如何在Python中实现循环查找文本的功能?
在Python中,可以使用for
循环结合字符串的方法进行文本查找。例如,使用in
运算符检查子字符串是否存在于主字符串中,或使用str.find()
方法查找子字符串的索引。以下是一个基本示例:
text = "这是一个示例文本,包含多个示例。"
keyword = "示例"
for i in range(len(text)):
if text[i:i+len(keyword)] == keyword:
print(f"找到'{keyword}'在位置: {i}")
如何处理大小写敏感的文本查找?
当需要忽略大小写进行文本查找时,可以将文本和关键字都转换为同一种大小写。使用str.lower()
或str.upper()
方法可以方便地实现这一点。以下是一个示例:
text = "这是一个示例文本,包含多个示例。"
keyword = "示例"
text_lower = text.lower()
keyword_lower = keyword.lower()
for i in range(len(text_lower)):
if text_lower[i:i+len(keyword_lower)] == keyword_lower:
print(f"找到'{keyword}'在位置: {i}")
在循环查找文本时如何避免重复匹配?
为了避免在循环查找中重复匹配相同的关键字,可以在找到匹配后,更新循环的起始位置。通过使用break
语句或调整循环变量,可以有效地跳过已匹配的部分。以下是一个示例:
text = "这是一个示例文本,包含多个示例。"
keyword = "示例"
start = 0
while start < len(text):
start = text.find(keyword, start)
if start == -1:
break
print(f"找到'{keyword}'在位置: {start}")
start += len(keyword) # 更新起始位置,避免重复
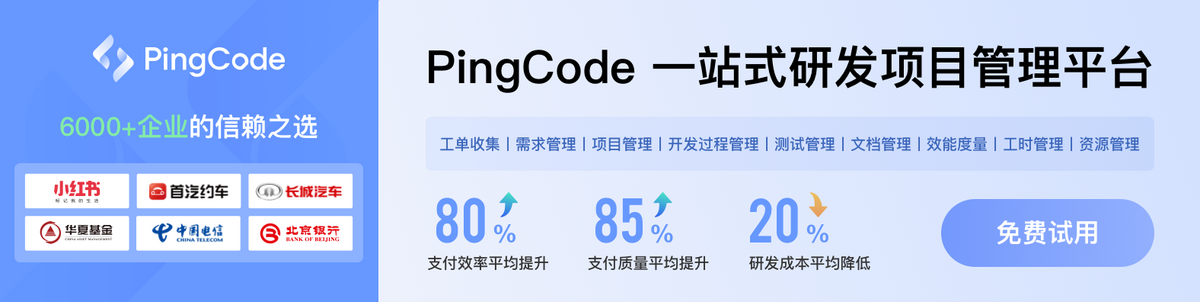