在Python中,删除文件主要通过os
模块和pathlib
模块实现,os.remove()
、os.unlink()
、pathlib.Path.unlink()
是常用方法。 os.remove()
和os.unlink()
基本相同,它们用于删除文件而不是目录。pathlib.Path.unlink()
是面向对象的方法,提供更现代化的文件操作方式。在使用这些方法时,确保文件存在以避免出现错误。下面将详细介绍这些方法及其使用场景。
一、使用OS模块删除文件
os
模块是Python内置的一个模块,提供了与操作系统进行交互的多种功能,其中包括删除文件的功能。主要使用os.remove()
和os.unlink()
方法,这两个方法在功能上是等价的。
1. os.remove()
方法
os.remove()
是用来删除文件的一个简单方法。其用法如下:
import os
删除文件
file_path = "example.txt"
if os.path.exists(file_path):
os.remove(file_path)
print(f"{file_path} has been deleted.")
else:
print(f"{file_path} does not exist.")
注意事项:
- 使用
os.remove()
之前,建议使用os.path.exists()
检查文件是否存在,以避免引发FileNotFoundError
。 - 该方法仅适用于文件,不能用于删除目录。如果需要删除目录,应使用
os.rmdir()
或shutil.rmtree()
。
2. os.unlink()
方法
os.unlink()
与os.remove()
功能相同,通常用于强调符号链接的删除,但在文件删除方面,两者没有实际区别。
import os
删除文件
file_path = "example.txt"
if os.path.exists(file_path):
os.unlink(file_path)
print(f"{file_path} has been deleted.")
else:
print(f"{file_path} does not exist.")
使用场景:
- 当需要删除符号链接文件时,
os.unlink()
更加语义化,但删除普通文件时无区别。
二、使用Pathlib模块删除文件
pathlib
模块是Python 3.4引入的,用于提供面向对象的文件系统路径操作。在删除文件时,Path.unlink()
方法被广泛使用。
1. Path.unlink()
方法
pathlib.Path.unlink()
提供了一种更现代和清晰的文件删除方式:
from pathlib import Path
删除文件
file_path = Path("example.txt")
if file_path.exists():
file_path.unlink()
print(f"{file_path} has been deleted.")
else:
print(f"{file_path} does not exist.")
优点:
Path
对象提供了更丰富的路径操作功能,可以很方便地进行路径组合、文件属性检查等。- 面向对象的语法使代码更具可读性和维护性。
三、错误处理与安全性
1. 错误处理
在删除文件的过程中,可能会遇到多种错误,例如文件不存在、权限不足等。因此,使用try-except
块进行错误处理是一个好习惯。
import os
file_path = "example.txt"
try:
os.remove(file_path)
print(f"{file_path} has been deleted.")
except FileNotFoundError:
print(f"Error: {file_path} does not exist.")
except PermissionError:
print(f"Error: You do not have permission to delete {file_path}.")
except Exception as e:
print(f"An unexpected error occurred: {e}")
2. 安全性
删除文件是一个不可逆的操作,因此需要格外谨慎。在进行批量删除操作时,建议先打印出将要删除的文件列表,确认后再执行删除操作。
四、删除目录的方法
如果需要删除的是目录而不是文件,os.remove()
和os.unlink()
方法是不适用的。对于目录,通常使用以下方法:
1. os.rmdir()
方法
用于删除空目录:
import os
删除空目录
dir_path = "empty_directory"
try:
os.rmdir(dir_path)
print(f"{dir_path} has been deleted.")
except FileNotFoundError:
print(f"Error: {dir_path} does not exist.")
except OSError:
print(f"Error: {dir_path} is not empty or cannot be deleted.")
2. shutil.rmtree()
方法
用于递归删除目录及其所有内容:
import shutil
删除目录及其所有内容
dir_path = "directory_with_contents"
try:
shutil.rmtree(dir_path)
print(f"{dir_path} and all its contents have been deleted.")
except FileNotFoundError:
print(f"Error: {dir_path} does not exist.")
except Exception as e:
print(f"An unexpected error occurred: {e}")
注意: 使用shutil.rmtree()
时要特别小心,因为它会删除目录中的所有文件和子目录。
五、实际应用示例
在实际项目中,删除文件通常是清理临时文件、日志文件或用户生成的内容的一部分。下面是一个实际应用中的示例:
应用场景
假设有一个应用程序在运行过程中会生成一系列临时文件,应用程序结束后需要清理这些临时文件以释放空间。
import os
import tempfile
创建临时文件
temp_dir = tempfile.gettempdir()
temp_file = os.path.join(temp_dir, "temp_file.txt")
创建并写入临时文件
with open(temp_file, "w") as f:
f.write("This is a temporary file.")
删除临时文件
if os.path.exists(temp_file):
try:
os.remove(temp_file)
print(f"Temporary file {temp_file} has been deleted.")
except Exception as e:
print(f"Failed to delete temporary file {temp_file}: {e}")
else:
print(f"Temporary file {temp_file} does not exist.")
批量删除
如果需要删除多个文件,可以使用循环:
import os
文件列表
files_to_delete = ["file1.txt", "file2.txt", "file3.txt"]
for file_name in files_to_delete:
if os.path.exists(file_name):
try:
os.remove(file_name)
print(f"{file_name} has been deleted.")
except Exception as e:
print(f"Failed to delete {file_name}: {e}")
else:
print(f"{file_name} does not exist.")
通过以上方法,Python提供了灵活多样的文件删除方式,适用于不同的应用场景。确保在删除文件前进行必要的检查和错误处理,以提高程序的可靠性和安全性。
相关问答FAQs:
在Python中使用哪个模块可以删除文件?
在Python中,可以使用内置的os
模块或pathlib
模块来删除文件。os
模块提供了os.remove()
函数,而pathlib
模块则提供了Path.unlink()
方法。这两种方法都可以有效地删除指定路径下的文件。
删除文件时需要注意哪些事项?
在删除文件时,确保文件路径正确,避免误删重要文件。此外,删除操作是不可逆的,因此最好在删除之前备份重要数据。使用os.path.exists()
函数可以先检查文件是否存在,以避免引发错误。
如何处理删除文件时可能出现的异常?
在删除文件的过程中,可能会遇到权限问题或文件不存在等异常。使用try...except
语句可以捕获这些异常,确保程序不会因错误而崩溃。例如,可以捕获FileNotFoundError
和PermissionError
,并给予用户友好的提示信息。
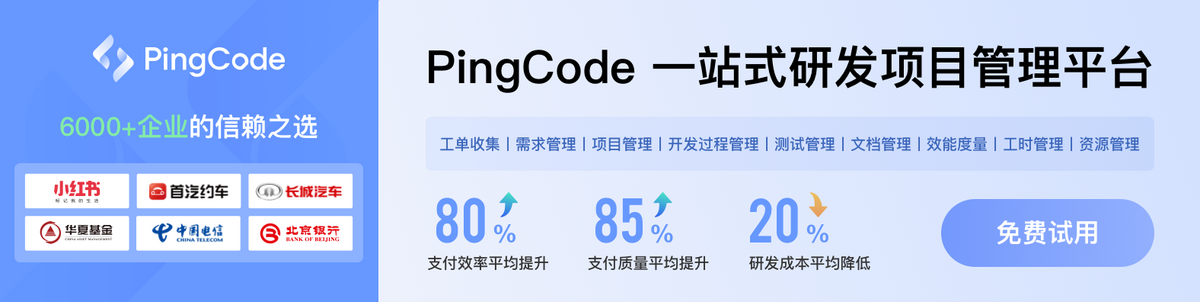