Python中转换编码的方法主要有:使用内置的encode()
和decode()
方法、利用codecs
模块、以及使用chardet
库自动检测编码。其中,encode()
和decode()
方法是最常用的,encode()
可以将字符串编码为指定编码格式的字节串,而decode()
则是将字节串解码为字符串。下面我们将详细讨论这些方法。
一、使用encode()
和decode()
方法
Python中的字符串是Unicode格式的,通常在处理文本数据时,我们需要将其转换为其他编码格式,比如UTF-8或GBK。encode()
方法可以帮助我们完成这一转换。
- 使用
encode()
方法
encode()
方法用于将字符串转换为指定编码格式的字节串。它的基本语法是:
str.encode(encoding='utf-8', errors='strict')
encoding
参数指定目标编码格式。errors
参数指定错误处理方案,常用的值包括'strict'
(抛出异常)、'ignore'
(忽略错误)、'replace'
(用替代字符代替)。
例如,将字符串转换为UTF-8编码:
original_str = "你好,世界"
encoded_bytes = original_str.encode('utf-8')
print(encoded_bytes)
- 使用
decode()
方法
decode()
方法用于将字节串转换回字符串。它的基本语法是:
bytes.decode(encoding='utf-8', errors='strict')
例如,将UTF-8编码的字节串解码为字符串:
decoded_str = encoded_bytes.decode('utf-8')
print(decoded_str)
二、利用codecs
模块
codecs
模块提供了更底层的编码和解码接口,可以用于文件的读写操作,支持多种编码格式。
- 打开文件时指定编码
codecs
模块可以在打开文件时指定编码格式,确保读写时的编码正确。
import codecs
写入文件时指定编码
with codecs.open('example.txt', 'w', encoding='utf-8') as f:
f.write("你好,世界")
读取文件时指定编码
with codecs.open('example.txt', 'r', encoding='utf-8') as f:
content = f.read()
print(content)
- 手动编码转换
codecs
模块也可以用于手动转换字符串的编码:
import codecs
original_str = "你好,世界"
将字符串转换为GBK编码的字节串
encoded_bytes = codecs.encode(original_str, 'gbk')
将GBK编码的字节串转换回Unicode字符串
decoded_str = codecs.decode(encoded_bytes, 'gbk')
print(decoded_str)
三、使用chardet
库自动检测编码
有时,我们可能不知道文本数据的编码格式,这时可以使用chardet
库自动检测。
- 安装
chardet
库
在使用chardet
之前,需要先安装它:
pip install chardet
- 使用
chardet
检测编码
chardet
可以自动检测字节串的编码格式,并返回检测结果。
import chardet
假设有一段未知编码的字节串
unknown_bytes = b'\xe4\xbd\xa0\xe5\xa5\xbd\xef\xbc\x8c\xe4\xb8\x96\xe7\x95\x8c'
使用chardet检测编码
result = chardet.detect(unknown_bytes)
encoding = result['encoding']
confidence = result['confidence']
print(f"Detected encoding: {encoding}, Confidence: {confidence}")
使用检测出的编码进行解码
decoded_str = unknown_bytes.decode(encoding)
print(decoded_str)
四、处理文本文件中的编码问题
在处理文本文件时,可能会遇到各种编码问题,以下是一些常见的处理策略。
- 确保文件读写时使用一致的编码
在读写文件时,务必指定相同的编码格式,以避免编码不一致导致的错误。
with open('example.txt', 'w', encoding='utf-8') as f:
f.write("你好,世界")
with open('example.txt', 'r', encoding='utf-8') as f:
content = f.read()
print(content)
- 处理编码错误
在处理文件时,如果遇到编码错误,可以通过指定错误处理策略来解决。
# 忽略编码错误
with open('example.txt', 'r', encoding='utf-8', errors='ignore') as f:
content = f.read()
用替代字符替换错误
with open('example.txt', 'r', encoding='utf-8', errors='replace') as f:
content = f.read()
- 批量转换文件编码
在处理大量文件时,可以编写脚本批量转换文件的编码格式。
import os
import codecs
def convert_file_encoding(src_path, dest_path, src_encoding='gbk', dest_encoding='utf-8'):
with codecs.open(src_path, 'r', encoding=src_encoding) as src_file:
content = src_file.read()
with codecs.open(dest_path, 'w', encoding=dest_encoding) as dest_file:
dest_file.write(content)
批量转换目录下的所有文件
src_directory = 'source_files'
dest_directory = 'converted_files'
for filename in os.listdir(src_directory):
src_file_path = os.path.join(src_directory, filename)
dest_file_path = os.path.join(dest_directory, filename)
convert_file_encoding(src_file_path, dest_file_path)
五、处理网络数据的编码问题
在处理网络数据时,编码问题也经常出现,特别是在处理非英文字符时。
- HTTP请求中的编码
在使用HTTP请求获取数据时,通常需要根据Content-Type
头部中的charset
指定编码进行解码。
import requests
response = requests.get('https://example.com')
content_type = response.headers['Content-Type']
从Content-Type中提取编码信息
encoding = 'utf-8' # 默认编码
if 'charset=' in content_type:
encoding = content_type.split('charset=')[-1]
使用提取的编码解码响应内容
decoded_content = response.content.decode(encoding)
print(decoded_content)
- 处理JSON数据中的编码
在处理JSON数据时,通常JSON库会自动处理编码问题,但在某些情况下可能需要手动指定编码。
import json
假设有一段JSON格式的字节串
json_bytes = b'{"message": "\xe4\xbd\xa0\xe5\xa5\xbd"}'
使用指定编码解码为字符串
json_str = json_bytes.decode('utf-8')
解析JSON字符串
data = json.loads(json_str)
print(data['message'])
六、编码转换的注意事项
在进行编码转换时,需要注意以下几点:
- 始终明确源数据的编码格式
在转换编码之前,必须明确源数据的编码格式,否则可能导致解码错误。
- 避免使用不支持的编码
在选择编码格式时,确保目标系统或应用程序支持该编码。
- 处理编码转换中的异常
在编码转换过程中,可能会出现异常,建议使用try-except
块进行捕获和处理。
try:
decoded_str = unknown_bytes.decode('utf-8')
except UnicodeDecodeError as e:
print(f"Decoding failed: {e}")
通过本文的详细介绍,相信您对Python中的编码转换有了更深入的了解和掌握。在实际应用中,根据具体需求选择合适的方法和策略,能够有效解决编码相关的问题。
相关问答FAQs:
如何在Python中查看当前字符串的编码格式?
在Python中,可以使用str.encode()
方法将字符串转换为字节流,从而查看其编码格式。可以通过chardet
库来检测字符串的编码。安装此库后,使用chardet.detect()
函数,可以获取字符串的编码信息。示例代码如下:
import chardet
text = "示例文本"
result = chardet.detect(text.encode())
print(result)
这将返回一个字典,其中包含字符串的编码格式和置信度。
在Python中如何将文件从一种编码转换为另一种编码?
可以使用Python的内置open()
函数结合encode()
和decode()
方法来实现文件编码的转换。打开文件时指定原编码,读取内容后再以目标编码写入新文件。示例代码如下:
with open('input_file.txt', 'r', encoding='utf-8') as infile:
content = infile.read()
with open('output_file.txt', 'w', encoding='gbk') as outfile:
outfile.write(content)
这段代码将UTF-8编码的文件转换为GBK编码。
如何处理Python中的Unicode编码错误?
在进行编码和解码时,可能会遇到Unicode编码错误。为了处理这些错误,可以使用errors
参数,例如ignore
、replace
或backslashreplace
。这将允许程序在遇到无法编码的字符时采取相应的处理策略。示例代码:
text = "示例文本"
encoded_text = text.encode('ascii', errors='replace') # 用?替代无法编码的字符
print(encoded_text)
根据需要选择适合的错误处理策略,可以有效避免程序崩溃。
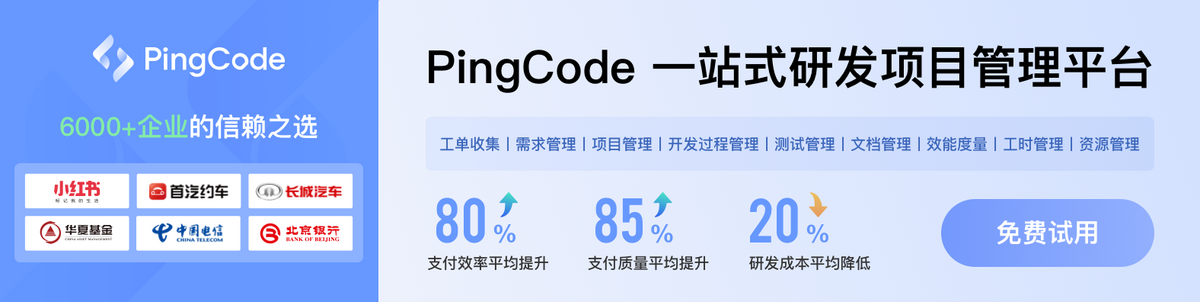