在Python中划分验证集可以通过多种方法实现,常见的方法包括使用sklearn
库的train_test_split
函数、手动划分、以及使用其他专用的库。常见的方法有:使用train_test_split
函数、手动划分、使用KFold交叉验证。下面将重点介绍如何使用train_test_split
函数来划分验证集。
使用train_test_split
函数是最常见的方法之一。这个函数是scikit-learn
库的一部分,它可以轻松地将数据集划分为训练集和验证集。首先,确保已经安装scikit-learn
库,然后可以通过以下代码实现验证集的划分:
from sklearn.model_selection import train_test_split
假设X是特征数据,y是标签数据
X_train, X_val, y_train, y_val = train_test_split(X, y, test_size=0.2, random_state=42)
在上述代码中,test_size=0.2
表示验证集的大小是整个数据集的20%。random_state=42
用于确保每次分割的数据是相同的,以便结果可重复。
一、使用TRAIN_TEST_SPLIT函数
train_test_split
函数是Python中最常用的划分数据集的方法之一。它可以轻松地将数据分为训练集和验证集。使用此方法时,你可以指定验证集的大小和随机状态,以确保可重复性。
-
基本用法
train_test_split
函数可以轻松地将数据集划分为训练集和验证集。其基本用法如下:from sklearn.model_selection import train_test_split
X是特征数据,y是标签数据
X_train, X_val, y_train, y_val = train_test_split(X, y, test_size=0.2, random_state=42)
这里,
test_size=0.2
表示验证集的大小为总数据集的20%,random_state=42
用来确保分割的随机性可重复。 -
调整验证集比例
train_test_split
的test_size
参数允许你自由调整验证集的大小。例如,如果希望验证集占总数据集的30%,可以将test_size
设置为0.3:X_train, X_val, y_train, y_val = train_test_split(X, y, test_size=0.3, random_state=42)
通过调整
test_size
参数,可以根据需要灵活调整验证集的比例。
二、手动划分数据集
在某些情况下,你可能希望对数据集进行更精细的控制,手动划分数据集是一种有效的方法。手动划分可以根据特定的逻辑或规则来对数据进行分配。
-
随机打乱数据
在手动划分数据集之前,首先需要确保数据是随机的。可以使用
numpy
库的shuffle
函数来打乱数据:import numpy as np
假设data是数据集
np.random.shuffle(data)
随机打乱数据后,可以按照一定的比例手动划分训练集和验证集。
-
按比例划分
假设数据集中有
n
个样本,可以手动将前80%的数据分为训练集,后20%的数据分为验证集:train_size = int(0.8 * len(data))
train_data = data[:train_size]
val_data = data[train_size:]
通过手动划分,可以对数据集的分割过程有更好的掌控。
三、使用KFOLD交叉验证
KFold交叉验证是一种更高级的验证集划分方法,它通过多次划分数据集来提高模型的稳定性和可靠性。
-
基本概念
KFold交叉验证将数据集分成
k
个子集(折叠)。在每次迭代中,使用其中k-1
个子集作为训练集,剩下的一个子集作为验证集。通过这种方式,可以确保每个样本都被用作验证集一次。 -
实现方法
使用
scikit-learn
库的KFold
类可以轻松实现KFold交叉验证:from sklearn.model_selection import KFold
kf = KFold(n_splits=5, shuffle=True, random_state=42)
for train_index, val_index in kf.split(X):
X_train, X_val = X[train_index], X[val_index]
y_train, y_val = y[train_index], y[val_index]
# 在这里进行模型训练和验证
通过
n_splits=5
参数,可以将数据集分为5个子集。shuffle=True
确保每次分割数据之前打乱数据,random_state=42
用于结果可重复。
四、使用STRATIFIEDKOLD
StratifiedKFold是一种更为高级的KFold交叉验证方法,它确保每个子集中的标签分布与整个数据集的标签分布相同。
-
基本概念
在处理分类问题时,确保每个子集中的标签分布与整个数据集一致是非常重要的。StratifiedKFold通过分层抽样来实现这一点。
-
实现方法
使用
StratifiedKFold
类可以实现分层KFold交叉验证:from sklearn.model_selection import StratifiedKFold
skf = StratifiedKFold(n_splits=5, shuffle=True, random_state=42)
for train_index, val_index in skf.split(X, y):
X_train, X_val = X[train_index], X[val_index]
y_train, y_val = y[train_index], y[val_index]
# 在这里进行模型训练和验证
通过这种方法,可以确保在每次迭代中,训练集和验证集的标签分布相同。
五、使用数据集工具库
除了scikit-learn
,还可以使用其他数据集工具库来划分验证集,例如pandas
、numpy
等。
-
使用PANDAS
pandas
库提供了强大的数据操作功能,可以轻松地进行数据集的划分:import pandas as pd
from sklearn.model_selection import train_test_split
假设df是一个DataFrame
train_df, val_df = train_test_split(df, test_size=0.2, random_state=42)
通过将数据集转换为
DataFrame
格式,可以利用pandas
的强大功能进行数据操作。 -
使用NUMPY
numpy
库同样提供了强大的数组操作功能,可以用于数据集的划分:import numpy as np
假设X, y是numpy数组
indices = np.arange(X.shape[0])
np.random.shuffle(indices)
train_indices = indices[:int(0.8 * len(indices))]
val_indices = indices[int(0.8 * len(indices)):]
X_train, X_val = X[train_indices], X[val_indices]
y_train, y_val = y[train_indices], y[val_indices]
通过对索引进行随机打乱,可以实现数据集的划分。
总结来说,Python中提供了多种方法来划分验证集,选择合适的方法可以根据具体需求和数据集的特点来进行。无论是使用train_test_split
函数、手动划分、还是使用KFold交叉验证,合理的验证集划分是模型训练和评估的重要步骤。
相关问答FAQs:
如何在Python中创建验证集?
在Python中创建验证集通常涉及将数据集划分为训练集和验证集。可以使用train_test_split
函数来自sklearn.model_selection
模块,轻松实现这一过程。通过设置test_size
参数,您可以指定验证集所占的比例,例如0.2表示20%的数据将用于验证。以下是一个基本示例:
from sklearn.model_selection import train_test_split
# 假设X是特征,y是标签
X_train, X_val, y_train, y_val = train_test_split(X, y, test_size=0.2, random_state=42)
这种方法确保了随机划分,从而避免了过拟合。
验证集的大小应该如何选择?
验证集的大小通常取决于数据集的整体规模。一般来说,数据集越大,验证集可以相对较小,比如10%到20%。对于较小的数据集,可能需要留出更多的数据以确保验证结果的可靠性,建议比例在20%到30%之间。同时,确保训练集仍然足够大以保持模型的有效性。
在深度学习中如何划分验证集?
在深度学习中,划分验证集的方法与传统机器学习相似,但通常会使用更复杂的数据预处理。使用如TensorFlow
或PyTorch
等框架时,可以在数据加载阶段使用train_test_split
,或者在创建数据生成器时指定验证集的比例。例如,在TensorFlow中,您可以使用ImageDataGenerator
来划分验证集:
from tensorflow.keras.preprocessing.image import ImageDataGenerator
datagen = ImageDataGenerator(validation_split=0.2)
train_generator = datagen.flow_from_directory(
'data/train',
target_size=(150, 150),
batch_size=32,
class_mode='binary',
subset='training'
)
validation_generator = datagen.flow_from_directory(
'data/train',
target_size=(150, 150),
batch_size=32,
class_mode='binary',
subset='validation'
)
这种方法确保数据的随机性和多样性,为模型训练提供了良好的基础。
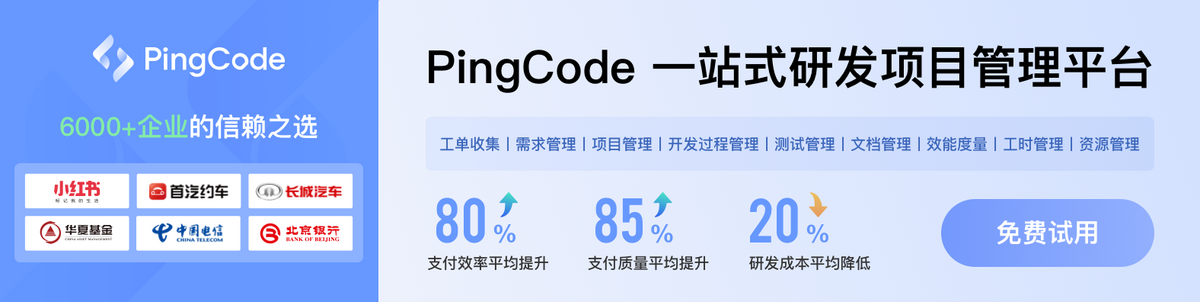