在Python中获取网页编码的方法主要有:使用requests库获取响应的编码信息、使用BeautifulSoup库解析网页并获取编码、以及通过chardet库进行编码检测。使用requests库是最直接的方法,因为它可以直接获取服务器返回的编码信息。
通过requests库获取网页编码非常简单,可以通过response.encoding
属性来获取。例如:
import requests
url = "http://example.com"
response = requests.get(url)
encoding = response.encoding
print(f"The encoding of the webpage is: {encoding}")
下面我们详细介绍如何在Python中通过不同的方法获取网页的编码。
一、使用REQUESTS库
requests库是Python中非常流行的HTTP请求库,它提供了简洁的API来发送HTTP请求。使用requests库获取网页编码的步骤如下:
- 发送HTTP请求:使用
requests.get()
方法发送请求并获取响应对象。 - 获取编码信息:通过
response.encoding
属性可以获取服务器返回的编码信息。如果服务器未指定编码,可以通过response.apparent_encoding
自动检测编码。
import requests
def get_webpage_encoding(url):
response = requests.get(url)
encoding = response.encoding if response.encoding else response.apparent_encoding
return encoding
url = "http://example.com"
encoding = get_webpage_encoding(url)
print(f"The encoding of the webpage is: {encoding}")
使用requests库非常方便,可以自动处理HTTP头中指定的编码信息,并且提供了自动检测编码的功能。
二、使用BEAUTIFULSOUP库
BeautifulSoup是一个用于解析HTML和XML文档的Python库,它提供了灵活的API来提取网页内容和结构。使用BeautifulSoup获取网页编码的步骤如下:
- 发送HTTP请求并获取网页内容:首先需要使用requests库获取网页内容。
- 解析网页并获取编码信息:使用BeautifulSoup解析网页,并通过
original_encoding
属性获取编码信息。
import requests
from bs4 import BeautifulSoup
def get_webpage_encoding_with_bs(url):
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
encoding = soup.original_encoding
return encoding
url = "http://example.com"
encoding = get_webpage_encoding_with_bs(url)
print(f"The encoding of the webpage is: {encoding}")
BeautifulSoup会根据网页的meta标签和文档声明来自动检测编码信息,并且在解析过程中会尝试纠正编码错误。
三、使用CHARDET库
chardet是一个用于检测字符编码的Python库,它可以根据文本的字节序列来推测编码。使用chardet获取网页编码的步骤如下:
- 发送HTTP请求并获取网页内容:首先需要使用requests库获取网页内容。
- 使用chardet检测编码:通过
chardet.detect()
方法检测编码信息。
import requests
import chardet
def get_webpage_encoding_with_chardet(url):
response = requests.get(url)
result = chardet.detect(response.content)
encoding = result['encoding']
return encoding
url = "http://example.com"
encoding = get_webpage_encoding_with_chardet(url)
print(f"The encoding of the webpage is: {encoding}")
chardet库可以用于检测没有明确指定编码的网页,对于某些非标准网页或编码信息不准确的网页特别有用。
四、编码检测的注意事项
在获取网页编码时,需要注意以下几点:
-
服务器返回的编码可能不准确:某些服务器返回的编码可能不正确,导致网页内容显示异常。因此,建议在获取编码后进行验证。
-
自动检测可能不完美:自动检测编码的方法在某些情况下可能不够准确,特别是在处理多种语言和复杂字符集时。因此,在可能的情况下,优先使用服务器提供的编码信息。
-
处理异常情况:在获取和解析网页内容时,可能会遇到异常情况,如网络错误、超时等。因此,需要在代码中加入异常处理逻辑,以提高程序的健壮性。
import requests
import chardet
def safe_get_webpage_encoding(url):
try:
response = requests.get(url, timeout=10)
response.raise_for_status()
encoding = response.encoding if response.encoding else chardet.detect(response.content)['encoding']
return encoding
except requests.exceptions.RequestException as e:
print(f"An error occurred: {e}")
return None
url = "http://example.com"
encoding = safe_get_webpage_encoding(url)
if encoding:
print(f"The encoding of the webpage is: {encoding}")
else:
print("Failed to get the encoding of the webpage.")
通过上述方法,可以有效地获取网页的编码信息,并确保程序在异常情况下能够正常运行。
相关问答FAQs:
如何判断一个网页的编码方式?
在Python中,可以使用requests
库获取网页的内容及其编码。通过response.encoding
属性,可以获取到网页的编码方式。若网页未明确指定编码,可以通过chardet
库进行自动检测,帮助您判断网页的实际编码。
使用Python获取网页编码的最佳实践是什么?
为了确保获取的网页编码准确,建议在发送请求时,使用requests.get()
方法获取响应后,检查response.encoding
属性。如果编码不正确,可以使用response.content
与chardet
库结合来进行进一步的分析,从而确保数据的准确性和完整性。
Python中如何处理不同编码的网页内容?
在处理不同编码的网页内容时,可以先获取网页的编码方式,然后使用response.content.decode(encoding)
方法将字节流解码为字符串。确保使用正确的编码格式解码,可以避免出现乱码问题,从而顺利提取网页信息。
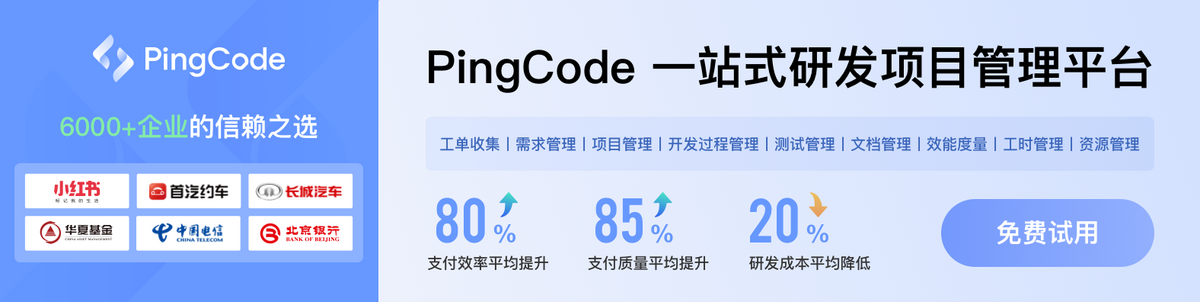