用Python访问文件的方法包括使用内置函数open()、利用上下文管理器with、以及读取文件内容等步骤。建议使用with语句管理文件的打开和关闭,可以确保文件在操作完成后正确关闭,避免资源泄露。
在Python中访问文件的过程通常包括几个步骤:打开文件、读取或写入文件、关闭文件。下面将详细介绍这些步骤及其相关的实践。
一、使用open()函数打开文件
在Python中,访问文件的第一步是打开文件。可以使用内置的open()函数来实现。open()函数的基本语法是:
open(file, mode='r', buffering=-1, encoding=None, errors=None, newline=None, closefd=True, opener=None)
- file: 表示文件路径,可以是绝对路径或相对路径。
- mode: 表示文件的打开模式,比如'r'表示读取,'w'表示写入,'a'表示追加等。默认是'r'。
- buffering: 设置缓冲策略,默认为-1。
- encoding: 指定编码,文本模式下推荐使用'utf-8'。
- errors: 设置错误处理策略。
- newline: 控制换行符的处理。
- closefd: 控制文件描述符的关闭。
- opener: 自定义文件打开函数。
二、读取文件内容
读取文件内容的方法有多种,取决于文件的大小和读取需求。
- read()方法
read()方法用于一次性读取整个文件的内容。适用于小文件。
with open('example.txt', 'r', encoding='utf-8') as file:
content = file.read()
print(content)
- readline()方法
readline()方法用于逐行读取文件内容,适用于需要按行处理的文件。
with open('example.txt', 'r', encoding='utf-8') as file:
line = file.readline()
while line:
print(line, end='')
line = file.readline()
- readlines()方法
readlines()方法将文件的每一行读入一个列表中,适用于需要一次性获取所有行的场景。
with open('example.txt', 'r', encoding='utf-8') as file:
lines = file.readlines()
for line in lines:
print(line, end='')
三、写入文件内容
写入文件内容同样可以使用不同的方法,取决于写入需求。
- write()方法
write()方法用于将字符串写入文件。注意,写入时需要打开文件模式为'w'或'a'。
with open('example.txt', 'w', encoding='utf-8') as file:
file.write("Hello, World!")
- writelines()方法
writelines()方法用于将多个字符串写入文件。需要提供一个字符串列表。
lines = ["Hello, World!\n", "Welcome to Python file handling.\n"]
with open('example.txt', 'w', encoding='utf-8') as file:
file.writelines(lines)
四、使用上下文管理器with
使用with语句可以自动管理文件的打开和关闭,确保文件在操作完成后正确关闭。这是推荐的文件操作方式。
with open('example.txt', 'r', encoding='utf-8') as file:
content = file.read()
print(content)
五、文件路径处理
在处理文件时,路径是一个重要的方面。在不同操作系统中,文件路径的格式不同。Python提供了os模块和pathlib模块来处理路径问题。
- os模块
os模块提供了许多处理文件和目录的方法。
import os
获取当前工作目录
current_directory = os.getcwd()
连接路径
file_path = os.path.join(current_directory, 'example.txt')
- pathlib模块
pathlib模块提供了面向对象的路径操作方法。
from pathlib import Path
获取当前工作目录
current_directory = Path.cwd()
连接路径
file_path = current_directory / 'example.txt'
六、错误处理
在文件操作中,可能会出现各种错误,比如文件不存在、权限不足等。可以使用try-except块来处理这些错误。
try:
with open('example.txt', 'r', encoding='utf-8') as file:
content = file.read()
print(content)
except FileNotFoundError:
print("The file does not exist.")
except PermissionError:
print("You do not have permission to access this file.")
except Exception as e:
print(f"An error occurred: {e}")
七、文件的追加和修改
有时需要在现有文件的末尾追加内容,可以使用'a'模式打开文件。
with open('example.txt', 'a', encoding='utf-8') as file:
file.write("\nThis is an appended line.")
对于修改文件,可以先读取文件内容,进行修改后再写入文件。
# 读取文件内容
with open('example.txt', 'r', encoding='utf-8') as file:
lines = file.readlines()
修改内容
lines = [line.replace('old_string', 'new_string') for line in lines]
写入修改后的内容
with open('example.txt', 'w', encoding='utf-8') as file:
file.writelines(lines)
八、使用CSV文件
Python中常见的文件格式之一是CSV文件。可以使用csv模块进行操作。
import csv
读取CSV文件
with open('example.csv', 'r', newline='', encoding='utf-8') as csvfile:
reader = csv.reader(csvfile)
for row in reader:
print(row)
写入CSV文件
with open('example.csv', 'w', newline='', encoding='utf-8') as csvfile:
writer = csv.writer(csvfile)
writer.writerow(['Column1', 'Column2', 'Column3'])
writer.writerow(['Value1', 'Value2', 'Value3'])
九、使用JSON文件
JSON文件是另一种常见的文件格式,Python提供了json模块进行处理。
import json
读取JSON文件
with open('example.json', 'r', encoding='utf-8') as jsonfile:
data = json.load(jsonfile)
print(data)
写入JSON文件
data = {'name': 'John', 'age': 30, 'city': 'New York'}
with open('example.json', 'w', encoding='utf-8') as jsonfile:
json.dump(data, jsonfile, ensure_ascii=False, indent=4)
十、二进制文件操作
对于二进制文件,如图像、音频,可以使用'b'模式。
# 读取二进制文件
with open('example.jpg', 'rb') as binary_file:
data = binary_file.read()
写入二进制文件
with open('copy_example.jpg', 'wb') as binary_file:
binary_file.write(data)
通过以上步骤和示例,您应该能够在Python中有效地访问和处理文件。无论是文本文件、CSV、JSON还是二进制文件,Python都提供了灵活和强大的工具来满足您的需求。
相关问答FAQs:
如何在Python中打开和读取文件?
在Python中,可以使用内置的open()
函数来打开文件。通过指定文件名和模式(如'r'表示只读),可以读取文件内容。例如:
with open('example.txt', 'r') as file:
content = file.read()
print(content)
使用with
语句可以确保文件在操作完成后自动关闭,防止资源泄露。
Python中如何写入文件?
要将内容写入文件,可以使用open()
函数并指定写入模式(如'w'表示写入,'a'表示追加)。以下是一个写入文件的示例:
with open('output.txt', 'w') as file:
file.write('Hello, World!')
通过这种方式,可以创建新文件或覆盖已存在文件的内容。
如何处理Python中的文件异常?
在进行文件操作时,可能会遇到各种异常情况,如文件不存在或权限不足。使用try...except
语句可以捕获并处理这些异常。示例如下:
try:
with open('nonexistent.txt', 'r') as file:
content = file.read()
except FileNotFoundError:
print("文件未找到,请检查文件名和路径。")
except PermissionError:
print("您没有权限访问此文件。")
这种方式能够使程序更稳健,并提供用户友好的反馈。
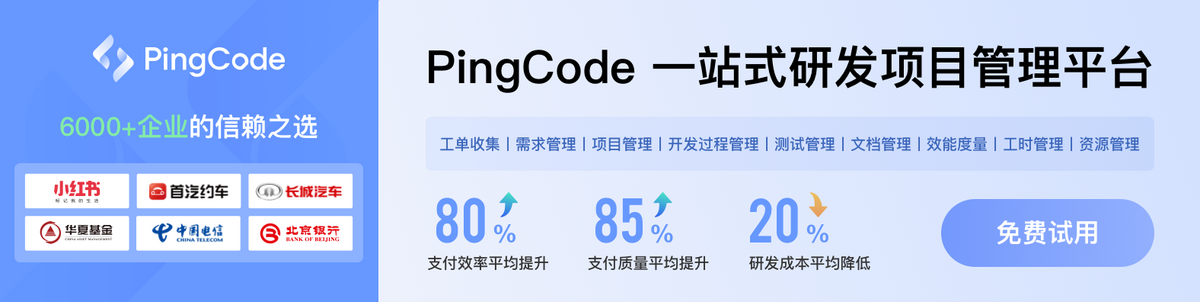