在Python中避免重复打印的方法包括:使用集合(set)存储已打印的数据、条件判断控制打印、使用日志模块控制输出。使用集合存储已打印的数据是一种常见且有效的方法,因为集合天然去重。例如,我们可以在每次打印之前,检查数据是否已存在于集合中,若不存在则打印并将其加入集合。下面将详细介绍这些方法。
一、使用集合(set)
在Python中,集合是一种无序且不重复的数据结构,这使得它成为存储已打印数据的理想选择。我们可以在每次打印前检查数据是否在集合中,如果不在则打印并添加到集合中。
printed_data = set()
def print_unique(data):
if data not in printed_data:
print(data)
printed_data.add(data)
在这个函数中,printed_data
集合用于存储已打印过的数据。print_unique
函数在打印数据前检查集合,如果数据不存在于集合中,则执行打印并将数据添加到集合中。
二、条件判断
条件判断是最常见的方法之一,通过逻辑判断控制是否执行打印操作。可以在打印操作前添加条件判断,确保只有满足特定条件的数据才会被打印。
def print_if_not_printed(data, condition=lambda x: True):
if condition(data):
print(data)
在这个例子中,condition
是一个函数,只有在condition(data)
返回True
时,数据才会被打印。这种方法提供了更多的灵活性,可以根据具体需求自定义判断条件。
三、使用日志模块
Python的logging
模块提供了灵活的日志记录功能,可以通过配置不同的日志级别和输出目标来控制打印行为。通过配置日志记录器,可以避免重复输出相同的日志信息。
import logging
logger = logging.getLogger(__name__)
logger.setLevel(logging.INFO)
def log_once(message):
if not logger.hasHandlers():
handler = logging.StreamHandler()
logger.addHandler(handler)
if message not in {h.getMessage() for h in logger.handlers[0].stream.handlers}:
logger.info(message)
在这个例子中,log_once
函数通过检查日志处理程序的输出流来避免重复记录相同的消息。这种方法适用于需要记录大量日志并避免重复输出的场景。
四、使用自定义类
通过自定义类,我们可以实现更复杂的逻辑来控制打印行为。可以在类中维护一个状态或计数器,以记录已打印的数据并决定是否进行打印。
class UniquePrinter:
def __init__(self):
self.printed = set()
def print(self, data):
if data not in self.printed:
print(data)
self.printed.add(data)
printer = UniquePrinter()
printer.print("Hello")
printer.print("Hello")
printer.print("World")
在这个例子中,UniquePrinter
类通过维护一个集合printed
来记录已打印的数据。print
方法在打印前检查数据是否在集合中,从而避免重复打印。
五、使用装饰器
装饰器是一种强大的工具,可以在不修改原函数的情况下为其添加额外的功能。可以使用装饰器来包装打印函数,从而避免重复打印。
def avoid_duplicate_print(func):
printed = set()
def wrapper(data):
if data not in printed:
func(data)
printed.add(data)
return wrapper
@avoid_duplicate_print
def print_message(message):
print(message)
print_message("Hello")
print_message("Hello")
print_message("World")
在这个例子中,avoid_duplicate_print
是一个装饰器,它包装了print_message
函数。装饰器通过内部的printed
集合记录已打印的数据,确保每条消息只打印一次。
六、总结
在Python中避免重复打印可以通过多种方法实现,选择合适的方法取决于具体的应用场景。使用集合是一种简单且有效的方式,而条件判断和日志模块提供了更多的灵活性和可配置性。自定义类和装饰器则提供了更高的可扩展性,适合需要复杂逻辑控制的场景。无论采用哪种方法,关键在于理解其原理和适用场景,以便在实际开发中有效应用。
相关问答FAQs:
如何在Python中检查并避免重复打印的内容?
在Python中,可以使用集合(set)或字典(dict)来存储已经打印的内容,这样可以在打印之前先检查该内容是否已经存在于集合或字典中。如果存在,则跳过打印;如果不存在,则打印并将其添加到集合中。例如:
printed_items = set()
for item in items:
if item not in printed_items:
print(item)
printed_items.add(item)
使用什么方法可以有效减少重复输出的代码行?
使用函数或类封装打印逻辑是一个有效的方式。通过定义一个函数来处理打印操作,可以在函数内部进行重复检查,从而避免代码的重复。例如:
def print_unique(item, printed_items):
if item not in printed_items:
print(item)
printed_items.add(item)
printed_items = set()
for item in items:
print_unique(item, printed_items)
在多线程环境中,如何确保打印操作不发生重复?
在多线程环境中,可以使用锁(Lock)来确保在同一时间只有一个线程可以进行打印操作。这样可以避免多个线程同时打印相同的内容,从而造成重复输出。示例如下:
from threading import Lock
lock = Lock()
printed_items = set()
def thread_safe_print(item):
with lock:
if item not in printed_items:
print(item)
printed_items.add(item)
通过这些方法,可以有效避免在Python中重复打印的情况。
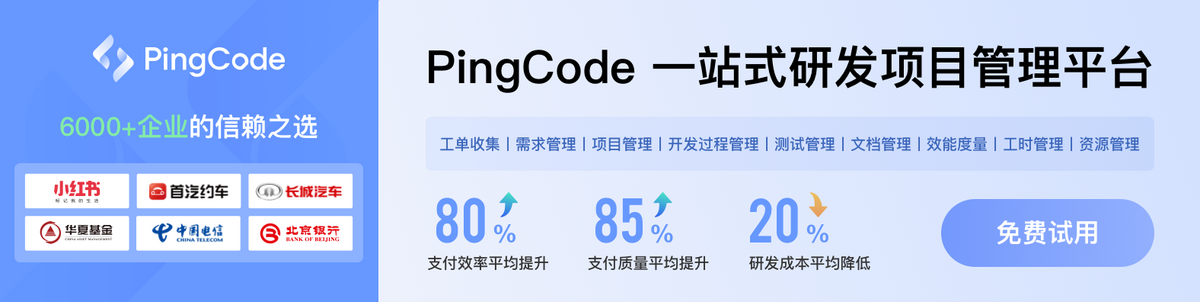