Python对文本进行清洗的方法包括:删除噪声字符、去除停用词、文本规范化、拼写纠正、词形还原。删除噪声字符是最基础的步骤,它能去掉文本中的无用信息,如标点符号和特殊字符。下面将详细描述删除噪声字符的过程。
删除噪声字符通常包括去除标点符号、数字、特殊字符等步骤。首先,使用Python的正则表达式库re
可以方便地匹配和替换这些字符。例如,使用re.sub(r'[^\w\s]', '', text)
可以去除文本中的所有标点符号。其次,对于特殊字符和数字,可以分别使用re.sub(r'\d+', '', text)
和re.sub(r'[^\w\s]', '', text)
来去除。这样做的目的是为了减少文本中的无关信息,使后续的分析和处理更为准确。
一、删除噪声字符
在文本清洗的过程中,删除噪声字符是首要步骤。噪声字符包括标点符号、数字以及特殊符号等。这些字符通常对文本分析没有帮助,甚至可能干扰文本的处理,因此需要在文本预处理阶段将其去除。
- 使用正则表达式去除标点符号
Python中的re
模块提供了强大的正则表达式功能,可以用来匹配和替换文本中的特定模式。去除标点符号可以通过以下代码实现:
import re
def remove_punctuation(text):
return re.sub(r'[^\w\s]', '', text)
text = "Hello, world! This is a test."
cleaned_text = remove_punctuation(text)
print(cleaned_text) # 输出: Hello world This is a test
- 去除数字和特殊字符
同样地,可以使用正则表达式去除数字和特殊字符:
def remove_digits(text):
return re.sub(r'\d+', '', text)
def remove_special_chars(text):
return re.sub(r'[^A-Za-z0-9\s]', '', text)
text = "Text with numbers 12345 and special characters #@$%!"
cleaned_text = remove_digits(text)
cleaned_text = remove_special_chars(cleaned_text)
print(cleaned_text) # 输出: Text with numbers and special characters
二、去除停用词
停用词是指那些在文本处理中被认为无意义的高频词,如“的”、“是”、“在”等。这些词通常不影响文本的主题或情感分析,但会增加计算的复杂度和时间。因此,在文本清洗过程中,需要去除停用词。
- 使用NLTK去除停用词
NLTK(自然语言工具包)是Python中一个强大的自然语言处理库,它提供了丰富的工具和数据集用于文本分析。我们可以使用NLTK的停用词列表来去除文本中的停用词:
import nltk
from nltk.corpus import stopwords
nltk.download('stopwords')
stop_words = set(stopwords.words('english'))
def remove_stopwords(text):
words = text.split()
filtered_words = [word for word in words if word.lower() not in stop_words]
return ' '.join(filtered_words)
text = "This is a simple text with some stopwords."
cleaned_text = remove_stopwords(text)
print(cleaned_text) # 输出: simple text stopwords
- 自定义停用词列表
根据具体的文本分析任务,有时需要自定义停用词列表。可以在标准停用词列表的基础上,添加或删除特定的词:
custom_stop_words = stop_words.union({'simple'})
cleaned_text = remove_stopwords(text)
print(cleaned_text) # 输出: text stopwords
三、文本规范化
文本规范化是指将文本转换为统一的格式,以便于后续的处理和分析。文本规范化的步骤通常包括大小写转换、字符编码转换等。
- 大小写转换
将文本中的所有字符转换为小写,有助于消除大小写对文本分析的影响:
def to_lowercase(text):
return text.lower()
text = "This Is A Test."
cleaned_text = to_lowercase(text)
print(cleaned_text) # 输出: this is a test
- 字符编码转换
确保文本使用统一的字符编码格式(如UTF-8),可以避免因为编码不一致导致的文本处理错误:
def to_utf8(text):
return text.encode('utf-8', 'ignore').decode('utf-8')
text = "Text with special characters: ñ, é, ü."
cleaned_text = to_utf8(text)
print(cleaned_text) # 输出: Text with special characters: ñ, é, ü.
四、拼写纠正
拼写错误可能会影响文本分析的准确性,尤其是在情感分析和主题建模等任务中。因此,文本清洗时通常需要进行拼写纠正。
- 使用
TextBlob
进行拼写纠正
TextBlob
是一个简单易用的Python库,可以用于拼写纠正:
from textblob import TextBlob
def correct_spelling(text):
blob = TextBlob(text)
return str(blob.correct())
text = "This is a smple text with speling erors."
cleaned_text = correct_spelling(text)
print(cleaned_text) # 输出: This is a sample text with spelling errors.
- 使用
autocorrect
库
另一个常用的拼写纠正库是autocorrect
:
from autocorrect import Speller
spell = Speller(lang='en')
def correct_spelling_autocorrect(text):
return spell(text)
text = "This is a smple text with speling erors."
cleaned_text = correct_spelling_autocorrect(text)
print(cleaned_text) # 输出: This is a simple text with spelling errors.
五、词形还原
词形还原是指将词语还原为其词根形式。这可以帮助减少文本的多样性,提高分析的准确性。词形还原通常包括词干提取和词形还原两种方法。
- 使用NLTK进行词干提取
词干提取是指将词语还原为其词干形式,通常使用算法如Porter Stemmer:
from nltk.stem import PorterStemmer
def stem_words(text):
stemmer = PorterStemmer()
words = text.split()
stemmed_words = [stemmer.stem(word) for word in words]
return ' '.join(stemmed_words)
text = "running runs runner"
cleaned_text = stem_words(text)
print(cleaned_text) # 输出: run run runner
- 使用NLTK进行词形还原
词形还原是将词语还原为其标准形式,通常使用WordNet Lemmatizer:
from nltk.stem import WordNetLemmatizer
def lemmatize_words(text):
lemmatizer = WordNetLemmatizer()
words = text.split()
lemmatized_words = [lemmatizer.lemmatize(word) for word in words]
return ' '.join(lemmatized_words)
text = "running runs runner"
cleaned_text = lemmatize_words(text)
print(cleaned_text) # 输出: running run runner
通过这些步骤,我们可以对文本进行有效的清洗,从而提高文本分析和处理的准确性和效率。在实际应用中,根据具体的文本分析任务,可能需要选择和组合不同的清洗步骤,以达到最佳的效果。
相关问答FAQs:
如何使用Python进行文本清洗?
文本清洗是自然语言处理中的重要步骤,通常涉及去除无用的字符、标点符号、以及多余的空格。在Python中,可以使用内置的字符串函数和正则表达式库(如re
)来实现这些操作。例如,可以通过str.replace()
方法去除特定字符,或者使用re.sub()
函数进行复杂的模式匹配和替换。
Python中的文本清洗工具和库有哪些?
有许多库可以帮助简化文本清洗的过程。pandas
库提供了强大的数据处理功能,可以使用DataFrame
的str
方法进行批量清洗;nltk
和spaCy
是两个流行的自然语言处理库,提供了文本预处理的工具,如分词、去停用词等。利用这些工具,可以高效地处理和清洗文本数据。
如何处理文本中的停用词?
停用词是指在特定上下文中频繁出现但对分析结果贡献不大的词汇。使用Python时,可以通过nltk
库中的stopwords
模块获取常见的停用词列表。清洗文本时,可以将这些停用词从文本中移除,以便更专注于有意义的词汇和短语,提高后续分析的效果。
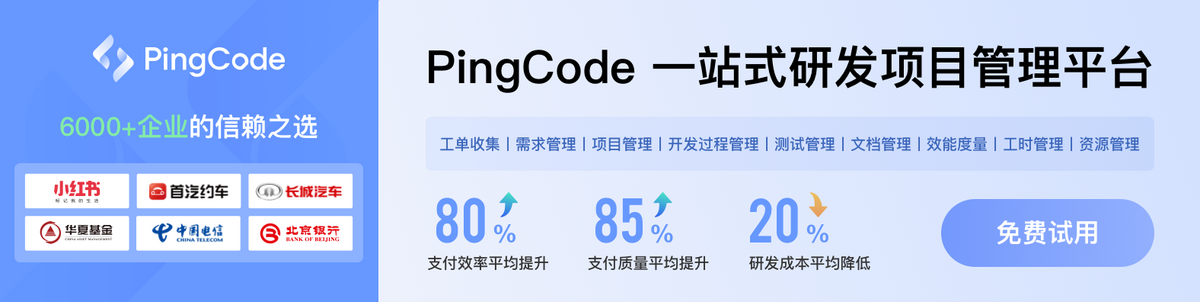