要在Python中使用Git,可以使用GitPython库、os模块、subprocess模块等方法。其中,GitPython库最为简单,os模块和subprocess模块则提供了更大的灵活性。下面将详细介绍如何使用这些方法来操作Git。
一、GITPYTHON库
GitPython是一个Python库,专门用于在Python中操作Git仓库。它提供了简单而强大的接口来处理Git命令。
- 安装GitPython
要使用GitPython,首先需要安装它。可以通过以下命令安装:
pip install GitPython
- 使用GitPython初始化Git仓库
使用GitPython初始化一个新的Git仓库非常简单。以下是一个示例代码:
import git
初始化一个新的Git仓库
repo = git.Repo.init('/path/to/repo')
在这个例子中,我们使用Repo.init
方法来初始化一个新的Git仓库。需要提供仓库的路径。
- 克隆Git仓库
GitPython可以很容易地克隆一个Git仓库。以下是一个简单的示例:
import git
克隆Git仓库
repo = git.Repo.clone_from('https://github.com/your/repo.git', '/path/to/destination')
在这个例子中,我们使用Repo.clone_from
方法来克隆一个Git仓库。需要提供仓库的URL和克隆的目标路径。
- 检查仓库状态
可以使用GitPython检查仓库的状态,比如哪些文件被修改或未跟踪:
repo = git.Repo('/path/to/repo')
untracked_files = repo.untracked_files
changed_files = [item.a_path for item in repo.index.diff(None)]
这段代码获取了未跟踪文件列表和已修改文件列表。
- 提交更改
GitPython还可以用于提交更改:
repo = git.Repo('/path/to/repo')
repo.git.add(all=True)
repo.index.commit('Your commit message')
这段代码将所有更改添加到Git索引,并提交带有信息的更改。
二、OS模块
使用os模块可以直接在Python中执行Git命令。虽然这种方法没有GitPython那么简洁,但它提供了更大的灵活性。
- 使用os.system执行Git命令
可以使用os.system来执行Git命令:
import os
os.system('git clone https://github.com/your/repo.git')
这段代码克隆了一个Git仓库。
- 使用os.popen获取命令输出
如果需要获取命令的输出,可以使用os.popen:
import os
stream = os.popen('git status')
output = stream.read()
print(output)
这段代码执行git status
命令并打印其输出。
三、SUBPROCESS模块
subprocess模块是Python中执行外部命令的强大工具。它提供了对命令执行的更细粒度控制。
- 使用subprocess.run执行Git命令
subprocess.run是执行命令的推荐方法。它可以捕获输出,处理错误等:
import subprocess
result = subprocess.run(['git', 'status'], capture_output=True, text=True)
print(result.stdout)
这段代码执行git status
命令并打印其输出。
- 使用subprocess.Popen进行更复杂的操作
对于更复杂的操作,可以使用subprocess.Popen:
import subprocess
process = subprocess.Popen(['git', 'status'], stdout=subprocess.PIPE, stderr=subprocess.PIPE)
stdout, stderr = process.communicate()
print(stdout.decode())
这段代码捕获并打印git status
命令的标准输出和错误输出。
四、综合应用实例
结合以上方法,可以创建一个更复杂的应用程序来管理Git仓库。以下是一个示例,展示了如何使用Python创建一个简单的Git管理工具:
import os
import git
import subprocess
class GitManager:
def __init__(self, repo_path):
self.repo_path = repo_path
self.repo = git.Repo(repo_path)
def clone_repo(self, repo_url, destination):
git.Repo.clone_from(repo_url, destination)
print(f'Repo cloned to {destination}')
def get_status(self):
status = self.repo.git.status()
print(status)
def commit_changes(self, message):
self.repo.git.add(all=True)
self.repo.index.commit(message)
print('Changes committed')
def pull_changes(self):
subprocess.run(['git', 'pull'], cwd=self.repo_path)
print('Changes pulled from remote')
def push_changes(self):
subprocess.run(['git', 'push'], cwd=self.repo_path)
print('Changes pushed to remote')
使用示例
if __name__ == '__main__':
manager = GitManager('/path/to/repo')
manager.get_status()
manager.commit_changes('Update files')
manager.push_changes()
在这个示例中,GitManager
类封装了常见的Git操作,包括克隆、获取状态、提交更改、拉取和推送更改。这是一个简单的开始,可以根据需要进行扩展和修改。
通过以上介绍,您可以选择最适合您需求的方法在Python中使用Git。无论是GitPython库、os模块还是subprocess模块,它们都提供了强大的功能来管理Git仓库。根据项目的复杂性和特定需求,选择合适的工具将帮助您更高效地完成任务。
相关问答FAQs:
如何在Python项目中集成Git功能?
在Python项目中,可以使用GitPython库来集成Git功能。该库提供了简单的接口来执行常见的Git操作,比如克隆、提交和推送。首先,确保安装了GitPython,可以通过运行pip install GitPython
命令完成。接下来,可以使用以下代码示例来执行Git操作:
import git
# 克隆一个远程仓库
repo = git.Repo.clone_from('https://github.com/username/repo.git', 'local_repo')
# 提交更改
repo.git.add('your_file.txt')
repo.index.commit('Commit message')
# 推送更改
repo.git.push()
可以在Python中使用哪些Git命令?
在Python中,可以通过GitPython库执行多种Git命令,包括但不限于:clone
、add
、commit
、push
、pull
、checkout
、branch
等。每个命令都对应相应的库方法,能够帮助开发者轻松管理Git仓库。通过文档可以找到具体的用法和示例代码,便于快速上手。
在Python中使用Git时,如何处理冲突?
处理Git冲突时,可以通过GitPython库的Repo
对象来查看当前状态,并识别发生冲突的文件。可以使用repo.git.status()
查看状态,接着通过手动解决冲突后,使用repo.git.add()
和repo.index.commit()
来完成合并。确保在解决完所有冲突后再推送更改,以免影响其他协作者的工作。
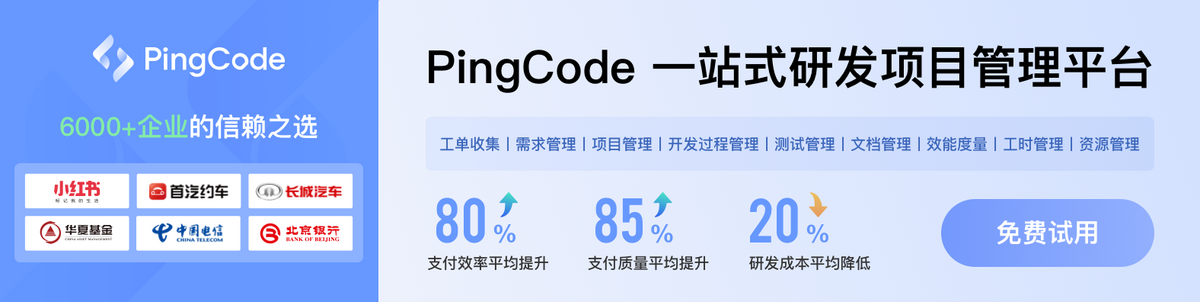