使用Python搜索文件可以通过多种方式实现,主要方法包括:os模块遍历文件系统、glob模块进行模式匹配、fnmatch模块进行文件名匹配。os模块提供了底层的文件操作功能、glob模块支持简单的通配符匹配、fnmatch模块允许复杂的模式匹配。本文将详细介绍这些方法的使用,并提供实际应用场景的示例代码。
一、OS模块遍历文件系统
os模块是Python中用于与操作系统进行交互的标准库,它提供了访问文件系统的基本方法。通过os模块,我们可以遍历目录,查找匹配条件的文件。
1. os.walk()方法
os.walk()是一个生成器,用于遍历目录树。它会生成目录路径、目录名和文件名的三元组。
import os
def search_files(directory, target_file):
for dirpath, dirnames, filenames in os.walk(directory):
if target_file in filenames:
print(f"File found: {os.path.join(dirpath, target_file)}")
search_files('/path/to/search', 'example.txt')
在这个例子中,search_files函数遍历指定目录及其子目录中的所有文件,并检查目标文件是否存在。
2. os.listdir()方法
os.listdir()列出指定目录中的所有文件和目录,但不递归子目录。
import os
def search_files(directory, target_file):
for filename in os.listdir(directory):
if filename == target_file:
print(f"File found: {os.path.join(directory, target_file)}")
search_files('/path/to/search', 'example.txt')
os.listdir()适用于仅需检查单一目录的情况。
二、GLOB模块进行模式匹配
glob模块提供了基于Unix风格路径名模式扩展的文件操作功能。它支持使用通配符进行文件搜索。
1. 使用glob.glob()方法
glob.glob()方法返回所有匹配的文件路径列表。
import glob
def search_files(pattern):
files = glob.glob(pattern)
for file in files:
print(f"File found: {file}")
search_files('/path/to/search//*.txt')
在这个例子中,我们使用通配符“”递归搜索所有子目录中的文本文件。
2. glob.iglob()方法
glob.iglob()与glob相似,但返回的是一个迭代器,适用于处理大量文件的情况。
import glob
def search_files(pattern):
for file in glob.iglob(pattern, recursive=True):
print(f"File found: {file}")
search_files('/path/to/search//*.txt')
使用glob.iglob()在处理大数据量时更为高效,因为它不一次性加载所有匹配文件的路径。
三、FNMATCH模块进行文件名匹配
fnmatch模块允许使用shell样式的通配符进行字符串匹配,适合用于过滤文件名。
1. 使用fnmatch.fnmatch()方法
fnmatch.fnmatch()用于匹配文件名与模式。
import os
import fnmatch
def search_files(directory, pattern):
for dirpath, dirnames, filenames in os.walk(directory):
for filename in filenames:
if fnmatch.fnmatch(filename, pattern):
print(f"File found: {os.path.join(dirpath, filename)}")
search_files('/path/to/search', '*.txt')
这个例子中,fnmatch.fnmatch()用于在遍历的文件名中匹配指定的模式。
四、综合应用与优化
在实际应用中,选择合适的方法取决于具体需求和应用场景。
1. 性能优化
当处理大量文件或大目录树时,性能可能成为瓶颈。以下是一些优化建议:
- 使用生成器(如os.walk()和glob.iglob())以减少内存占用。
- 在可能的情况下,通过限制搜索深度或过滤条件减少文件数。
- 考虑多线程或异步IO以提高并发性能。
2. 应用场景
- 日志分析:通过搜索特定模式的日志文件,自动化分析和汇总。
- 批量重命名:匹配特定文件,批量进行重命名操作。
- 文件备份:查找特定类型的文件并定期备份。
五、代码示例与实践
结合上述方法,以下是一个综合示例,展示了如何在Python中实现文件搜索功能。
import os
import fnmatch
import glob
def search_using_os(directory, target_file):
for dirpath, dirnames, filenames in os.walk(directory):
if target_file in filenames:
print(f"OS Search - File found: {os.path.join(dirpath, target_file)}")
def search_using_glob(pattern):
for file in glob.iglob(pattern, recursive=True):
print(f"GLOB Search - File found: {file}")
def search_using_fnmatch(directory, pattern):
for dirpath, dirnames, filenames in os.walk(directory):
for filename in filenames:
if fnmatch.fnmatch(filename, pattern):
print(f"FNMATCH Search - File found: {os.path.join(dirpath, filename)}")
Example usage
search_using_os('/path/to/search', 'example.txt')
search_using_glob('/path/to/search//*.txt')
search_using_fnmatch('/path/to/search', '*.txt')
六、总结
Python提供了多种搜索文件的方法,每种方法都有其独特的优势和适用场景。通过os模块、glob模块和fnmatch模块,我们可以灵活高效地实现文件搜索功能。在实际开发中,结合具体需求和性能考虑选择合适的方法,将帮助我们更好地完成任务。
相关问答FAQs:
如何在特定目录中使用Python搜索文件?
您可以使用Python的os
和fnmatch
模块来在特定目录中搜索文件。首先,使用os.walk()
遍历目录及其子目录,然后利用fnmatch.fnmatch()
来匹配您想要的文件名模式。例如,如果您想找到所有的.txt
文件,可以这样写代码:
import os
import fnmatch
def search_files(directory, pattern):
for dirpath, dirnames, filenames in os.walk(directory):
for filename in fnmatch.filter(filenames, pattern):
print(os.path.join(dirpath, filename))
search_files('/your/directory/path', '*.txt')
如何使用Python搜索文件内容而不仅仅是文件名?
若要搜索文件内容,可以使用Python的open()
函数来读取文件,并结合in
关键字检查特定字符串是否存在于文件中。您可以在遍历文件时,读取每个文件并进行匹配。例如:
def search_file_content(directory, keyword):
for dirpath, dirnames, filenames in os.walk(directory):
for filename in filenames:
file_path = os.path.join(dirpath, filename)
with open(file_path, 'r', encoding='utf-8', errors='ignore') as file:
if keyword in file.read():
print(f'Found "{keyword}" in: {file_path}')
search_file_content('/your/directory/path', 'search_term')
Python是否有第三方库来简化文件搜索?
是的,Python有许多第三方库可以简化文件搜索,例如pathlib
和glob
。pathlib
提供了面向对象的文件系统路径操作,而glob
可以使用通配符简单地查找符合条件的文件。例如,使用pathlib
查找所有.jpg
文件:
from pathlib import Path
def find_images(directory):
path = Path(directory)
for image in path.glob('*.jpg'):
print(image)
find_images('/your/directory/path')
这些库不仅使代码更加简洁,而且提高了可读性和维护性。
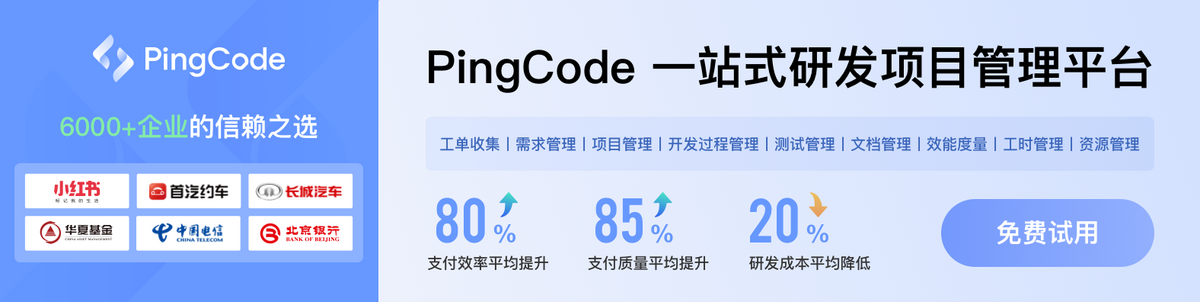