制作随机题库可以通过以下方法实现:使用Python内置的random
模块生成随机数、将题目存储在列表或字典中、从中随机选择题目。可以通过定义一个函数来实现题目的随机选择,并且结合用户输入进行交互。接下来,我们将详细讨论如何使用Python创建一个随机题库,以及如何通过编程实现题库的随机化和用户交互。
一、使用random
模块生成随机数
Python的random
模块提供了多种生成随机数的方法。在制作随机题库时,random.choice()
和random.sample()
是两个非常有用的函数。
-
random.choice()
函数random.choice()
用于从一个非空序列中随机选择一个元素。对于题库来说,可以用它来随机选择一道题。- 示例代码:
import random
questions = ["What is the capital of France?", "What is 2 + 2?", "What is the largest mammal?"]
random_question = random.choice(questions)
print(random_question)
- 在这个例子中,我们创建了一个包含三个问题的列表,然后使用
random.choice()
从中随机选择一个问题。
-
random.sample()
函数random.sample()
用于从一个序列中随机选择多个元素,返回一个指定长度的新列表。- 示例代码:
import random
questions = ["What is the capital of France?", "What is 2 + 2?", "What is the largest mammal?", "Who wrote 'Macbeth'?", "What is the speed of light?"]
random_questions = random.sample(questions, 3)
print(random_questions)
- 在这个例子中,我们使用
random.sample()
从题库中随机选择了三个问题。
二、将题目存储在数据结构中
为了更好地管理题库,可以使用Python的数据结构,如列表、字典、JSON文件等。
-
列表
- 列表适用于存储简单的题目集合,尤其是当题目不需要附加信息时。
- 示例代码:
questions = [
"What is the capital of France?",
"What is 2 + 2?",
"What is the largest mammal?"
]
-
字典
- 如果每个题目需要附加信息(如选项、答案、难度等级等),可以使用字典。
- 示例代码:
questions = {
1: {"question": "What is the capital of France?", "options": ["Paris", "London", "Rome"], "answer": "Paris"},
2: {"question": "What is 2 + 2?", "options": [3, 4, 5], "answer": 4},
3: {"question": "What is the largest mammal?", "options": ["Elephant", "Whale", "Giraffe"], "answer": "Whale"}
}
-
JSON文件
- 使用JSON文件存储题库可以方便地进行数据的读写和共享。
- 示例代码:
import json
questions = {
"1": {"question": "What is the capital of France?", "options": ["Paris", "London", "Rome"], "answer": "Paris"},
"2": {"question": "What is 2 + 2?", "options": [3, 4, 5], "answer": 4},
"3": {"question": "What is the largest mammal?", "options": ["Elephant", "Whale", "Giraffe"], "answer": "Whale"}
}
with open('questions.json', 'w') as json_file:
json.dump(questions, json_file)
三、从题库中随机选择题目
创建一个函数来实现从题库中随机选择题目的逻辑。这个函数可以根据不同的数据结构进行调整。
-
从列表中随机选择
import random
def get_random_question(questions):
return random.choice(questions)
questions = [
"What is the capital of France?",
"What is 2 + 2?",
"What is the largest mammal?"
]
print(get_random_question(questions))
-
从字典中随机选择
import random
def get_random_question(questions):
question_id = random.choice(list(questions.keys()))
return questions[question_id]
questions = {
1: {"question": "What is the capital of France?", "options": ["Paris", "London", "Rome"], "answer": "Paris"},
2: {"question": "What is 2 + 2?", "options": [3, 4, 5], "answer": 4},
3: {"question": "What is the largest mammal?", "options": ["Elephant", "Whale", "Giraffe"], "answer": "Whale"}
}
print(get_random_question(questions))
-
从JSON文件中随机选择
import json
import random
def load_questions(filename):
with open(filename, 'r') as json_file:
return json.load(json_file)
def get_random_question(questions):
question_id = random.choice(list(questions.keys()))
return questions[question_id]
questions = load_questions('questions.json')
print(get_random_question(questions))
四、实现用户交互
为了提升题库的实用性,可以加入用户交互部分,让用户回答问题。
-
交互式答题
import random
def get_random_question(questions):
question_id = random.choice(list(questions.keys()))
return question_id, questions[question_id]
def ask_question(question_data):
print(question_data["question"])
for idx, option in enumerate(question_data["options"], 1):
print(f"{idx}. {option}")
answer = int(input("Please choose the correct option number: "))
return answer
questions = {
1: {"question": "What is the capital of France?", "options": ["Paris", "London", "Rome"], "answer": "Paris"},
2: {"question": "What is 2 + 2?", "options": [3, 4, 5], "answer": 4},
3: {"question": "What is the largest mammal?", "options": ["Elephant", "Whale", "Giraffe"], "answer": "Whale"}
}
question_id, question_data = get_random_question(questions)
user_answer = ask_question(question_data)
if question_data["options"][user_answer - 1] == question_data["answer"]:
print("Correct!")
else:
print("Incorrect.")
-
提供反馈和统计
- 可以在用户回答后提供即时反馈,并统计用户的正确率。
import random
def get_random_question(questions):
question_id = random.choice(list(questions.keys()))
return question_id, questions[question_id]
def ask_question(question_data):
print(question_data["question"])
for idx, option in enumerate(question_data["options"], 1):
print(f"{idx}. {option}")
answer = int(input("Please choose the correct option number: "))
return answer
questions = {
1: {"question": "What is the capital of France?", "options": ["Paris", "London", "Rome"], "answer": "Paris"},
2: {"question": "What is 2 + 2?", "options": [3, 4, 5], "answer": 4},
3: {"question": "What is the largest mammal?", "options": ["Elephant", "Whale", "Giraffe"], "answer": "Whale"}
}
correct_answers = 0
total_questions = 5
for _ in range(total_questions):
question_id, question_data = get_random_question(questions)
user_answer = ask_question(question_data)
if question_data["options"][user_answer - 1] == question_data["answer"]:
print("Correct!")
correct_answers += 1
else:
print("Incorrect.")
print(f"You answered {correct_answers} out of {total_questions} questions correctly.")
通过以上步骤,您可以使用Python创建一个功能丰富的随机题库系统。这不仅可以用于教育目的,还可以用于面试准备、考试模拟以及其他需要题库的场景。无论是简单的列表还是复杂的JSON文件,Python都能提供强大的工具来实现这一目标。
相关问答FAQs:
如何在Python中生成随机题库?
在Python中,可以使用内置的random
模块来生成随机题库。首先,需要准备一个包含题目的列表或字典,然后利用random.choice()
或者random.sample()
方法从中随机选择题目。这样可以确保每次生成的题目都不相同,增加了题库的趣味性和挑战性。
我可以如何确保题库的多样性和覆盖面?
为了确保题库的多样性,可以从不同主题和难度等级中挑选题目。可以创建多个列表,每个列表代表一个主题,然后结合这些列表生成随机题目。此外,还可以定期更新题库,添加新题目,以保持内容的新鲜感和覆盖面。
如何将生成的随机题库保存到文件中?
将随机题库保存到文件中可以使用Python的文件操作功能。可以选择将题库保存为文本文件、CSV文件或JSON格式。通过使用with open()
语句,可以轻松地将生成的题目写入文件。这不仅方便后续查看,还可以方便地与他人分享。
如何为随机题库添加评分系统?
可以为随机题库添加简单的评分系统,通过跟踪用户的回答情况来实现。每次用户回答一个问题时,可以记录他们的回答是否正确,并为每个正确答案给予分数。这样不仅增加了互动性,还能帮助用户了解自己的学习进度。
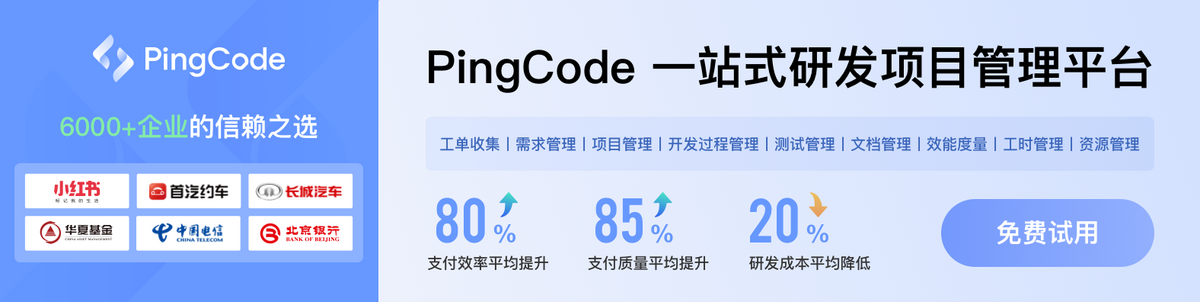