Python读取多个文件的方式有多种,常用的方法包括使用os模块、glob模块和pandas库。对于初学者来说,使用os和glob模块读取文件名列表,然后逐个读取文件内容是最为直观的方法。而对于数据分析任务,pandas库提供了更为简洁的解决方案,尤其适用于读取CSV文件的场景。以下详细介绍这几种方法。
一、使用OS模块读取多个文件
os模块是Python内置的标准库,提供了与操作系统进行交互的功能。其中,os.listdir()
函数可以列出指定目录下的所有文件名。
1.1 列出目录中的文件
首先,我们需要获取要读取的文件所在目录中所有文件的文件名。使用os.listdir()
可以实现这一目的:
import os
指定文件目录
directory = '/path/to/directory'
获取目录中的所有文件名
files = os.listdir(directory)
print(files)
1.2 读取文件内容
接下来,我们可以使用open()
函数逐个读取文件的内容:
for file_name in files:
file_path = os.path.join(directory, file_name)
with open(file_path, 'r') as file:
content = file.read()
print(content)
这里需要注意的是,open()
函数打开文件时,建议使用with
语句,以确保文件会在使用后正确关闭。
二、使用GLOB模块读取多个文件
glob模块提供了文件模式匹配的功能,可以通过模式匹配来获取特定类型的文件列表。
2.1 使用GLOB获取文件列表
glob模块的glob.glob()
函数可以通过通配符匹配获取文件列表:
import glob
使用通配符获取所有txt文件
file_paths = glob.glob('/path/to/directory/*.txt')
print(file_paths)
2.2 读取文件内容
获取文件路径列表后,同样可以使用open()
函数逐个读取文件内容:
for file_path in file_paths:
with open(file_path, 'r') as file:
content = file.read()
print(content)
三、使用PANDAS读取多个CSV文件
pandas库是Python中常用的数据分析工具,提供了强大的数据读取和处理功能。对于CSV文件,可以使用pandas.read_csv()
函数读取。
3.1 利用PANDAS读取CSV文件
pandas的read_csv()
函数可以直接读取CSV文件并转换为DataFrame对象:
import pandas as pd
import glob
获取所有CSV文件路径
csv_files = glob.glob('/path/to/directory/*.csv')
读取所有CSV文件并存储在一个列表中
dataframes = [pd.read_csv(file) for file in csv_files]
3.2 合并多个DataFrame
如果需要将多个CSV文件的数据合并在一起,可以使用pandas.concat()
函数:
# 合并所有DataFrame
merged_df = pd.concat(dataframes, ignore_index=True)
print(merged_df)
四、使用迭代器和生成器提高效率
在读取大量文件时,使用迭代器和生成器可以有效提高内存使用效率。
4.1 使用生成器逐个读取文件
通过生成器函数,可以逐个读取文件而不一次性将所有内容加载到内存中:
def file_reader(file_paths):
for file_path in file_paths:
with open(file_path, 'r') as file:
yield file.read()
使用生成器读取文件内容
file_contents = file_reader(file_paths)
for content in file_contents:
print(content)
五、错误处理与日志记录
在读取多个文件时,可能会出现文件不存在或无法读取的情况,因此需要进行错误处理和日志记录。
5.1 错误处理
可以使用try-except
块来捕获并处理可能的异常:
for file_path in file_paths:
try:
with open(file_path, 'r') as file:
content = file.read()
print(content)
except FileNotFoundError:
print(f"File {file_path} not found.")
except IOError:
print(f"Error reading file {file_path}.")
5.2 日志记录
使用Python的logging
模块记录日志信息,便于调试和问题定位:
import logging
logging.basicConfig(filename='file_read.log', level=logging.INFO)
for file_path in file_paths:
try:
with open(file_path, 'r') as file:
content = file.read()
logging.info(f"Successfully read {file_path}")
except Exception as e:
logging.error(f"Error reading {file_path}: {e}")
通过以上几种方法和策略,可以有效地读取和处理多个文件,结合具体的应用场景选择合适的方案,能够大大提高开发效率和代码的鲁棒性。
相关问答FAQs:
如何使用Python读取多个文件的内容?
在Python中,可以使用os
模块和glob
模块来读取多个文件的内容。首先,利用os.listdir()
获取目录下的所有文件名,或者使用glob.glob()
根据特定模式匹配文件。接下来,使用open()
函数逐个打开文件并读取其内容。示例代码如下:
import os
# 指定文件夹路径
folder_path = 'path/to/your/files'
# 遍历文件夹中的所有文件
for filename in os.listdir(folder_path):
if filename.endswith('.txt'): # 只读取txt文件
with open(os.path.join(folder_path, filename), 'r') as file:
content = file.read()
print(content) # 处理文件内容
如何处理读取多个文件时可能出现的异常?
在读取多个文件时,可能会遇到文件不存在或权限不足等异常。使用try...except
语句可以有效地捕获这些异常并进行处理。例如,可以在打开文件时添加异常处理逻辑,确保程序不会因为个别文件的问题而中断。以下是一个示例:
import os
folder_path = 'path/to/your/files'
for filename in os.listdir(folder_path):
if filename.endswith('.txt'):
try:
with open(os.path.join(folder_path, filename), 'r') as file:
content = file.read()
print(content)
except FileNotFoundError:
print(f"文件 {filename} 未找到")
except PermissionError:
print(f"没有权限读取文件 {filename}")
如何将多个文件的内容合并到一个文件中?
若希望将多个文件的内容合并为一个文件,可以在读取每个文件时,将其内容写入新的文件中。使用open()
函数以写入模式打开目标文件,并在读取每个源文件时,将内容逐步写入目标文件。示例代码如下:
import os
folder_path = 'path/to/your/files'
output_file = 'merged_file.txt'
with open(output_file, 'w') as outfile: # 创建或覆盖输出文件
for filename in os.listdir(folder_path):
if filename.endswith('.txt'):
with open(os.path.join(folder_path, filename), 'r') as infile:
content = infile.read()
outfile.write(content + "\n") # 添加换行符以分隔文件内容
通过上述方法,用户可以高效地读取、处理和合并多个文件的内容。
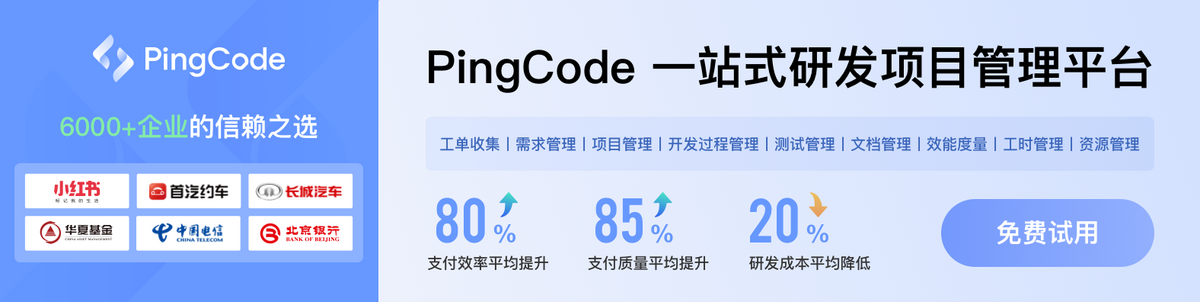