要计算梯形的面积,可以使用公式:(上底 + 下底) * 高 / 2。通过Python编程实现这一公式,可以通过定义一个函数,将上底、下底和高作为参数传入,返回计算结果。下面将详细介绍如何在Python中实现这一过程,以及一些关于梯形的基本知识和计算技巧。
一、梯形的基本知识
梯形是一种四边形,其特点是只有一组对边平行。平行的边称为梯形的上底和下底,而不平行的边被称为梯形的腰。梯形的面积计算公式为:
[ \text{面积} = \frac{(\text{上底} + \text{下底}) \times \text{高}}{2} ]
这个公式的原理是将梯形拆分成两个三角形和一个矩形,求和得到面积。
二、Python实现梯形面积计算
在Python中,可以通过定义函数来实现梯形面积的计算。以下是一个简单的函数示例:
def trapezoid_area(top_base, bottom_base, height):
"""
计算梯形的面积
:param top_base: 上底
:param bottom_base: 下底
:param height: 高
:return: 梯形的面积
"""
area = (top_base + bottom_base) * height / 2
return area
示例使用
假设上底为5,底边为10,高为7,可以通过调用函数来计算面积:
top_base = 5
bottom_base = 10
height = 7
area = trapezoid_area(top_base, bottom_base, height)
print(f"梯形的面积是: {area}")
以上代码会输出:“梯形的面积是: 52.5”。
三、用户输入与数据验证
为了使程序更加实用,可以接受用户输入,并验证输入的有效性。以下是一个增强版的程序:
def get_float_input(prompt):
while True:
try:
value = float(input(prompt))
if value <= 0:
raise ValueError("值必须是正数!")
return value
except ValueError as e:
print(f"无效输入: {e}")
def main():
print("计算梯形面积")
top_base = get_float_input("请输入上底:")
bottom_base = get_float_input("请输入下底:")
height = get_float_input("请输入高:")
area = trapezoid_area(top_base, bottom_base, height)
print(f"梯形的面积是: {area}")
if __name__ == "__main__":
main()
四、扩展计算
1、支持不同单位
在实际应用中,可能需要支持不同的单位(例如,厘米、米、英尺等)。这可以通过要求用户指定单位,并在输出中包含单位来实现。
def trapezoid_area_with_units(top_base, bottom_base, height, unit="cm"):
area = (top_base + bottom_base) * height / 2
return area, unit
def main_with_units():
print("计算梯形面积(支持单位)")
unit = input("请输入单位(例如 cm, m, ft):")
top_base = get_float_input(f"请输入上底 ({unit}):")
bottom_base = get_float_input(f"请输入下底 ({unit}):")
height = get_float_input(f"请输入高 ({unit}):")
area, unit = trapezoid_area_with_units(top_base, bottom_base, height, unit)
print(f"梯形的面积是: {area} {unit}²")
if __name__ == "__main__":
main_with_units()
2、可视化梯形
通过使用Python的matplotlib库,可以对梯形进行简单的可视化:
import matplotlib.pyplot as plt
def plot_trapezoid(top_base, bottom_base, height):
x = [0, (bottom_base - top_base) / 2, (bottom_base + top_base) / 2, bottom_base]
y = [0, height, height, 0]
plt.figure()
plt.plot(x + [x[0]], y + [y[0]], 'b-') # 绘制梯形
plt.fill(x + [x[0]], y + [y[0]], 'lightblue', alpha=0.5) # 填充颜色
plt.title('梯形')
plt.xlabel('宽度')
plt.ylabel('高度')
plt.grid(True)
plt.show()
示例使用
plot_trapezoid(5, 10, 7)
五、总结
通过学习如何在Python中计算梯形面积,我们不仅掌握了基本的数学公式,还了解了如何在程序中接收用户输入、进行数据验证以及进行简单的图形可视化。这种方法可以扩展到更多几何形状的面积和体积计算中,帮助我们解决实际生活中的问题。
相关问答FAQs:
如何用Python编写计算梯形面积的函数?
可以通过定义一个函数来计算梯形的面积。该函数需要两个底边的长度和高度作为参数。面积的计算公式为:面积 = (上底 + 下底) × 高 / 2。以下是一个示例代码:
def trapezoid_area(base1, base2, height):
return (base1 + base2) * height / 2
调用该函数时,只需传入相应的值即可。
在Python中如何处理用户输入的梯形参数?
可以使用input()
函数来获取用户输入的梯形参数。为了确保输入数据的有效性,可以使用try-except
结构处理可能的错误。以下是一个示例:
try:
base1 = float(input("请输入上底的长度:"))
base2 = float(input("请输入下底的长度:"))
height = float(input("请输入梯形的高度:"))
area = trapezoid_area(base1, base2, height)
print(f"梯形的面积是:{area}")
except ValueError:
print("请输入有效的数字。")
这种方式能够确保用户输入的数据类型正确。
是否可以使用Python的第三方库来计算梯形的面积?
是的,Python有许多第三方库可以简化数学计算,例如NumPy或SymPy。使用这些库可以更方便地进行复杂的数学运算。以下是一个使用NumPy的示例:
import numpy as np
def trapezoid_area_np(base1, base2, height):
return np.mean([base1, base2]) * height
area = trapezoid_area_np(5, 10, 4)
print(f"梯形的面积是:{area}")
借助NumPy,代码变得更加简洁和易读。
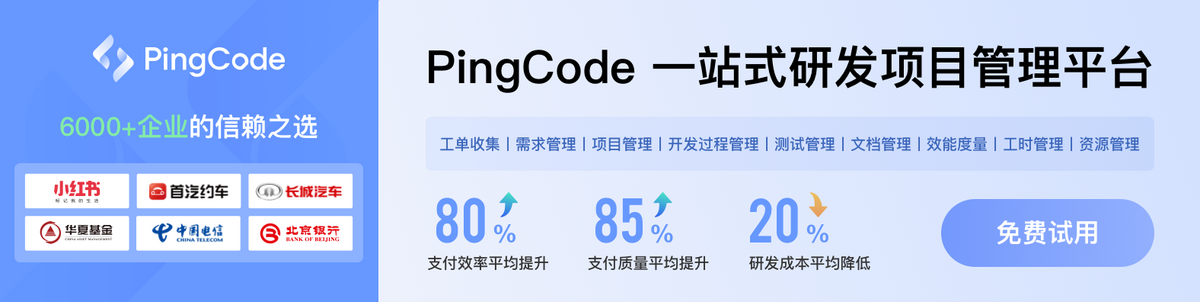