使用Python启动VCS(版本控制系统)通常可以通过调用命令行工具或使用相关库来实现。主要的方法包括使用subprocess模块来运行命令行指令、利用Python库与特定VCS系统的API进行交互、编写自动化脚本来简化重复性任务。在这些方法中,subprocess模块非常常用,因为它能够直接与系统命令行交互,执行VCS指令。
接下来,我们将详细讨论如何使用Python与不同的VCS系统进行交互,包括Git、SVN和Mercurial等,并提供相关的代码示例和注意事项。
一、使用SUBPROCESS模块
在Python中,subprocess模块是一个非常强大的工具,可以用于执行外部命令和与系统交互。它可以用于启动VCS命令行工具,如Git、SVN等。
1. 使用subprocess启动Git
Git是一个广泛使用的分布式版本控制系统。我们可以通过subprocess模块来运行Git命令。
import subprocess
克隆一个Git仓库
def clone_repository(repo_url, destination):
try:
subprocess.run(["git", "clone", repo_url, destination], check=True)
print(f"Repository cloned to {destination}")
except subprocess.CalledProcessError as e:
print(f"Error cloning repository: {e}")
示例用法
repo_url = "https://github.com/your/repo.git"
destination = "/path/to/destination"
clone_repository(repo_url, destination)
在这个例子中,我们使用subprocess.run
来执行Git的clone
命令,并设置check=True
以确保在命令失败时抛出异常。
2. 使用subprocess启动SVN
SVN(Subversion)是另一个常用的版本控制系统。类似于Git,我们可以使用subprocess模块来执行SVN命令。
import subprocess
检出一个SVN仓库
def checkout_svn(repo_url, destination):
try:
subprocess.run(["svn", "checkout", repo_url, destination], check=True)
print(f"Repository checked out to {destination}")
except subprocess.CalledProcessError as e:
print(f"Error checking out repository: {e}")
示例用法
repo_url = "http://svn.example.com/repo"
destination = "/path/to/destination"
checkout_svn(repo_url, destination)
3. 使用subprocess启动Mercurial
Mercurial是另一个分布式版本控制系统,subprocess模块同样适用于它。
import subprocess
克隆一个Mercurial仓库
def clone_hg(repo_url, destination):
try:
subprocess.run(["hg", "clone", repo_url, destination], check=True)
print(f"Repository cloned to {destination}")
except subprocess.CalledProcessError as e:
print(f"Error cloning repository: {e}")
示例用法
repo_url = "https://www.mercurial-scm.org/repo/hg"
destination = "/path/to/destination"
clone_hg(repo_url, destination)
二、使用特定VCS的Python库
除了直接调用命令行工具外,Python还提供了一些库,可以直接与特定的VCS系统进行交互,这些库通常提供更加友好的API。
1. 使用GitPython与Git交互
GitPython是一个用于与Git仓库交互的Python库。它提供了一个Pythonic的方式来操作Git仓库。
from git import Repo
克隆一个Git仓库
def clone_repository(repo_url, destination):
try:
Repo.clone_from(repo_url, destination)
print(f"Repository cloned to {destination}")
except Exception as e:
print(f"Error cloning repository: {e}")
示例用法
repo_url = "https://github.com/your/repo.git"
destination = "/path/to/destination"
clone_repository(repo_url, destination)
GitPython使得许多Git操作变得非常简单和直观,例如克隆、提交、推送等。
2. 使用Pysvn与SVN交互
Pysvn是一个用于与Subversion(SVN)交互的Python库。它提供了一个简单的API来进行SVN操作。
import pysvn
检出一个SVN仓库
def checkout_svn(repo_url, destination):
client = pysvn.Client()
try:
client.checkout(repo_url, destination)
print(f"Repository checked out to {destination}")
except pysvn.ClientError as e:
print(f"Error checking out repository: {e}")
示例用法
repo_url = "http://svn.example.com/repo"
destination = "/path/to/destination"
checkout_svn(repo_url, destination)
3. 使用HgAPI与Mercurial交互
HgAPI是一个用于与Mercurial仓库交互的Python库。它提供了一个简单的接口来进行Mercurial操作。
from hglib import client
克隆一个Mercurial仓库
def clone_hg(repo_url, destination):
try:
client.clone(repo_url, destination)
print(f"Repository cloned to {destination}")
except Exception as e:
print(f"Error cloning repository: {e}")
示例用法
repo_url = "https://www.mercurial-scm.org/repo/hg"
destination = "/path/to/destination"
clone_hg(repo_url, destination)
三、编写自动化脚本
为了简化重复性任务,我们可以编写自动化脚本,以便在不同的VCS系统中执行常见操作。
1. 自动化Git操作
可以编写脚本来简化Git的常见操作,例如提交、推送和拉取。
import subprocess
def git_commit_push(repo_path, commit_message):
try:
# 进入仓库目录
subprocess.run(["git", "-C", repo_path, "add", "."], check=True)
subprocess.run(["git", "-C", repo_path, "commit", "-m", commit_message], check=True)
subprocess.run(["git", "-C", repo_path, "push"], check=True)
print("Changes committed and pushed.")
except subprocess.CalledProcessError as e:
print(f"Error during Git operations: {e}")
示例用法
repo_path = "/path/to/repo"
commit_message = "Updated README file"
git_commit_push(repo_path, commit_message)
2. 自动化SVN操作
类似地,我们可以编写脚本来简化SVN的常见操作。
import subprocess
def svn_commit(repo_path, commit_message):
try:
# 进入仓库目录
subprocess.run(["svn", "add", "--force", "."], cwd=repo_path, check=True)
subprocess.run(["svn", "commit", "-m", commit_message], cwd=repo_path, check=True)
print("Changes committed.")
except subprocess.CalledProcessError as e:
print(f"Error during SVN operations: {e}")
示例用法
repo_path = "/path/to/repo"
commit_message = "Updated README file"
svn_commit(repo_path, commit_message)
3. 自动化Mercurial操作
对于Mercurial,我们可以编写脚本来简化常见的操作。
import subprocess
def hg_commit_push(repo_path, commit_message):
try:
# 进入仓库目录
subprocess.run(["hg", "add"], cwd=repo_path, check=True)
subprocess.run(["hg", "commit", "-m", commit_message], cwd=repo_path, check=True)
subprocess.run(["hg", "push"], cwd=repo_path, check=True)
print("Changes committed and pushed.")
except subprocess.CalledProcessError as e:
print(f"Error during Mercurial operations: {e}")
示例用法
repo_path = "/path/to/repo"
commit_message = "Updated README file"
hg_commit_push(repo_path, commit_message)
四、注意事项
在使用Python与VCS系统交互时,有一些重要的注意事项需要考虑。
1. 安全性
确保在脚本中不泄露敏感信息,例如用户名、密码或API密钥。可以使用环境变量或配置文件来安全地存储这些信息。
2. 错误处理
在执行外部命令时,可能会遇到各种错误,例如网络连接问题、权限问题等。应当在脚本中加入适当的错误处理机制,以便在出现问题时能够进行适当的处理。
3. 兼容性
不同的操作系统可能对命令行工具的支持有所不同。在编写跨平台脚本时,确保测试脚本在所有目标平台上均能正常运行。
4. 性能
对于大型仓库和复杂操作,执行时间可能会较长。可以考虑使用异步操作或多线程技术来提高性能。
五、总结
使用Python启动VCS系统不仅可以提高工作效率,还可以通过自动化脚本简化复杂的操作。无论是通过subprocess模块直接调用命令行工具,还是使用专门的Python库与VCS系统进行交互,Python都提供了丰富的工具和方法来实现这一目标。通过本文的详细介绍和示例,您应该能够更好地利用Python来管理和操作您的版本控制系统。
相关问答FAQs:
使用Python启动VCS需要哪些基本步骤?
要使用Python启动版本控制系统(VCS),首先需要确保已在系统中安装相应的VCS工具,例如Git或Mercurial。接下来,可以使用Python的subprocess
模块来执行命令行指令。通过创建一个新的Python脚本,您可以调用VCS命令并处理其输出。此外,可以根据需要设置环境变量,以确保VCS正常运行。
Python能与哪些版本控制系统兼容?
Python与多种版本控制系统兼容,包括Git、Mercurial、Subversion等。每种系统都有相应的命令行工具,您可以通过Python脚本与之交互。特别是Git因其广泛应用而常被选择,您可以利用Python脚本进行自动化任务,例如提交代码、拉取更新等。
在Python中如何处理VCS命令的输出?
处理VCS命令的输出可以通过subprocess
模块来完成。使用subprocess.run()
或subprocess.Popen()
可以获取命令执行后的返回信息。您可以通过指定参数获取标准输出和错误信息,这样可以灵活地处理不同的情况,例如在执行失败时进行错误处理或记录日志。
是否可以通过Python脚本实现VCS的自动化操作?
完全可以。通过Python脚本,您可以实现版本控制系统的自动化操作。例如,您可以编写脚本定期拉取代码、自动提交更改、创建分支等。这种自动化不仅提高了工作效率,还可以减少人为错误,确保代码库的更新和维护更加顺畅。
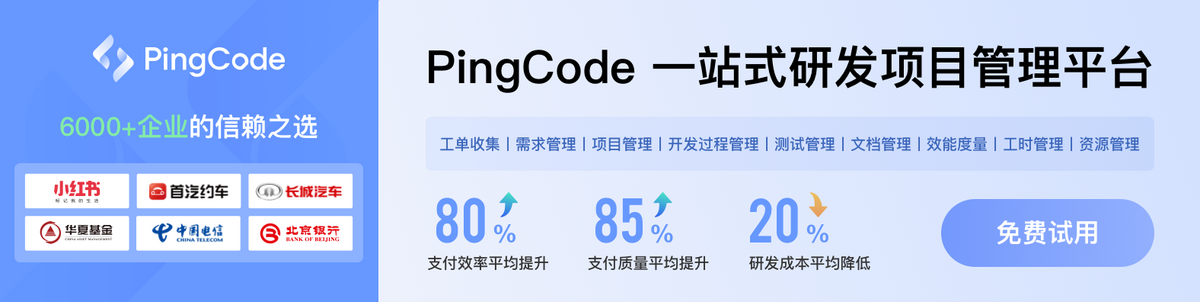