在Python中使用线程有助于提高程序的并发性和性能,尤其是在I/O操作密集型任务中。可以通过threading
模块创建和管理线程、使用锁机制防止数据竞争、通过Thread
类实现线程的创建和启动。下面将详细介绍这些方法。
一、使用threading
模块创建线程
Python中的threading
模块提供了创建和管理线程的基本功能。要使用线程,首先需要导入这个模块。通过创建Thread
对象并调用它的start()
方法来启动线程。
1. 创建线程
threading.Thread
类是Python中实现线程的基础类。要创建一个线程,可以继承这个类并重写run
方法,或者直接传入目标函数。
import threading
def print_numbers():
for i in range(5):
print(i)
创建一个线程,目标是print_numbers函数
thread = threading.Thread(target=print_numbers)
启动线程
thread.start()
2. 线程的生命周期
线程的生命周期包括创建、运行和终止。线程在调用start()
方法后进入可运行状态,run()
方法执行完后自动终止。可以使用join()
方法等待线程完成。
thread.join()
二、使用锁机制防止数据竞争
在多线程环境中,如果多个线程同时访问和修改共享数据,可能会导致数据不一致的问题。Python提供了锁机制来避免这种竞争。
1. 使用Lock
对象
可以通过threading.Lock
对象来确保同一时间只有一个线程可以访问共享资源。
lock = threading.Lock()
def synchronized_function():
lock.acquire() # 请求锁
try:
# 对共享资源进行操作
pass
finally:
lock.release() # 释放锁
2. 使用上下文管理器
为了更简洁地使用锁,可以利用上下文管理器(with
语句),它会自动获取和释放锁。
def synchronized_function():
with lock:
# 对共享资源进行操作
pass
三、通过Thread
类实现线程的创建和启动
除了直接创建Thread
对象并传入目标函数,还可以通过继承Thread
类来创建线程。这种方式在需要更多定制化操作时非常有用。
1. 自定义线程类
通过继承threading.Thread
类,可以创建一个自定义线程类,并重写run()
方法。
class MyThread(threading.Thread):
def __init__(self, name):
threading.Thread.__init__(self)
self.name = name
def run(self):
print(f"Thread {self.name} is running")
创建并启动自定义线程
thread = MyThread("A")
thread.start()
2. 传递参数给线程
在自定义线程类中,可以通过构造函数传递参数给线程。
class MyThread(threading.Thread):
def __init__(self, name, data):
threading.Thread.__init__(self)
self.name = name
self.data = data
def run(self):
print(f"Thread {self.name} with data {self.data}")
thread = MyThread("A", [1, 2, 3])
thread.start()
四、线程同步与通信
多线程环境中,线程之间的同步与通信也非常重要。Python提供了多种机制来实现这些功能。
1. 使用Queue
进行线程间通信
queue.Queue
提供了线程安全的队列,可以在生产者-消费者模式中使用。
from queue import Queue
def producer(queue):
for i in range(5):
queue.put(i)
def consumer(queue):
while not queue.empty():
item = queue.get()
print(item)
queue = Queue()
producer_thread = threading.Thread(target=producer, args=(queue,))
consumer_thread = threading.Thread(target=consumer, args=(queue,))
producer_thread.start()
consumer_thread.start()
producer_thread.join()
consumer_thread.join()
2. 使用Event
对象进行线程同步
threading.Event
对象可以用来实现线程之间的简单通信和同步。
event = threading.Event()
def task():
event.wait() # 等待事件信号
print("Event received, task is running")
thread = threading.Thread(target=task)
thread.start()
触发事件
event.set()
五、线程池的使用
在需要管理大量线程时,直接创建和管理Thread
对象可能会导致效率低下和资源浪费。Python提供了concurrent.futures
模块,可以更方便地管理线程池。
1. 使用ThreadPoolExecutor
ThreadPoolExecutor
是一个高效的线程池管理器,可以通过简单的API提交任务并获取结果。
from concurrent.futures import ThreadPoolExecutor
def compute_square(n):
return n * n
with ThreadPoolExecutor(max_workers=5) as executor:
futures = [executor.submit(compute_square, i) for i in range(10)]
for future in futures:
print(future.result())
2. 使用map
方法
ThreadPoolExecutor
的map
方法可以用于将函数应用到迭代器的每个元素上,并返回结果。
with ThreadPoolExecutor(max_workers=5) as executor:
results = executor.map(compute_square, range(10))
for result in results:
print(result)
六、线程的优缺点
多线程编程可以提高程序的并发性,但也有一些缺点和挑战。
1. 优点
- 并发执行:线程允许多个任务同时执行,适用于I/O密集型任务。
- 资源共享:线程共享进程的内存空间,可以高效地共享数据。
2. 缺点
- 复杂性:多线程编程增加了程序的复杂性,尤其是线程间的同步和通信。
- 竞争条件:不当的线程管理可能导致数据竞争和死锁。
七、GIL对Python线程的影响
Python的全局解释器锁(GIL)是一个影响Python线程性能的重要因素,尤其是在CPU密集型任务中。
1. 什么是GIL
GIL是Python解释器用来保护访问Python对象的互斥锁,确保同一时间只有一个线程执行Python字节码。
2. GIL的影响
GIL限制了多线程的并发能力,使得在CPU密集型任务中,多线程不能有效地利用多核CPU。
3. 解决方案
对于CPU密集型任务,可以使用多进程(multiprocessing
模块)替代多线程,以绕过GIL限制。
八、使用multiprocessing
模块
在需要充分利用多核CPU的场景中,multiprocessing
模块提供了更合适的解决方案。
1. 创建进程
multiprocessing
模块提供了Process
类,可以创建和管理进程。
from multiprocessing import Process
def task():
print("Task is running")
process = Process(target=task)
process.start()
process.join()
2. 进程池
multiprocessing
还提供了Pool
类,用于管理进程池。
from multiprocessing import Pool
def compute_square(n):
return n * n
with Pool(processes=5) as pool:
results = pool.map(compute_square, range(10))
for result in results:
print(result)
综上所述,Python中的线程提供了一个强大的工具来提高程序的并发性能,尤其是在I/O密集型任务中。然而,在使用多线程时,需要注意线程间的同步与通信,并且了解GIL对多线程的影响。在CPU密集型任务中,可以考虑使用多进程来充分利用多核CPU资源。
相关问答FAQs:
在Python中,线程的基本使用方法是什么?
在Python中,使用线程可以通过threading
模块实现。你可以创建一个新的线程,首先需要定义一个函数,该函数包含线程需要执行的代码。接着,创建一个Thread
对象,将函数作为参数传入,最后调用start()
方法启动线程。以下是一个简单的示例:
import threading
def my_function():
print("Hello from the thread!")
thread = threading.Thread(target=my_function)
thread.start()
thread.join() # 等待线程完成
使用线程时需要注意哪些常见问题?
在使用线程时,有几个常见问题需要注意。首先是线程安全问题,多线程同时访问共享数据可能导致数据不一致。可以使用锁(Lock)来确保同一时间只有一个线程可以访问共享资源。其次,线程的创建和销毁也会消耗资源,因此在设计时应考虑线程的生命周期和数量。此外,Python中的全局解释器锁(GIL)可能会影响多线程程序的性能,尤其是在CPU密集型任务中。
如何在Python中管理线程的生命周期和状态?
管理线程的生命周期可以通过Thread
类提供的方法来实现。可以使用is_alive()
方法检查线程是否仍在运行,使用join()
方法等待线程完成。为了更加灵活地管理线程,可以考虑使用线程池(如concurrent.futures.ThreadPoolExecutor
),它允许你预先定义线程数量并管理任务队列,从而简化线程的创建和销毁过程。以下是一个使用线程池的示例:
from concurrent.futures import ThreadPoolExecutor
def task(n):
print(f"Task {n} is running")
with ThreadPoolExecutor(max_workers=5) as executor:
for i in range(10):
executor.submit(task, i)
通过这种方式,可以有效地管理线程的生命周期和提高程序的性能。
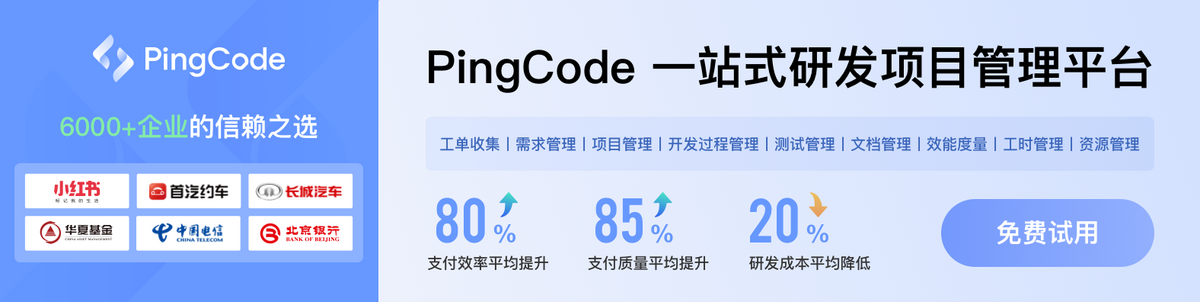