使用Python打出爱心的主要方法包括:利用字符画、使用图形库进行绘制、通过数学函数绘制心形曲线。字符画是最基础的方法,适合初学者;图形库(如Matplotlib或Turtle)可以创建更复杂和精美的图案;数学函数绘制心形曲线则是通过数学公式生成精确的心形。下面将详细介绍利用Python绘制爱心的几种方法。
一、字符画绘制爱心
字符画是一种通过在控制台打印字符来形成图案的艺术形式。对于初学者,这是一种简单且易于理解的方法。通过控制字符的排列,可以在终端输出一个简单的爱心形状。
def print_heart():
heart = [
" <strong> </strong> ",
"<strong></strong> <strong></strong>",
"<strong></strong><strong></strong><strong></strong>",
" <strong></strong><strong></strong> ",
" <strong></strong> ",
" <strong></strong> ",
" "
]
for line in heart:
print(line)
print_heart()
这种方法的优点是简单直接,但图案的复杂程度受限于控制台字符的排列。
二、使用Turtle库绘制爱心
Turtle库是Python内置的一个简单图形绘制库,适合用于绘制简单的图形。通过Turtle库,我们可以绘制一个更为复杂和精美的爱心。
import turtle
def draw_heart():
turtle.bgcolor("white")
turtle.pensize(2)
turtle.speed(1)
turtle.color("red")
turtle.begin_fill()
turtle.left(140)
turtle.forward(224)
turtle.circle(-112, 200)
turtle.left(120)
turtle.circle(-112, 200)
turtle.forward(224)
turtle.end_fill()
turtle.hideturtle()
turtle.done()
draw_heart()
通过调整Turtle的速度、颜色、笔宽等参数,可以获得不同的效果。这种方法不仅适合绘制爱心,也可以用于其他图形的绘制。
三、使用Matplotlib绘制心形曲线
Matplotlib是一个强大的绘图库,可以用于绘制各种复杂图形。通过数学公式,可以精确地绘制出心形曲线。
import numpy as np
import matplotlib.pyplot as plt
def draw_heart_curve():
t = np.linspace(0, 2 * np.pi, 1000)
x = 16 * np.sin(t)3
y = 13 * np.cos(t) - 5 * np.cos(2*t) - 2 * np.cos(3*t) - np.cos(4*t)
plt.plot(x, y, 'r')
plt.fill(x, y, 'r')
plt.title("Heart Shape")
plt.show()
draw_heart_curve()
通过这种方法绘制的爱心具有高度的精确性和美观性,适合对图形要求较高的场合。
四、使用数学函数绘制心形曲线
数学函数绘制心形曲线是一种基于数学公式的精确方法。心形曲线的方程如下:
x = 16 * sin(t)^3
y = 13 * cos(t) – 5 * cos(2t) – 2 * cos(3t) – cos(4*t)
利用该公式,可以生成一个完美的心形曲线。
import numpy as np
import matplotlib.pyplot as plt
def plot_heart():
t = np.linspace(0, 2 * np.pi, 1000)
x = 16 * np.sin(t)3
y = 13 * np.cos(t) - 5 * np.cos(2*t) - 2 * np.cos(3*t) - np.cos(4*t)
plt.figure(figsize=(6, 6))
plt.plot(x, y, color='red')
plt.fill_between(x, y, color='pink')
plt.xlim(-20, 20)
plt.ylim(-20, 20)
plt.title("Mathematical Heart Curve")
plt.show()
plot_heart()
这种方法非常适合需要精确表达心形曲线的场合,例如在数学教学或研究中。
五、总结
通过以上方法,我们可以用Python轻松绘制出不同风格的爱心。字符画简单易懂,适合初学者;Turtle库提供了更多的绘图功能,适合较复杂的图案;Matplotlib和数学函数则可以生成精确和美观的心形图案。选择哪种方法主要取决于个人的需求和编程水平。
相关问答FAQs:
如何用Python绘制爱心图形?
使用Python绘制爱心图形可以通过多种方式实现,其中最流行的方法之一是使用绘图库,例如Matplotlib。下面是一个简单的代码示例,帮助您快速创建爱心图形:
import numpy as np
import matplotlib.pyplot as plt
t = np.linspace(0, 2 * np.pi, 1000)
x = 16 * np.sin(t)**3
y = 13 * np.cos(t) - 5 * np.cos(2*t) - 2 * np.cos(3*t) - np.cos(4*t)
plt.figure(figsize=(8, 6))
plt.plot(x, y, color='red')
plt.title('Heart Shape')
plt.axis('equal')
plt.show()
运行这段代码,将会绘制出一个红色的爱心图形。
绘制爱心图形所需的Python库有哪些?
要绘制爱心图形,推荐使用NumPy和Matplotlib这两个库。NumPy用于生成数据点,Matplotlib则负责将这些数据点可视化。您可以通过以下命令安装这些库:
pip install numpy matplotlib
是否可以使用其他图形库绘制爱心图形?
当然可以,除了Matplotlib,您还可以使用其他图形库,例如Turtle或Pygame。Turtle库适合初学者,它提供了简单易用的绘图功能。以下是使用Turtle绘制爱心的示例代码:
import turtle
t = turtle.Turtle()
t.fillcolor("red")
t.begin_fill()
t.left(140)
t.forward(224)
t.circle(-112, 200)
t.left(120)
t.circle(-112, 200)
t.forward(224)
t.end_fill()
turtle.done()
运行这段代码,您也能看到一个漂亮的爱心图案。
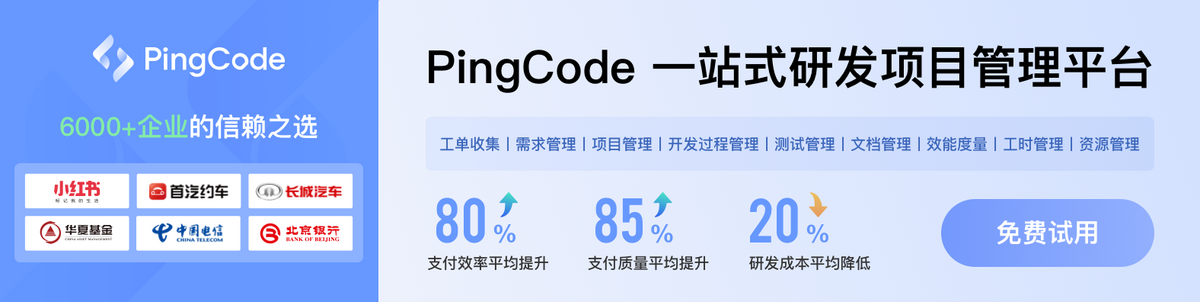