Python访问C类的方式主要有以下几种:使用C扩展模块、通过Cython、使用ctypes库。其中,使用C扩展模块是通过编写Python C API来实现的,适用于需要高性能和复杂交互的场景;Cython是一种将Python代码转换为C代码的工具,适合简化C和Python的交互;而ctypes库可以用来直接调用动态链接库中的函数,适合简单的C函数调用。
下面我将详细介绍这几种方法,并提供相关的示例和使用场景。
一、使用C扩展模块
C扩展模块是指通过编写C代码来扩展Python的功能。通过这种方式,可以直接在Python中调用C代码,从而实现更高的性能。这个方法需要编写C代码并使用Python C API。
1. 编写C代码
首先,需要编写一个C语言文件,例如example.c
。假设我们在C语言中有一个简单的类结构:
#include <Python.h>
typedef struct {
int value;
} Example;
static Example* Example_new(int value) {
Example* self = (Example*)malloc(sizeof(Example));
if (!self) return NULL;
self->value = value;
return self;
}
static void Example_del(Example* self) {
free(self);
}
static int Example_get_value(Example* self) {
return self->value;
}
// Add other C functions as necessary
2. 编写Python接口
接下来,我们需要编写一个Python接口文件,例如examplemodule.c
,用于将C代码暴露给Python:
#include <Python.h>
#include "example.h"
// Wrapper function for creating a new Example
static PyObject* py_example_new(PyObject* self, PyObject* args) {
int value;
if (!PyArg_ParseTuple(args, "i", &value)) {
return NULL;
}
Example* example = Example_new(value);
return PyCapsule_New(example, "Example", NULL);
}
// Wrapper function for getting the value
static PyObject* py_example_get_value(PyObject* self, PyObject* args) {
PyObject* capsule;
if (!PyArg_ParseTuple(args, "O", &capsule)) {
return NULL;
}
Example* example = (Example*)PyCapsule_GetPointer(capsule, "Example");
return Py_BuildValue("i", Example_get_value(example));
}
// Module method table
static PyMethodDef ExampleMethods[] = {
{"new", py_example_new, METH_VARARGS, "Create a new Example"},
{"get_value", py_example_get_value, METH_VARARGS, "Get the value"},
{NULL, NULL, 0, NULL}
};
// Module definition
static struct PyModuleDef examplemodule = {
PyModuleDef_HEAD_INIT,
"example",
NULL,
-1,
ExampleMethods
};
// Module initialization function
PyMODINIT_FUNC PyInit_example(void) {
return PyModule_Create(&examplemodule);
}
3. 编译和使用模块
使用setup.py
编译这个模块:
from setuptools import setup, Extension
example_module = Extension(
'example',
sources=['examplemodule.c'],
)
setup(
name='example',
version='1.0',
description='Example module written in C',
ext_modules=[example_module],
)
运行命令python setup.py build_ext --inplace
来编译模块。然后可以在Python中使用这个模块:
import example
ex = example.new(10)
value = example.get_value(ex)
print(value) # 输出: 10
二、通过Cython
Cython是一种将Python代码转换为C代码的工具。它可以很容易地将Python代码和C代码混合在一起,从而提高性能。
1. 编写Cython代码
首先,创建一个.pyx
文件,例如example.pyx
:
cdef class Example:
cdef int value
def __init__(self, int value):
self.value = value
def get_value(self):
return self.value
2. 编写setup.py
然后,编写一个setup.py
文件来编译Cython代码:
from setuptools import setup
from Cython.Build import cythonize
setup(
ext_modules=cythonize("example.pyx"),
)
运行命令python setup.py build_ext --inplace
来编译模块。然后可以在Python中使用这个模块:
from example import Example
ex = Example(10)
value = ex.get_value()
print(value) # 输出: 10
三、使用ctypes库
ctypes
是Python的一个内置库,它允许Python代码调用C语言动态链接库中的函数。适合于简单的C函数调用。
1. 编写C代码并编译为动态库
首先,编写一个C语言文件,例如example.c
,然后将其编译为动态链接库:
#include <stdio.h>
typedef struct {
int value;
} Example;
Example* Example_new(int value) {
Example* self = (Example*)malloc(sizeof(Example));
if (!self) return NULL;
self->value = value;
return self;
}
void Example_del(Example* self) {
free(self);
}
int Example_get_value(Example* self) {
return self->value;
}
编译为动态库(以Linux为例):
gcc -shared -o libexample.so -fPIC example.c
2. 使用ctypes调用
在Python中使用ctypes
来加载这个库并调用其中的函数:
import ctypes
Load the shared library
example_lib = ctypes.CDLL('./libexample.so')
Define the argument and return types for the C functions
example_lib.Example_new.argtypes = [ctypes.c_int]
example_lib.Example_new.restype = ctypes.POINTER(ctypes.c_void_p)
example_lib.Example_get_value.argtypes = [ctypes.POINTER(ctypes.c_void_p)]
example_lib.Example_get_value.restype = ctypes.c_int
Create an instance of Example
ex = example_lib.Example_new(10)
Get the value
value = example_lib.Example_get_value(ex)
print(value) # 输出: 10
Clean up
example_lib.Example_del(ex)
总结
通过以上三种方式,Python可以方便地访问和调用C语言的类和函数。使用C扩展模块和Cython可以更高效地集成复杂的C代码,而ctypes更适合快速调用简单的C函数。根据项目需求选择合适的方法,可以在Python中实现对C语言代码的高效访问和使用。
相关问答FAQs:
如何在Python中创建和使用C语言编写的类?
在Python中访问C语言类的常用方式是通过Python的C扩展。您需要使用Python的C API来创建扩展模块,定义C类,并在Python中调用它们。首先,您需要编写C代码并使用PyObject
结构体进行类的定义和方法的实现。编译后,您可以在Python中导入这个模块,并像使用普通Python类一样使用C类。
在Python中调用C类的方法需要注意哪些事项?
调用C类的方法时,确保正确管理内存,避免内存泄漏。C语言中的指针和内存管理与Python大相径庭,因此在C代码中,务必使用Py_DECREF
和Py_INCREF
来管理对象引用计数。此外,确保传递给C方法的数据类型与C代码中定义的类型相匹配,以避免运行时错误。
是否可以使用其他工具来简化Python与C类之间的交互?
确实可以,使用像Cython、SWIG或Pybind11等工具,可以大大简化Python与C语言的交互。这些工具提供了更高层次的抽象,允许您更轻松地定义接口和处理数据类型转换,减少手动编写C扩展的工作量。通过这些工具,可以快速将C类和函数暴露给Python,增强开发效率。
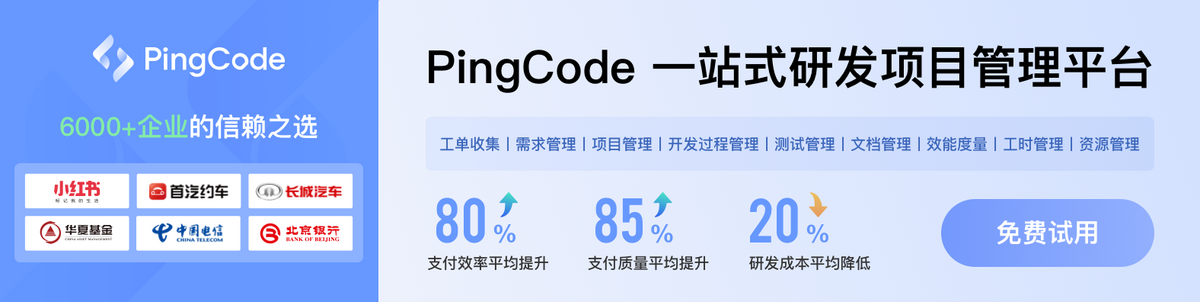