在Python中,使用sort()
方法或sorted()
函数可以轻松实现降序排序。通过在调用sort()
方法时设置reverse=True
参数,或在使用sorted()
函数时传入相同的参数,可以将列表按降序排列。下面详细介绍如何使用这两种方法对列表进行降序排序。
一、使用sort()
方法降序排序
sort()
方法是列表对象的方法,用于对列表进行就地排序,意味着它会直接修改原列表而不返回新列表。要实现降序排序,只需将reverse
参数设置为True
。
# 示例
numbers = [1, 3, 5, 2, 4]
numbers.sort(reverse=True)
print(numbers) # 输出: [5, 4, 3, 2, 1]
在这个例子中,numbers.sort(reverse=True)
将列表numbers
按降序排序。此方法非常高效,但注意它会修改原始列表。
二、使用sorted()
函数降序排序
sorted()
函数与sort()
方法类似,但它不会修改原列表,而是返回一个新的已排序列表。使用同样的reverse=True
参数可以实现降序排序。
# 示例
numbers = [1, 3, 5, 2, 4]
sorted_numbers = sorted(numbers, reverse=True)
print(sorted_numbers) # 输出: [5, 4, 3, 2, 1]
print(numbers) # 输出: [1, 3, 5, 2, 4]
在这个例子中,sorted(numbers, reverse=True)
返回了一个新的列表sorted_numbers
,其元素按降序排列,而原始列表numbers
保持不变。
三、sort()
方法与sorted()
函数的区别
-
就地排序与返回新列表:
sort()
方法直接对列表进行就地排序,不返回新列表;而sorted()
函数会返回一个新的排序后的列表,不影响原始列表。 -
使用场景:如果需要保留原始列表的顺序并获取一个新的排序列表,使用
sorted()
函数是更好的选择;如果只需要对列表进行排序并不需要保留原始顺序,sort()
方法更高效。 -
性能差异:由于
sort()
方法是在列表对象上直接操作,因此在某些情况下其性能可能比sorted()
函数稍好,尤其是当不需要保留原始列表时。
四、在其他数据类型上的应用
虽然sort()
方法仅限于列表对象,但sorted()
函数可以用于任何可迭代对象,如元组、字符串、字典等。
- 对元组进行降序排序:
# 示例
numbers_tuple = (1, 3, 5, 2, 4)
sorted_numbers = sorted(numbers_tuple, reverse=True)
print(sorted_numbers) # 输出: [5, 4, 3, 2, 1]
- 对字符串进行降序排序:
# 示例
text = "python"
sorted_text = sorted(text, reverse=True)
print(''.join(sorted_text)) # 输出: 'ytponh'
- 对字典进行降序排序:
对于字典,可以通过sorted()
函数对字典的键或值进行排序。
# 示例:按字典的值降序排序
scores = {'Alice': 90, 'Bob': 70, 'Charlie': 80}
sorted_scores = sorted(scores.items(), key=lambda item: item[1], reverse=True)
print(sorted_scores) # 输出: [('Alice', 90), ('Charlie', 80), ('Bob', 70)]
五、复杂数据结构的降序排序
对于包含复杂数据结构的列表,如列表中的字典,可以通过指定key
参数来实现自定义排序规则。
# 示例:按字典中的某个键进行降序排序
students = [{'name': 'Alice', 'score': 90}, {'name': 'Bob', 'score': 70}, {'name': 'Charlie', 'score': 80}]
students.sort(key=lambda student: student['score'], reverse=True)
print(students) # 输出: [{'name': 'Alice', 'score': 90}, {'name': 'Charlie', 'score': 80}, {'name': 'Bob', 'score': 70}]
在这个例子中,我们使用了key=lambda student: student['score']
来指定排序规则,即根据每个字典的score
键的值进行排序。
通过以上方法,Python提供了灵活且强大的工具来对各种数据结构进行降序排序。无论是简单的数据类型还是复杂的数据结构,合理使用sort()
方法和sorted()
函数都可以满足不同的排序需求。
相关问答FAQs:
如何在Python中使用sort方法实现降序排序?
在Python中,使用列表的sort方法可以轻松实现降序排序。只需设置reverse=True
参数即可。例如,my_list.sort(reverse=True)
将会对my_list
进行降序排序。此外,sort方法会对原始列表进行修改,而不是返回一个新列表。
在Python中,sort方法与sorted函数有什么区别?
sort方法是列表对象的一个方法,它直接修改原列表的顺序,而sorted函数是一个内置函数,可以接受任何可迭代对象并返回一个新的列表。使用sorted时,可以使用reverse=True
来实现降序排序,而list.sort只能用于列表本身。
在排序时,如何自定义排序规则以满足特定需求?
可以通过传递一个自定义的关键字函数给sort方法或sorted函数来实现特定的排序需求。使用key
参数时,指定一个函数,该函数将应用于每个列表元素以计算排序依据。例如,my_list.sort(key=len, reverse=True)
将根据字符串的长度进行降序排序。这样可以根据不同的需求灵活排序。
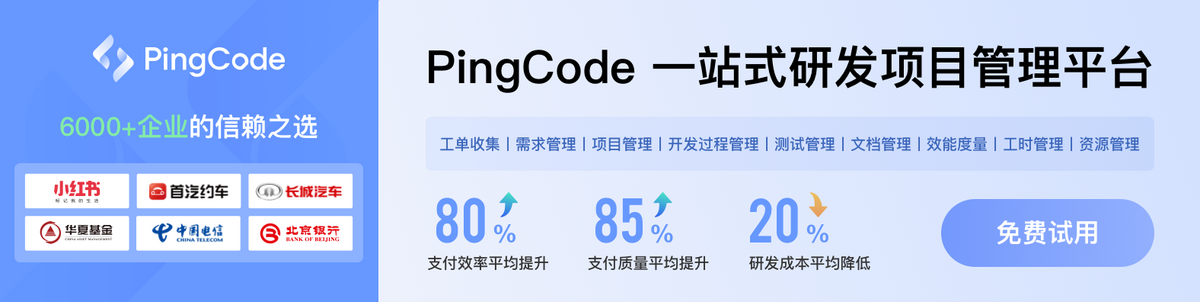