在Python中删除空行可以通过多种方法来实现,常见的方法包括使用字符串方法、列表解析以及正则表达式等。可以使用字符串的splitlines()方法结合列表解析、使用正则表达式去匹配空行、使用文件处理逐行读取并写回非空行的方式来删除空行。接下来,我们详细介绍其中一种方法:使用正则表达式匹配空行并删除。
使用正则表达式可以非常高效地处理文本数据。正则表达式提供了一种强大的方式来搜索和操作复杂的文本模式。在Python中,可以使用re
模块来处理正则表达式。下面是一个简单的示例,演示如何使用正则表达式删除文本中的空行。
import re
def remove_blank_lines(text):
# 使用正则表达式去匹配空行并删除
clean_text = re.sub(r'\n\s*\n', '\n', text)
return clean_text
示例文本
text = """This is a line with text.
This is another line with text.
And this is yet another line.
"""
删除空行
clean_text = remove_blank_lines(text)
print(clean_text)
在这个示例中,re.sub(r'\n\s*\n', '\n', text)
使用正则表达式r'\n\s*\n'
来匹配一个或多个空行,并将其替换为单个换行符,从而删除空行。
一、使用字符串方法删除空行
在处理字符串时,可以使用Python的字符串方法来删除空行。通常,我们可以通过splitlines()方法将字符串分割成行,然后使用列表解析去除空行。
-
使用splitlines()方法和列表解析
使用splitlines()方法可以将文本分割为行,并且不包含换行符。然后通过列表解析过滤掉空行。
def remove_blank_lines_with_splitlines(text):
lines = text.splitlines()
clean_lines = [line for line in lines if line.strip() != '']
return '\n'.join(clean_lines)
示例文本
text = """This is a line with text.
This is another line with text.
And this is yet another line.
"""
删除空行
clean_text = remove_blank_lines_with_splitlines(text)
print(clean_text)
在这个示例中,通过列表解析
[line for line in lines if line.strip() != '']
来过滤掉空行。 -
使用split()方法和join()方法
split()方法可以用于分割字符串并去除空行,然后使用join()方法将其重新组合。
def remove_blank_lines_with_split(text):
lines = text.split('\n')
clean_lines = [line for line in lines if line.strip() != '']
return '\n'.join(clean_lines)
示例文本
text = """This is a line with text.
This is another line with text.
And this is yet another line.
"""
删除空行
clean_text = remove_blank_lines_with_split(text)
print(clean_text)
在这个示例中,
split('\n')
用于分割文本,join()
用于重新组合非空行。
二、使用文件处理逐行读取
在处理文件时,可以通过逐行读取文件内容,并将非空行写入新文件的方法来删除空行。
-
读取文件,去除空行后写入新文件
可以通过逐行读取文件内容,过滤掉空行后再写入新文件。
def remove_blank_lines_from_file(input_file, output_file):
with open(input_file, 'r') as infile, open(output_file, 'w') as outfile:
for line in infile:
if line.strip():
outfile.write(line)
输入文件路径和输出文件路径
input_file = 'input.txt'
output_file = 'output.txt'
删除空行
remove_blank_lines_from_file(input_file, output_file)
在这个示例中,通过逐行读取文件
input.txt
,并将非空行写入output.txt
。 -
使用上下文管理器
使用
with
语句可以更简洁地管理文件的打开和关闭。def remove_blank_lines_with_context_manager(input_file, output_file):
with open(input_file, 'r') as infile, open(output_file, 'w') as outfile:
clean_lines = (line for line in infile if line.strip())
outfile.writelines(clean_lines)
输入文件路径和输出文件路径
input_file = 'input.txt'
output_file = 'output.txt'
删除空行
remove_blank_lines_with_context_manager(input_file, output_file)
通过生成器表达式
(line for line in infile if line.strip())
来过滤掉空行,并使用outfile.writelines()
将结果写入文件。
三、使用正则表达式删除空行
正则表达式提供了强大的文本处理能力,可以高效地处理复杂的文本模式。
-
使用re模块
Python的
re
模块提供了正则表达式的支持,可以用于匹配和替换文本中的空行。import re
def remove_blank_lines_with_regex(text):
clean_text = re.sub(r'\n\s*\n', '\n', text)
return clean_text
示例文本
text = """This is a line with text.
This is another line with text.
And this is yet another line.
"""
删除空行
clean_text = remove_blank_lines_with_regex(text)
print(clean_text)
在这个示例中,正则表达式
r'\n\s*\n'
用于匹配一个或多个空行,并将其替换为单个换行符。 -
处理多种空行情况
可以调整正则表达式以处理多种空行情况,例如行首尾空格的空行。
def remove_varied_blank_lines_with_regex(text):
clean_text = re.sub(r'^\s*\n', '', text, flags=re.MULTILINE)
return clean_text
示例文本
text = """This is a line with text.
This is another line with text.
And this is yet another line.
"""
删除空行
clean_text = remove_varied_blank_lines_with_regex(text)
print(clean_text)
通过
flags=re.MULTILINE
选项,使正则表达式匹配每一行的行首。
四、结合多种方法提高效率
在实际应用中,可以结合多种方法来提高删除空行的效率,特别是在处理大文件或复杂文本时。
-
预处理和正则表达式结合
可以先使用简单的字符串方法进行预处理,然后再使用正则表达式进行更精细的匹配。
def combined_method_for_blank_lines(text):
lines = text.splitlines()
# 初步去除空行
lines = [line for line in lines if line.strip() != '']
# 使用正则表达式进行精细处理
clean_text = re.sub(r'\n\s*\n', '\n', '\n'.join(lines))
return clean_text
示例文本
text = """This is a line with text.
This is another line with text.
And this is yet another line.
"""
删除空行
clean_text = combined_method_for_blank_lines(text)
print(clean_text)
这种方法结合了字符串方法和正则表达式的优点,提高了处理效率。
-
自定义函数处理特定场景
在特定场景下,可以根据实际需求自定义函数以处理不同类型的空行。
def custom_blank_line_removal(text, preserve_single_blank=False):
if preserve_single_blank:
clean_text = re.sub(r'\n\s*\n\s*\n', '\n\n', text)
else:
clean_text = re.sub(r'\n\s*\n', '\n', text)
return clean_text
示例文本
text = """This is a line with text.
This is another line with text.
And this is yet another line.
"""
删除空行
clean_text = custom_blank_line_removal(text, preserve_single_blank=True)
print(clean_text)
在这个示例中,
preserve_single_blank=True
参数用于控制是否保留单个空行。
通过这些方法,您可以在Python中灵活地删除空行,根据不同的需求选择最合适的方法来处理文本数据。
相关问答FAQs:
在Python中,如何判断文本文件中的空行?
在处理文本文件时,可以通过读取每一行并使用strip()
方法来判断空行。strip()
方法会移除行首和行尾的空白字符(包括空格、制表符和换行符)。如果经过strip()
处理后,行为空字符串,则可以认为该行是空行。
如何使用Python读取文件并删除空行?
可以使用with open()
语句打开文件,读取每一行并将非空行存储到一个新的列表中。接着,使用writelines()
方法将非空行写入新的文件中。这样可以有效地删除原文件中的空行。
使用Python脚本删除字符串列表中的空行,应该怎么做?
可以使用列表推导式来快速过滤字符串列表中的空行。在列表推导式中,结合strip()
方法,可以生成一个新的列表,包含所有非空字符串,轻松实现空行的删除。
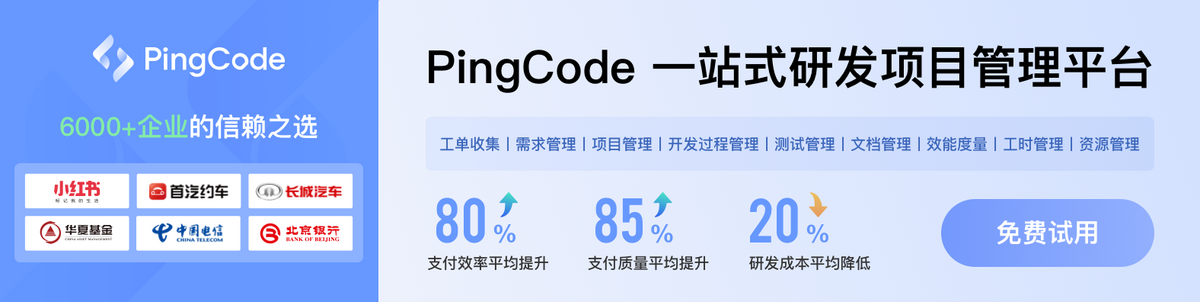