在Python中判断蓝牙连接可以通过使用蓝牙库如PyBluez、检查设备连接状态、轮询连接设备等方式。其中,PyBluez是一个常用的库,适用于在Python中进行蓝牙通信。通过该库,你可以扫描附近的蓝牙设备、创建连接、发送和接收数据等。下面我们将详细介绍这些方法,并为您提供一些代码示例。
一、使用PyBluez库进行蓝牙连接判断
1、安装PyBluez
要在Python中使用PyBluez库,首先需要安装它。可以通过以下命令安装:
pip install pybluez
PyBluez提供了对蓝牙协议的访问,可以帮助我们扫描附近的蓝牙设备以及创建蓝牙连接。
2、扫描蓝牙设备
使用PyBluez库,可以通过以下代码扫描附近的蓝牙设备:
import bluetooth
def scan_devices():
print("Scanning for bluetooth devices:")
devices = bluetooth.discover_devices(duration=8, lookup_names=True, flush_cache=True, lookup_class=False)
for addr, name in devices:
print(f"Device: {name}, Address: {addr}")
return devices
devices = scan_devices()
这段代码会扫描附近的蓝牙设备,并输出设备的名称和地址。你可以根据扫描结果来判断设备是否可连接。
3、判断蓝牙连接状态
在创建蓝牙连接后,可以通过异常处理来判断连接状态。例如:
import bluetooth
def connect_bluetooth_device(addr):
service_matches = bluetooth.find_service(address=addr)
if len(service_matches) == 0:
print("Could not find any services.")
return False
for service in service_matches:
port = service["port"]
name = service["name"]
host = service["host"]
print(f"Connecting to \"{name}\" on {host}")
sock = bluetooth.BluetoothSocket(bluetooth.RFCOMM)
try:
sock.connect((host, port))
print("Connection successful")
return True
except bluetooth.btcommon.BluetoothError as err:
print(f"Connection failed: {err}")
return False
finally:
sock.close()
addr = "00:11:22:33:44:55" # 替换为目标设备的蓝牙地址
is_connected = connect_bluetooth_device(addr)
这里通过尝试连接到服务并捕获可能的错误来判断连接是否成功。
二、检查设备连接状态
1、使用OS命令行工具
在某些操作系统中,可以使用命令行工具来检查蓝牙设备的连接状态。例如,在Linux上,可以使用hcitool
命令来检查设备连接状态:
hcitool con
该命令会列出当前连接的蓝牙设备。你可以在Python中调用该命令并解析其输出:
import subprocess
def check_connection():
try:
result = subprocess.run(["hcitool", "con"], capture_output=True, text=True)
print("Connected devices:")
print(result.stdout)
except subprocess.CalledProcessError as e:
print(f"Failed to check connection: {e}")
check_connection()
这种方法需要根据操作系统和可用工具进行调整。
2、使用D-Bus接口(适用于Linux系统)
在Linux系统中,可以使用D-Bus接口与蓝牙管理器交互,获取设备连接状态。以下是一个示例:
import dbus
def is_device_connected(device_address):
bus = dbus.SystemBus()
manager = dbus.Interface(bus.get_object("org.bluez", "/"), "org.freedesktop.DBus.ObjectManager")
objects = manager.GetManagedObjects()
for path, interfaces in objects.items():
if "org.bluez.Device1" in interfaces:
properties = interfaces["org.bluez.Device1"]
if properties["Address"] == device_address and properties["Connected"]:
return True
return False
device_address = "00:11:22:33:44:55" # 替换为目标设备的蓝牙地址
connected = is_device_connected(device_address)
print(f"Device connected: {connected}")
这种方法依赖于D-Bus,需要在具有D-Bus支持的Linux系统上运行。
三、轮询连接设备
1、定期扫描并检查设备
一种简单的方法是定期扫描蓝牙设备,并检查目标设备是否出现在扫描结果中。这种方法适用于不需要实时连接状态的场景。
import time
import bluetooth
def monitor_device(device_name):
while True:
devices = bluetooth.discover_devices(duration=8, lookup_names=True, flush_cache=True, lookup_class=False)
for addr, name in devices:
if name == device_name:
print(f"Device {device_name} is in range.")
return True
print(f"Device {device_name} not found. Retrying...")
time.sleep(5)
device_name = "My Bluetooth Device" # 替换为目标设备的名称
monitor_device(device_name)
2、使用事件驱动框架
对于需要实时响应的应用,可以使用事件驱动框架,例如PyGObject与BlueZ结合,来监听蓝牙设备的连接和断开事件。这种方法比较复杂,需要深入了解D-Bus和BlueZ的工作机制。
综上所述,判断Python中的蓝牙连接状态可以通过多种方法实现,包括使用PyBluez库进行连接尝试、通过OS命令检查连接状态,以及利用D-Bus接口获取设备状态等。选择合适的方法需要根据操作系统环境和具体应用场景来决定。
相关问答FAQs:
如何在Python中检查蓝牙设备的连接状态?
要检查蓝牙设备的连接状态,可以使用pybluez
库。通过该库,可以扫描周围的蓝牙设备并获取其连接状态。您需要首先安装pybluez
,然后使用相关的API来查询特定设备是否已连接。
有哪些Python库可以用来管理蓝牙连接?
Python中有几个库可以帮助您管理蓝牙连接,包括pybluez
、Bleak
和bluepy
。这些库各有特点,您可以根据自己的需求选择合适的库。例如,Bleak
支持BLE(蓝牙低能耗),适用于需要与现代设备通信的场景。
在Python中如何处理蓝牙连接失败的情况?
连接蓝牙设备时,可能会遇到失败的情况。可以通过捕获异常来处理这些错误。确保您的代码中包含错误处理机制,以便在连接失败时输出合适的错误信息或尝试重新连接。
如何优化Python蓝牙连接的性能?
优化蓝牙连接的性能可以通过多种方式实现,例如减少扫描时间、限制并发连接数以及使用高效的协议。使用合适的蓝牙库并合理配置参数,可以显著提高连接的稳定性和速度。
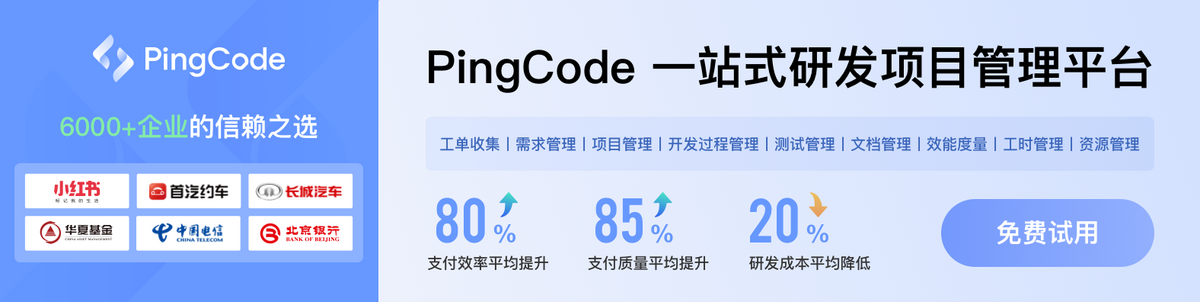